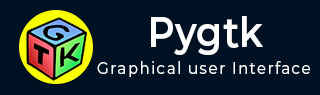
- PyGTK Tutorial
- PyGTK - Home
- PyGTK - Introduction
- PyGTK - Environment
- PyGTK - Hello World
- PyGTK - Important Classes
- PyGTK - Window Class
- PyGTK - Button Class
- PyGTK - Label CLass
- PyGTK - Entry Class
- PyGTK - Signal Handling
- PyGTK - Event Handling
- PyGTK - Containers
- PyGTK - Box Class
- PyGTK - ButtonBox Class
- PyGTK - Alignment Class
- PyGTK - EventBox Class
- PyGTK - Layout Class
- PyGTK - ComboBox Class
- PyGTK - ToggleButton Class
- PyGTK - CheckButton Class
- PyGTK - RadioButton Class
- PyGTK - MenuBar, Menu & MenuItem
- PyGTK - Toolbar Class
- PyGTK - Adjustment Class
- PyGTK - Range Class
- PyGTK - Scale Class
- PyGTK - Scrollbar Class
- PyGTK - Dialog Class
- PyGTK - MessageDialog Class
- PyGTK - AboutDialog Class
- PyGTK - Font Selection Dialog
- PyGTK - Color Selection Dialog
- PyGTK - File Chooser Dialog
- PyGTK - Notebook Class
- PyGTK - Frame Class
- PyGTK - AspectFrame Class
- PyGTK - TreeView Class
- PyGTK - Paned Class
- PyGTK - Statusbar Class
- PyGTK - ProgressBar Class
- PyGTK - Viewport Class
- PyGTK - Scrolledwindow Class
- PyGTK - Arrow Class
- PyGTK - Image Class
- PyGTK - DrawingArea Class
- PyGTK - SpinButton Class
- PyGTK - Calendar Class
- PyGTK - Clipboard Class
- PyGTK - Ruler Class
- PyGTK - Timeout
- PyGTK - Drag and Drop
- PyGTK Useful Resources
- PyGTK - Quick Guide
- PyGTK - Useful Resources
- PyGTK - Discussion
PyGTK - SpinButton Class
The SpinnButton widget, often called the Spinner is a gtk.Entry widget with up and down arrows on its right. A user can type in a numeric value directly in it or increment or decrement using up and down arrows. The gtk.SpinButton class is inherited from the gtk.Entry class. It uses a gtk.Adjustment object with which the range and step of the numeric value in the spinner can be restricted.
The SpinButton widget is created using the following constructor −
sp = gtk.SpinButton(adj, climb_rate, digits)
Here, adj represents the gtk.Adjustment object controlling range, climb_rate is an acceleration factor and the number of decimals specified by digits.
The gtk.SpinButton class has the following methods −
SpinButton.set_adjustment() − This sets the "adjustment" property.
SpinButton.set_digits() − This sets the "digits" property to the value to determine the number of decimal places to be displayed by the spinbutton.
SpinButton.set_increments(step, page) − This sets the step value which has increment applied for each left mousebutton press and page value which is increment applied for each middle mousebutton press.
SpinButton.set_range() − This sets the minimum and maximum allowable values for spinbutton.
SpinButton.set_value() − This sets the spin button to a new value programmatically.
SpinButton.update_policy() − The valid values are gtk.UPDATE_ALWAYS and gtk.UPDATE_VALID
SpinButton.spin(direction, increment=1) − This increments or decrements Spinner's value in the specified direction.
The following are the predefined direction constants −
gtk.SPIN_STEP_FORWARD | forward by step_increment |
gtk.SPIN_STEP_BACKWARD | backward by step_increment |
gtk.SPIN_PAGE_FORWARD | forward by step_increment |
gtk.SPIN_PAGE_BACKWARD | backward by step_increment |
gtk.SPIN_HOME | move to minimum value |
gtk.SPIN_END | move to maximum value |
gtk.SPIN_USER_DEFINED | add increment to the value |
SpinButton.set_wrap() — If wrap is True, the spin button value wraps around to the opposite limit when the upper or lower limit of the range exceeds.
The gtk.SpinButton widget emits the following signals −
change-value | This is emitted when the spinbutton value is changed by keyboard action |
input | This is emitted when the value changes. |
output | This is emitted when the spinbutton display value is changed. Returns True if the handler successfully sets the text and no further processing is required. |
value-changed | This is emitted when any of the settings that change the display of the spinbutton is changed. |
wrapped | This is emitted right after the spinbutton wraps from its maximum to minimum value or vice-versa. |
Example
The following example constructs a simple Date Selector by using three SpinButton widgets. The Day Selector is applied an Adjustment object to restrict value between 1—31. The second selector is for the number of months 1—12. The third selector selects the year range 2000—2020.
Observe the code −
import gtk class PyApp(gtk.Window): def __init__(self): super(PyApp, self).__init__() self.set_title("SpinButton Demo") self.set_size_request(300, 200) self.set_position(gtk.WIN_POS_CENTER) self.set_border_width(20) vbox = gtk.VBox(False, 5) hbox = gtk.HBox(True, 3) lbl1 = gtk.Label("Date") hbox.add(lbl1) adj1 = gtk.Adjustment(1.0, 1.0, 31.0, 1.0, 5.0, 0.0) spin1 = gtk.SpinButton(adj1, 0, 0) spin1.set_wrap(True) hbox.add(spin1) lbl2 = gtk.Label("Month") hbox.add(lbl2) adj2 = gtk.Adjustment(1.0, 1.0, 12.0, 1.0, 5.0, 0.0) spin2 = gtk.SpinButton(adj2, 0, 0) spin2.set_wrap(True) hbox.add(spin2) lbl3 = gtk.Label("Year") hbox.add(lbl3) adj3 = gtk.Adjustment(1.0, 2000.0, 2020.0, 1.0, 5.0, 0.0) spin3 = gtk.SpinButton(adj3, 0, 0) spin3.set_wrap(True) hbox.add(spin3) frame = gtk.Frame() frame.add(hbox) frame.set_label("Date of Birth") vbox.add(frame) self.add(vbox) self.connect("destroy", gtk.main_quit) self.show_all() PyApp() gtk.main()
Upon execution, the above code will produce the following output −
