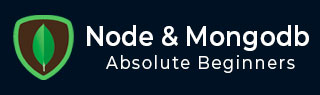
- Node & MongoDB Tutorial
- Node & MongoDB - Home
- Node & MongoDB - Overview
- Node & MongoDB - Environment Setup
- Node & MongoDB Examples
- Node & MongoDB - Connect Database
- Node & MongoDB - Show Databases
- Node & MongoDB - Drop Database
- Node & MongoDB - Create Collection
- Node & MongoDB - Drop Collection
- Node & MongoDB - Display Collections
- Node & MongoDB - Insert Document
- Node & MongoDB - Select Document
- Node & MongoDB - Update Document
- Node & MongoDB - Delete Document
- Node & MongoDB - Embedded Documents
- Node & MongoDB - Limiting Records
- Node & MongoDB - Sorting Records
- Node & MongoDB Useful Resources
- Node & MongoDB - Quick Guide
- Node & MongoDB - Useful Resources
- Node & MongoDB - Discussion
Node & MongoDB - Quick Guide
Node & MongoDB - Overview
What is Node.js?
Node.js is a server-side platform built on Google Chrome's JavaScript Engine (V8 Engine). Node.js was developed by Ryan Dahl in 2009 and its latest version is v0.10.36. The definition of Node.js as supplied by its official documentation is as follows −
Node.js is a platform built on Chrome's JavaScript runtime for easily building fast and scalable network applications. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
Node.js is an open source, cross-platform runtime environment for developing server-side and networking applications. Node.js applications are written in JavaScript, and can be run within the Node.js runtime on OS X, Microsoft Windows, and Linux.
Node.js also provides a rich library of various JavaScript modules which simplifies the development of web applications using Node.js to a great extent.
Node.js = Runtime Environment + JavaScript Library
mongodb
mongodb is node.js driver to connect and perform database operations on MongoDB. To install mongodb, run the following npm command.
npm install mongodb + mongodb@3.6.9 added 1 package from 1 contributor in 1.781s
Creating/Connecting to Database
Once mongoClient is instantiated, its connect() method can be used to get connection to a database.
// MongoDBClient const client = new MongoClient(url, { useUnifiedTopology: true }); // make a connection to the database client.connect(function(error) { if (error) throw error; console.log("Connected!"); // create or connect to database const db = client.db(database); // close the connection client.close(); });
In case database is not present then above command will create the same.
In subsequent chapters, we'll see the various operations on MongoDB using Node.
Node & MongoDB - Environment Setup
Install MongoDB database
Follow the MongoDB installation steps using MongoDB - Environment
Install Node
Live Demo Option Online
You really do not need to set up your own environment to start learning Node.js. Reason is very simple, we already have set up Node.js environment online, so that you can execute all the available examples online and learn through practice. Feel free to modify any example and check the results with different options.
Try the following example using the Live Demo option available at the top right corner of the below sample code box (on our website) −
/* Hello World! program in Node.js */ console.log("Hello World!");
For most of the examples given in this tutorial, you will find a Try it option, so just make use of it and enjoy your learning.
Local Environment Setup
If you are still willing to set up your environment for Node.js, you need the following two softwares available on your computer, (a) Text Editor and (b) The Node.js binary installables.
Text Editor
This will be used to type your program. Examples of few editors include Windows Notepad, OS Edit command, Brief, Epsilon, EMACS, and vim or vi.
Name and version of text editor can vary on different operating systems. For example, Notepad will be used on Windows, and vim or vi can be used on windows as well as Linux or UNIX.
The files you create with your editor are called source files and contain program source code. The source files for Node.js programs are typically named with the extension ".js".
Before starting your programming, make sure you have one text editor in place and you have enough experience to write a computer program, save it in a file, and finally execute it.
The Node.js Runtime
The source code written in source file is simply javascript. The Node.js interpreter will be used to interpret and execute your javascript code.
Node.js distribution comes as a binary installable for SunOS , Linux, Mac OS X, and Windows operating systems with the 32-bit (386) and 64-bit (amd64) x86 processor architectures.
Following section guides you on how to install Node.js binary distribution on various OS.
Download Node.js archive
Download latest version of Node.js installable archive file from Node.js Downloads. At the time of writing this tutorial, following are the versions available on different OS.
