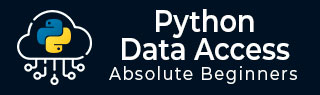
- Python Data Access Tutorial
- Python Data Access - Home
- Python MySQL
- Python MySQL - Introduction
- Python MySQL - Database Connection
- Python MySQL - Create Database
- Python MySQL - Create Table
- Python MySQL - Insert Data
- Python MySQL - Select Data
- Python MySQL - Where Clause
- Python MySQL - Order By
- Python MySQL - Update Table
- Python MySQL - Delete Data
- Python MySQL - Drop Table
- Python MySQL - Limit
- Python MySQL - Join
- Python MySQL - Cursor Object
- Python PostgreSQL
- Python PostgreSQL - Introduction
- Python PostgreSQL - Database Connection
- Python PostgreSQL - Create Database
- Python PostgreSQL - Create Table
- Python PostgreSQL - Insert Data
- Python PostgreSQL - Select Data
- Python PostgreSQL - Where Clause
- Python PostgreSQL - Order By
- Python PostgreSQL - Update Table
- Python PostgreSQL - Delete Data
- Python PostgreSQL - Drop Table
- Python PostgreSQL - Limit
- Python PostgreSQL - Join
- Python PostgreSQL - Cursor Object
- Python SQLite
- Python SQLite - Introduction
- Python SQLite - Establishing Connection
- Python SQLite - Create Table
- Python SQLite - Insert Data
- Python SQLite - Select Data
- Python SQLite - Where Clause
- Python SQLite - Order By
- Python SQLite - Update Table
- Python SQLite - Delete Data
- Python SQLite - Drop Table
- Python SQLite - Limit
- Python SQLite - Join
- Python SQLite - Cursor Object
- Python MongoDB
- Python MongoDB - Introduction
- Python MongoDB - Create Database
- Python MongoDB - Create Collection
- Python MongoDB - Insert Document
- Python MongoDB - Find
- Python MongoDB - Query
- Python MongoDB - Sort
- Python MongoDB - Delete Document
- Python MongoDB - Drop Collection
- Python MongoDB - Update
- Python MongoDB - Limit
- Python Data Access Resources
- Python Data Access - Quick Guide
- Python Data Access - Useful Resources
- Python Data Access - Discussion
Python MongoDB - Insert Document
You can store documents into MongoDB using the insert() method. This method accepts a JSON document as a parameter.
Syntax
Following is the syntax of the insert method.
>db.COLLECTION_NAME.insert(DOCUMENT_NAME)
Example
> use mydb switched to db mydb > db.createCollection("sample") { "ok" : 1 } > doc1 = {"name": "Ram", "age": "26", "city": "Hyderabad"} { "name" : "Ram", "age" : "26", "city" : "Hyderabad" } > db.sample.insert(doc1) WriteResult({ "nInserted" : 1 }) >
Similarly, you can also insert multiple documents using the insert() method.
> use testDB switched to db testDB > db.createCollection("sample") { "ok" : 1 } > data = [ { "_id": "1001", "name": "Ram", "age": "26", "city": "Hyderabad" }, { "_id": "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" }, { "_id": "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" } ] [ { "_id" : "1001", "name" : "Ram", "age" : "26", "city" : "Hyderabad" }, { "_id" : "1002", "name" : "Rahim", "age" : 27, "city" : "Bangalore" }, { "_id" : "1003", "name" : "Robert", "age" : 28, "city" : "Mumbai" } ] > db.sample.insert(data) BulkWriteResult ({ "writeErrors" : [ ], "writeConcernErrors" : [ ], "nInserted" : 3, "nUpserted" : 0, "nMatched" : 0, "nModified" : 0, "nRemoved" : 0, "upserted" : [ ] }) >
Creating a collection using python
Pymongo provides a method named insert_one() to insert a document in MangoDB. To this method, we need to pass the document in dictionary format.
Example
Following example inserts a document in the collection named example.
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['mydb'] #Creating a collection coll = db['example'] #Inserting document into a collection doc1 = {"name": "Ram", "age": "26", "city": "Hyderabad"} coll.insert_one(doc1) print(coll.find_one())
Output
{ '_id': ObjectId('5d63ad6ce043e2a93885858b'), 'name': 'Ram', 'age': '26', 'city': 'Hyderabad' }
To insert multiple documents into MongoDB using pymongo, you need to invoke the insert_many() method.
from pymongo import MongoClient #Creating a pymongo client client = MongoClient('localhost', 27017) #Getting the database instance db = client['mydb'] #Creating a collection coll = db['example'] #Inserting document into a collection data = [ { "_id": "101", "name": "Ram", "age": "26", "city": "Hyderabad" }, { "_id": "102", "name": "Rahim", "age": "27", "city": "Bangalore" }, { "_id": "103", "name": "Robert", "age": "28", "city": "Mumbai" } ] res = coll.insert_many(data) print("Data inserted ......") print(res.inserted_ids)
Output
Data inserted ...... ['101', '102', '103']
Advertisements
To Continue Learning Please Login