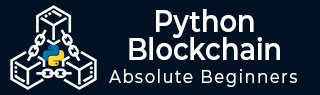
- Python Blockchain Tutorial
- Python Blockchain - Home
- Python Blockchain - Introduction
- Blockchain - Developing Client
- Blockchain - Client Class
- Blockchain - Transaction Class
- Creating Multiple Transactions
- Blockchain - Block Class
- Blockchain - Creating Genesis Block
- Blockchain - Creating Blockchain
- Blockchain - Adding Genesis Block
- Blockchain - Creating Miners
- Blockchain - Adding Blocks
- Blockchain - Scope & Conclusion
- Python Blockchain Resources
- Python Blockchain - Quick Guide
- Python Blockchain - Resources
- Python Blockchain - Discussion
Python Blockchain - Adding Blocks
Each miner will pick up the transactions from a previously created transaction pool. To track the number of messages already mined, we have to create a global variable −
last_transaction_index = 0
We will now have our first miner adding a block to the blockchain.
Adding First Block
To add a new block, we first create an instance of the Block class.
block = Block()
We pick up the top 3 transactions from the queue −
for i in range(3): temp_transaction = transactions[last_transaction_index] # validate transaction
Before adding the transaction to the block the miner will verify the validity of the transaction. The transaction validity is verified by testing for equality the hash provided by the sender against the hash generated by the miner using sender’s public key. Also, the miner will verify that the sender has sufficient balance to pay for the current transaction.
For brevity, we have not included this functionality in the tutorial. After the transaction is validated, we add it to the verified_transactions list in the block instance.
block.verified_transactions.append (temp_transaction)
We increment the last transaction index so that the next miner will pick up subsequent transactions in the queue.
last_transaction_index += 1
We add exactly three transactions to the block. Once this is done, we will initialize the rest of the instance variables of the Block class. We first add the hash of the last block.
block.previous_block_hash = last_block_hash
Next, we mine the block with a difficulty level of 2.
block.Nonce = mine (block, 2)
Note that the first parameter to the mine function is a binary object. We now hash the entire block and create a digest on it.
digest = hash (block)
Finally, we add the created block to the blockchain and re-initialize the global variable last_block_hash for the use in next block.
The entire code for adding the block is shown below −
block = Block() for i in range(3): temp_transaction = transactions[last_transaction_index] # validate transaction # if valid block.verified_transactions.append (temp_transaction) last_transaction_index += 1 block.previous_block_hash = last_block_hash block.Nonce = mine (block, 2) digest = hash (block) TPCoins.append (block) last_block_hash = digest
Adding More Blocks
We will now add two more blocks to our blockchain. The code for adding the next two blocks is given below −
# Miner 2 adds a block block = Block() for i in range(3): temp_transaction = transactions[last_transaction_index] # validate transaction # if valid block.verified_transactions.append (temp_transaction) last_transaction_index += 1 block.previous_block_hash = last_block_hash block.Nonce = mine (block, 2)digest = hash (block) TPCoins.append (block)last_block_hash = digest # Miner 3 adds a block block = Block() for i in range(3): temp_transaction = transactions[last_transaction_index] #display_transaction (temp_transaction) # validate transaction # if valid block.verified_transactions.append (temp_transaction) last_transaction_index += 1 block.previous_block_hash = last_block_hash block.Nonce = mine (block, 2) digest = hash (block) TPCoins.append (block) last_block_hash = digest
When you add these two blocks, you will also see the number of iterations it took to find the Nonce. At this point, our blockchain consists of totally 4 blocks including the genesis block.