OS | Archive name |
---|---|
Windows | node-v12.16.1-x64.msi |
Linux | node-v12.16.1-linux-x86.tar.gz |
Mac | node-v12.16.1-darwin-x86.tar.gz |
SunOS | node-v12.16.1-sunos-x86.tar.gz |
Installation on UNIX/Linux/Mac OS X, and SunOS
Based on your OS architecture, download and extract the archive node-v12.16.1-osname.tar.gz into /tmp, and then finally move extracted files into /usr/local/nodejs directory. For example:
$ cd /tmp $ wget http://nodejs.org/dist/v12.16.1/node-v12.16.1-linux-x64.tar.gz $ tar xvfz node-v12.16.1-linux-x64.tar.gz $ mkdir -p /usr/local/nodejs $ mv node-v12.16.1-linux-x64/* /usr/local/nodejs
Add /usr/local/nodejs/bin to the PATH environment variable.
OS | Output |
---|---|
Linux | export PATH=$PATH:/usr/local/nodejs/bin |
Mac | export PATH=$PATH:/usr/local/nodejs/bin |
FreeBSD | export PATH=$PATH:/usr/local/nodejs/bin |
Installation on Windows
Use the MSI file and follow the prompts to install the Node.js. By default, the installer uses the Node.js distribution in C:\Program Files\nodejs. The installer should set the C:\Program Files\nodejs\bin directory in window's PATH environment variable. Restart any open command prompts for the change to take effect.
Verify installation: Executing a File
Create a js file named main.js on your machine (Windows or Linux) having the following code.
/* Hello, World! program in node.js */ console.log("Hello, World!")
Now execute main.js file using Node.js interpreter to see the result −
$ node main.js
If everything is fine with your installation, this should produce the following result −
Hello, World!
mongodb
mongodb is node.js driver to connect and perform database operations on MongoDB. To install mongodb, run the following npm command.
npm install mongodb + mongodb@3.6.9 added 1 package from 1 contributor in 1.781s
Node & MongoDB - Connecting Database
Node mongodb provides mongoClient object which is used to connect a database connection using connect() method. This function takes multiple parameters and provides db object to do database operations.
Syntax
// MongoDBClient const client = new MongoClient(url, { useUnifiedTopology: true }); // make a connection to the database client.connect();
You can disconnect from the MongoDB database anytime using another connection object function close().
Syntax
client.close()
Example
Try the following example to connect to a MongoDB server −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected!
Node & MongoDB - Show Databases
To show databases, you can use admin.listDatabases() method to get the name of all the databases where admin represents the admin class.
MongoClient.connect(url, function(error, client) { // Use the admin database for the operation const adminDb = client.db('myDb').admin(); // List all the available databases adminDb.listDatabases(function(err, dbs) { console.log(dbs); }); });
Example
Try the following example to connect to a MongoDB server −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Use the admin database for the operation const adminDb = client.db('myDb').admin(); // List all the available databases adminDb.listDatabases(function(err, dbs) { console.log(dbs); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! { databases: [ { name: 'admin', sizeOnDisk: 40960, empty: false }, { name: 'config', sizeOnDisk: 36864, empty: false }, { name: 'local', sizeOnDisk: 73728, empty: false } ], totalSize: 151552, ok: 1 }
Node & MongoDB - Drop Database
To drop a database, you can use database.drop() method to drop the selected database.
MongoClient.connect(url, function(error, client) { // Connect to the database const database = client.db('myDb'); // Drop the database database.dropDatabase(); });
Example
Try the following example to drop a mongodb database −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); // Drop the database database.dropDatabase(); console.log("Database dropped!"); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! Database dropped!
Node & MongoDB - Create Collection
To create a collection, you can use database.createCollection() method to create a collection.
MongoClient.connect(url, function(error, client) { // Connect to the database const database = client.db('myDb'); // Create the collection database.createCollection('sampleCollection'); });
Example
Try the following example to create a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); // Create the collection database.createCollection('sampleCollection'); console.log("Collection created."); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! Collection created.
Node & MongoDB - Drop Collection
To drop a collection, you can use collection.drop() method to drop a collection.
MongoClient.connect(url, function(error, client) { // Connect to the database const database = client.db('myDb'); // drop the collection database.collection('sampleCollection').drop(function(error, status) { if (error) throw error; if (status) { console.log("Collection deleted"); } }); });
Example
Try the following example to drop a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); // Create the collection database.collection('sampleCollection').drop(function(error, status) { if (error) throw error; if (status) { console.log("Collection deleted."); } }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! Collection deleted.
