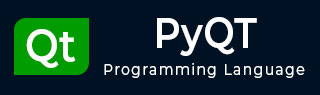
- PyQt5 Tutorial
- PyQt5 - Home
- PyQt5 - Introduction
- PyQt5 - What’s New
- PyQt5 - Hello World
- PyQt5 - Major Classes
- PyQt5 - Using Qt Designer
- PyQt5 - Signals & Slots
- PyQt5 - Layout Management
- PyQt5 - Basic Widgets
- PyQt5 - QDialog Class
- PyQt5 - QMessageBox
- PyQt5 - Multiple Document Interface
- PyQt5 - Drag & Drop
- PyQt5 - Database Handling
- PyQt5 - Drawing API
- PyQt5 - BrushStyle Constants
- PyQt5 - QClipboard
- PyQt5 - QPixmap Class
- PyQt5 Useful Resources
- PyQt5 - Quick Guide
- PyQt5 - Useful Resources
- PyQt5 - Discussion
PyQt5 - QCheckBox Widget
A rectangular box before the text label appears when a QCheckBox object is added to the parent window. Just as QRadioButton, it is also a selectable button. Its common use is in a scenario when the user is asked to choose one or more of the available options.
Unlike Radio buttons, check boxes are not mutually exclusive by default. In order to restrict the choice to one of the available items, the check boxes must be added to QButtonGroup.
The following table lists commonly used QCheckBox class methods −
Sr.No. | Methods & Description |
---|---|
1 |
setChecked() Changes the state of checkbox button |
2 |
setText() Sets the label associated with the button |
3 |
text() Retrieves the caption of the button |
4 |
isChecked() Checks if the button is selected |
5 |
setTriState() Provides no change state to checkbox |
Each time a checkbox is either checked or cleared, the object emits stateChanged() signal.
Example
Here, two QCheckBox objects are added to a horizontal layout. Their stateChanged() signal is connected to btnstate() function. The source object of signal is passed to the function using lambda.
self.b1.stateChanged.connect(lambda:self.btnstate(self.b1)) self.b2.toggled.connect(lambda:self.btnstate(self.b2))
The isChecked() function is used to check if the button is checked or not.
if b.text() == "Button1": if b.isChecked() == True: print b.text()+" is selected" else: print b.text()+" is deselected"
The complete code is as follows −
import sys from PyQt5.QtCore import * from PyQt5.QtGui import * from PyQt5.QtWidgets import * class checkdemo(QWidget): def __init__(self, parent = None): super(checkdemo, self).__init__(parent) layout = QHBoxLayout() self.b1 = QCheckBox("Button1") self.b1.setChecked(True) self.b1.stateChanged.connect(lambda:self.btnstate(self.b1)) layout.addWidget(self.b1) self.b2 = QCheckBox("Button2") self.b2.toggled.connect(lambda:self.btnstate(self.b2)) layout.addWidget(self.b2) self.setLayout(layout) self.setWindowTitle("checkbox demo") def btnstate(self,b): if b.text() == "Button1": if b.isChecked() == True: print b.text()+" is selected" else: print b.text()+" is deselected" if b.text() == "Button2": if b.isChecked() == True: print b.text()+" is selected" else: print b.text()+" is deselected" def main(): app = QApplication(sys.argv) ex = checkdemo() ex.show() sys.exit(app.exec_()) if __name__ == '__main__': main()
Output
The above code produces the following output −
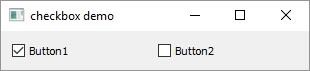
Button2 is selected Button2 is deselected Button1 is deselected Button1 is selected
As mentioned earlier, checkBox buttons can be made mutually exclusive by adding them in the QButtonGroup object.
self.bg = QButtonGroup() self.bg.addButton(self.b1,1) self.bg.addButton(self.b2,2)
QButtonGroup object, provides abstract container for buttons and doesn’t have a visual representation. It emits buttonCliked() signal and sends Button object’s reference to the slot function btngroup().
self.bg.buttonClicked[QAbstractButton].connect(self.btngroup)
The btngroup() function displays the caption of the clicked checkbox.
def btngroup(self,btn): print btn.text()+" is selected"
After above changes, the application window appears as follows −

Button1 is selected Button2 is selected Button1 is selected