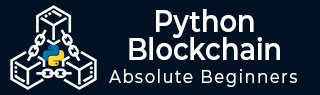
- Python Blockchain Tutorial
- Python Blockchain - Home
- Python Blockchain - Introduction
- Blockchain - Developing Client
- Blockchain - Client Class
- Blockchain - Transaction Class
- Creating Multiple Transactions
- Blockchain - Block Class
- Blockchain - Creating Genesis Block
- Blockchain - Creating Blockchain
- Blockchain - Adding Genesis Block
- Blockchain - Creating Miners
- Blockchain - Adding Blocks
- Blockchain - Scope & Conclusion
- Python Blockchain Resources
- Python Blockchain - Quick Guide
- Python Blockchain - Resources
- Python Blockchain - Discussion
Python Creating Blockchain
A blockchain contains a list of blocks chained to each other. To store the entire list, we will create a list variable called TPCoins −
TPCoins = []
We will also write a utility method called dump_blockchain for dumping the contents of the entire blockchain. We first print the length of the blockchain so that we know how many blocks are currently present in the blockchain.
def dump_blockchain (self): print ("Number of blocks in the chain: " + str(len (self)))
Note that as the time passes, the number of blocks in the blockchain would be extraordinarily high for printing. Thus, when you print the contents of the blockchain you may have to decide on the range that you would like to examine. In the code below, we have printed the entire blockchain as we would not be adding too many blocks in the current demo.
To iterate through the chain, we set up a for loop as follows −
for x in range (len(TPCoins)): block_temp = TPCoins[x]
Each referenced block is copied to a temporary variable called block_temp.
We print the block number as a heading for each block. Note that the numbers would start with zero, the first block is a genesis block that is numbered zero.
print ("block # " + str(x))
Within each block, we have stored a list of three transactions (except for the genesis block) in a variable called verified_transactions. We iterate this list in a for loop and for each retrieved item, we call display_transaction function to display the transaction details.
for transaction in block_temp.verified_transactions: display_transaction (transaction)
The entire function definition is shown below −
def dump_blockchain (self): print ("Number of blocks in the chain: " + str(len (self))) for x in range (len(TPCoins)): block_temp = TPCoins[x] print ("block # " + str(x)) for transaction in block_temp.verified_transactions: display_transaction (transaction) print ('--------------') print ('=====================================')
Note that here we have inserted the separators at appropriate points in the code to demarcate the blocks and transactions within it.
As we have now created a blockchain for storing blocks, our next task is to create blocks and start adding it to the blockchain. For this purpose, we will add a genesis block that you have already created in the earlier step.