Node & MongoDB - Display Collections
To display collections of a database, you can use database.listCollections() method to get list of collections.
MongoClient.connect(url, function(error, client) { // Connect to the database const database = client.db('myDb'); // get the list of collections database.listCollections().toArray(function(err, collections) { collections.forEach(collection => console.log(collection.name)); }); });
Example
Try the following example to create a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); // Create the collection database.createCollection('sampleCollection'); database.listCollections().toArray(function(err, collections) { collections.forEach(collection => console.log(collection.name)); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! sampleCollection
Node & MongoDB - Insert Document
To insert document(s) in a collection of a database, you can use collection.insertOne() or collection.insertMany() methods to insert one or multiple documents.
database.collection("sampleCollection").insertOne(firstDocument, function(error, res) { if (error) throw error; console.log("1 document inserted"); }); database.collection("sampleCollection").insertMany(documents, function(error, res) { if (error) throw error; console.log("Documents inserted: " + res.insertedCount); });
Example
Try the following example to insert documents in a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; const firstDocument = { First_Name : 'Mahesh', Last_Name : 'Parashar', Date_Of_Birth: '1990-08-21', e_mail: 'mahesh_parashar.123@gmail.com', phone: '9034343345' }; const documents = [{ First_Name : 'Radhika', Last_Name : 'Sharma', Date_Of_Birth: '1995-09-26', e_mail: 'radhika_sharma.123@gmail.com', phone: '9000012345' }, { First_Name : 'Rachel', Last_Name : 'Christopher', Date_Of_Birth: '1990-02-16', e_mail: 'rachel_christopher.123@gmail.com', phone: '9000054321' }, { First_Name : 'Fathima', Last_Name : 'Sheik', Date_Of_Birth: '1990-02-16', e_mail: 'fathima_sheik.123@gmail.com', phone: '9000012345' } ]; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); database.collection("sampleCollection").insertOne(firstDocument, function(error, res) { if (error) throw error; console.log("1 document inserted"); }); database.collection("sampleCollection").insertMany(documents, function(error, res) { if (error) throw error; console.log("Documents inserted: " + res.insertedCount); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Documents inserted: 3 1 document inserted
Node & MongoDB - Select Documents
To select documents of a collection, you can use collection.findOne() or collection.find() methods to select one or multiple documents.
database.collection("sampleCollection").findOne({}, function(error, result) { if (error) throw error; console.log(result); }); database.collection("sampleCollection").find({}).toArray(function(error, result) { if (error) throw error; console.log(result); });
Example
Try the following example to select documents in a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); database.collection("sampleCollection").findOne({}, function(error, result) { if (error) throw error; console.log(result); }); database.collection("sampleCollection").find({}).toArray(function(error, result) { if (error) throw error; console.log(result); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! { _id: 60c4bbb40f8c3920a0e30fdd, First_Name: 'Radhika', Last_Name: 'Sharma', Date_Of_Birth: '1995-09-26', e_mail: 'radhika_sharma.123@gmail.com', phone: '9000012345' } [ { _id: 60c4bbb40f8c3920a0e30fdd, First_Name: 'Radhika', Last_Name: 'Sharma', Date_Of_Birth: '1995-09-26', e_mail: 'radhika_sharma.123@gmail.com', phone: '9000012345' }, { _id: 60c4bbb40f8c3920a0e30fde, First_Name: 'Rachel', Last_Name: 'Christopher', Date_Of_Birth: '1990-02-16', e_mail: 'rachel_christopher.123@gmail.com', phone: '9000054321' }, { _id: 60c4bbb40f8c3920a0e30fdf, First_Name: 'Fathima', Last_Name: 'Sheik', Date_Of_Birth: '1990-02-16', e_mail: 'fathima_sheik.123@gmail.com', phone: '9000012345' }, { _id: 60c4bbb40f8c3920a0e30fdc, First_Name: 'Mahesh', Last_Name: 'Parashar', Date_Of_Birth: '1990-08-21', e_mail: 'mahesh_parashar.123@gmail.com', phone: '9034343345' } ]
Node & MongoDB - Update Documents
To update documents of a collection, you can use collection.updateOne() or collection.updateMany() methods to update one or multiple documents.
database.collection("sampleCollection").updateOne(query,updates, function(error, result) { if (error) throw error; console.log('Document Updated'); }); database.collection("sampleCollection").updateMany(query,updates, function(error, result) { if (error) throw error; console.log(result.result.nModified + " document(s) updated"); });
Example
Try the following example to update a document in a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); database.collection("sampleCollection").updateOne({First_Name:'Mahesh'}, { $set: { e_mail: 'maheshparashar@gmail.com' } }, function(error, result) { if (error) throw error; console.log('Document Updated.'); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! Document Updated.