Dumping Entire Blockchain
You can verify the contents of the entire blockchain using the following statement −
dump_blockchain(TPCoins)
You would see the output similar to the one shown below −
Number of blocks in the chain: 4 block # 0 sender: Genesis ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100ed272b52ccb539e2cd779 c6cc10ed1dfadf5d97c6ab6de90ed0372b2655626fb79f62d0e01081c163b0864cc68d426bbe943 8e8566303bb77414d4bfcaa3468ab7febac099294de10273a816f7047d4087b4bafa11f141544d4 8e2f10b842cab91faf33153900c7bf6c08c9e47a7df8aa7e60dc9e0798fb2ba3484bbdad2e44302 03010001 ----- value: 500.0 ----- time: 2019-01-14 16:18:02.042739 ----- -------------- ===================================== block # 1 sender: 30819f300d06092a864886f70d010101050003818d0030818902818100bb064c99c492144a9f463 480273aba93ac1db1f0da3cb9f3c1f9d058cf499fd8e54d244da0a8dd6ddd329ec86794b04d773e b4841c9f935ea4d9ccc2821c7a1082d23b6c928d59863407f52fa05d8b47e5157f8fe56c2ce3279 c657f9c6a80500073b0be8093f748aef667c03e64f04f84d311c4d866c12d79d3fc3034563dfb02 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100be93b516b28c6e674abe7 abdb11ce0fdf5bb728b75216b73f37a6432e4b402b3ad8139b8c0ba541a72c8add126b6e1a1308f b98b727beb63c6060356bb177bb7d54b54dbe87aee7353d0a6baa9397704de625d1836d3f42c7ee 5683f6703259592cc24b09699376807f28fe0e00ff882974484d805f874260dfc2d1627473b9102 03010001 ----- value: 15.0 ----- time: 2019-01-14 16:18:01.859915 ----- -------------- sender: 30819f300d06092a864886f70d010101050003818d0030818902818100bb064c99c492144a9f463 480273aba93ac1db1f0da3cb9f3c1f9d058cf499fd8e54d244da0a8dd6ddd329ec86794b04d773e b4841c9f935ea4d9ccc2821c7a1082d23b6c928d59863407f52fa05d8b47e5157f8fe56c2ce3279 c657f9c6a80500073b0be8093f748aef667c03e64f04f84d311c4d866c12d79d3fc3034563dfb02 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100a070c82b34ae143cbe59b 3a2afde7186e9d5bc274955d8112d87a00256a35369acc4d0edfe65e8f9dc93fbd9ee74b9e7ea12 334da38c8c9900e6ced1c4ce93f86e06611e656521a1eab561892b7db0961b4f212d1fd5b5e49ae 09cf8c603a068f9b723aa8a651032ff6f24e5de00387e4d062375799742a359b8f22c5362e56502 03010001 ----- value: 6.0 ----- time: 2019-01-14 16:18:01.860966 ----- -------------- sender: 30819f300d06092a864886f70d010101050003818d0030818902818100be93b516b28c6e674abe7 abdb11ce0fdf5bb728b75216b73f37a6432e4b402b3ad8139b8c0ba541a72c8add126b6e1a1308f b98b727beb63c6060356bb177bb7d54b54dbe87aee7353d0a6baa9397704de625d1836d3f42c7ee 5683f6703259592cc24b09699376807f28fe0e00ff882974484d805f874260dfc2d1627473b9102 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100cba097c0854876f41338c 62598c658f545182cfa4acebce147aedf328181f9c4930f14498fd03c0af6b0cce25be99452a81d f4fa30a53eddbb7bb7b203adf8764a0ccd9db6913a576d68d642d8fd47452590137869c25d9ff83 d68ebe6d616056a8425b85b52e69715b8b85ae807b84638d8f00e321b65e4c33acaf6469e18e302 03010001 ----- value: 2.0 ----- time: 2019-01-14 16:18:01.861958 ----- -------------- ===================================== block # 2 sender: 30819f300d06092a864886f70d010101050003818d0030818902818100a070c82b34ae143cbe59b 3a2afde7186e9d5bc274955d8112d87a00256a35369acc4d0edfe65e8f9dc93fbd9ee74b9e7ea12 334da38c8c9900e6ced1c4ce93f86e06611e656521a1eab561892b7db0961b4f212d1fd5b5e49ae 09cf8c603a068f9b723aa8a651032ff6f24e5de00387e4d062375799742a359b8f22c5362e56502 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100be93b516b28c6e674abe7 abdb11ce0fdf5bb728b75216b73f37a6432e4b402b3ad8139b8c0ba541a72c8add126b6e1a1308f b98b727beb63c6060356bb177bb7d54b54dbe87aee7353d0a6baa9397704de625d1836d3f42c7ee 5683f6703259592cc24b09699376807f28fe0e00ff882974484d805f874260dfc2d1627473b9102 03010001 ----- value: 4.0 ----- time: 2019-01-14 16:18:01.862946 ----- -------------- sender: 