Node & MongoDB - Delete Documents
To delete documents of a collection, you can use collection.deleteOne() or collection.deleteMany() methods to delete one or multiple documents.
database.collection("sampleCollection").deleteOne(query, function(error, result) { if (error) throw error; console.log('Document deleted.'); }); database.collection("sampleCollection").deleteMany(query, function(error, result) { if (error) throw error; console.log(result.result.n + " document(s) deleted."); });
Example
Try the following example to delete a document in a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); database.collection("sampleCollection").deleteOne({First_Name:'Mahesh'}, function(error, result) { if (error) throw error; console.log('Document Deleted.'); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! Document Deleted.
Node & MongoDB - Embedded Document
To insert embedded document(s) in a collection of a database, you can use collection.insertOne() or collection.insertMany() methods to insert one or multiple documents.
database.collection("sampleCollection").insertOne(firstDocument, function(error, res) { if (error) throw error; console.log("1 document inserted"); }); database.collection("sampleCollection").insertMany(documents, function(error, res) { if (error) throw error; console.log("Documents inserted: " + res.insertedCount); });
Example
Try the following example to insert documents in a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; const firstPost = { title : 'MongoDB Overview', description : 'MongoDB is no SQL database', by: 'tutorials point', url: 'http://www.tutorialspoint.com', comments: [{ user: 'user1', message: 'My First Comment', dateCreated: '20/2/2020', like: 0 }, { user: 'user2', message: 'My Second Comment', dateCreated: '20/2/2020', like: 0 }] }; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('posts'); database.collection("samplePost").insertOne(firstPost, function(error, res) { if (error) throw error; console.log("1 document inserted"); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected. 1 document inserted
Node & MongoDB - Limit Records
To limit the selected documents of a collection, you can use collection.find().limit() methods to select required documents.
database.collection("sampleCollection").find({}).limit(2).toArray(function(error, result) { if (error) throw error; console.log(result); });
Example
Try the following example to select limited documents in a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); database.collection("sampleCollection").find({}).limit(2).toArray(function(error, result) { if (error) throw error; console.log(result); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! [ { _id: 60c4bbb40f8c3920a0e30fdd, First_Name: 'Radhika', Last_Name: 'Sharma', Date_Of_Birth: '1995-09-26', e_mail: 'radhika_sharma.123@gmail.com', phone: '9000012345' }, { _id: 60c4bbb40f8c3920a0e30fde, First_Name: 'Rachel', Last_Name: 'Christopher', Date_Of_Birth: '1990-02-16', e_mail: 'rachel_christopher.123@gmail.com', phone: '9000054321' } ]
Node & MongoDB - Sorting Records
To sort the selected documents of a collection, you can use collection.find().sort() methods to sort documents.
database.collection("sampleCollection").find({}).sort({First_Name: -1}).toArray(function(error, result) { if (error) throw error; console.log(result); });
Example
Try the following example to select limited documents in a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); database.collection("sampleCollection").find({}).sort({First_Name: -1}).toArray(function(error, result) { if (error) throw error; console.log(result); }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! [ { _id: 60c4bbb40f8c3920a0e30fdd, First_Name: 'Radhika', Last_Name: 'Sharma', Date_Of_Birth: '1995-09-26', e_mail: 'radhika_sharma.123@gmail.com', phone: '9000012345' }, { _id: 60c4bbb40f8c3920a0e30fde, First_Name: 'Rachel', Last_Name: 'Christopher', Date_Of_Birth: '1990-02-16', e_mail: 'rachel_christopher.123@gmail.com', phone: '9000054321' }, { _id: 60c4bbb40f8c3920a0e30fdf, First_Name: 'Fathima', Last_Name: 'Sheik', Date_Of_Birth: '1990-02-16', e_mail: 'fathima_sheik.123@gmail.com', phone: '9000012345' } ]
To Continue Learning Please Login