30819f300d06092a864886f70d010101050003818d0030818902818100cba097c0854876f41338c 62598c658f545182cfa4acebce147aedf328181f9c4930f14498fd03c0af6b0cce25be99452a81d f4fa30a53eddbb7bb7b203adf8764a0ccd9db6913a576d68d642d8fd47452590137869c25d9ff83 d68ebe6d616056a8425b85b52e69715b8b85ae807b84638d8f00e321b65e4c33acaf6469e18e302 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100a070c82b34ae143cbe59b 3a2afde7186e9d5bc274955d8112d87a00256a35369acc4d0edfe65e8f9dc93fbd9ee74b9e7ea12 334da38c8c9900e6ced1c4ce93f86e06611e656521a1eab561892b7db0961b4f212d1fd5b5e49ae 09cf8c603a068f9b723aa8a651032ff6f24e5de00387e4d062375799742a359b8f22c5362e56502 03010001 ----- value: 7.0 ----- time: 2019-01-14 16:18:01.863932 ----- -------------- sender: 30819f300d06092a864886f70d010101050003818d0030818902818100be93b516b28c6e674abe7 abdb11ce0fdf5bb728b75216b73f37a6432e4b402b3ad8139b8c0ba541a72c8add126b6e1a1308f b98b727beb63c6060356bb177bb7d54b54dbe87aee7353d0a6baa9397704de625d1836d3f42c7ee 5683f6703259592cc24b09699376807f28fe0e00ff882974484d805f874260dfc2d1627473b9102 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100a070c82b34ae143cbe59b 3a2afde7186e9d5bc274955d8112d87a00256a35369acc4d0edfe65e8f9dc93fbd9ee74b9e7ea12 334da38c8c9900e6ced1c4ce93f86e06611e656521a1eab561892b7db0961b4f212d1fd5b5e49ae 09cf8c603a068f9b723aa8a651032ff6f24e5de00387e4d062375799742a359b8f22c5362e56502 03010001 ----- value: 3.0 ----- time: 2019-01-14 16:18:01.865099 ----- -------------- ===================================== block # 3 sender: 30819f300d06092a864886f70d010101050003818d0030818902818100a070c82b34ae143cbe59b 3a2afde7186e9d5bc274955d8112d87a00256a35369acc4d0edfe65e8f9dc93fbd9ee74b9e7ea12 334da38c8c9900e6ced1c4ce93f86e06611e656521a1eab561892b7db0961b4f212d1fd5b5e49ae 09cf8c603a068f9b723aa8a651032ff6f24e5de00387e4d062375799742a359b8f22c5362e56502 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100bb064c99c492144a9f463 480273aba93ac1db1f0da3cb9f3c1f9d058cf499fd8e54d244da0a8dd6ddd329ec86794b04d773e b4841c9f935ea4d9ccc2821c7a1082d23b6c928d59863407f52fa05d8b47e5157f8fe56c2ce3279 c657f9c6a80500073b0be8093f748aef667c03e64f04f84d311c4d866c12d79d3fc3034563dfb02 03010001 ----- value: 8.0 ----- time: 2019-01-14 16:18:01.866219 ----- -------------- sender: 30819f300d06092a864886f70d010101050003818d0030818902818100a070c82b34ae143cbe59b 3a2afde7186e9d5bc274955d8112d87a00256a35369acc4d0edfe65e8f9dc93fbd9ee74b9e7ea12 334da38c8c9900e6ced1c4ce93f86e06611e656521a1eab561892b7db0961b4f212d1fd5b5e49ae 09cf8c603a068f9b723aa8a651032ff6f24e5de00387e4d062375799742a359b8f22c5362e56502 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100be93b516b28c6e674abe7 abdb11ce0fdf5bb728b75216b73f37a6432e4b402b3ad8139b8c0ba541a72c8add126b6e1a1308f b98b727beb63c6060356bb177bb7d54b54dbe87aee7353d0a6baa9397704de625d1836d3f42c7ee 5683f6703259592cc24b09699376807f28fe0e00ff882974484d805f874260dfc2d1627473b9102 03010001 ----- value: 1.0 ----- time: 2019-01-14 16:18:01.867223 ----- -------------- sender: 30819f300d06092a864886f70d010101050003818d0030818902818100cba097c0854876f41338c 62598c658f545182cfa4acebce147aedf328181f9c4930f14498fd03c0af6b0cce25be99452a81d f4fa30a53eddbb7bb7b203adf8764a0ccd9db6913a576d68d642d8fd47452590137869c25d9ff83 d68ebe6d616056a8425b85b52e69715b8b85ae807b84638d8f00e321b65e4c33acaf6469e18e302 03010001 ----- recipient: 30819f300d06092a864886f70d010101050003818d0030818902818100bb064c99c492144a9f463 480273aba93ac1db1f0da3cb9f3c1f9d058cf499fd8e54d244da0a8dd6ddd329ec86794b04d773e b4841c9f935ea4d9ccc2821c7a1082d23b6c928d59863407f52fa05d8b47e5157f8fe56c2ce3279 c657f9c6a80500073b0be8093f748aef667c03e64f04f84d311c4d866c12d79d3fc3034563dfb02 03010001 ----- value: 5.0 ----- time: 2019-01-14 16:18:01.868241 ----- -------------- =====================================