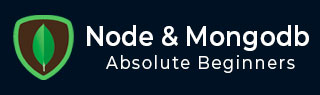
- Node & MongoDB Tutorial
- Node & MongoDB - Home
- Node & MongoDB - Overview
- Node & MongoDB - Environment Setup
- Node & MongoDB Examples
- Node & MongoDB - Connect Database
- Node & MongoDB - Show Databases
- Node & MongoDB - Drop Database
- Node & MongoDB - Create Collection
- Node & MongoDB - Drop Collection
- Node & MongoDB - Display Collections
- Node & MongoDB - Insert Document
- Node & MongoDB - Select Document
- Node & MongoDB - Update Document
- Node & MongoDB - Delete Document
- Node & MongoDB - Embedded Documents
- Node & MongoDB - Limiting Records
- Node & MongoDB - Sorting Records
- Node & MongoDB Useful Resources
- Node & MongoDB - Quick Guide
- Node & MongoDB - Useful Resources
- Node & MongoDB - Discussion
Node & MongoDB - Drop Collection
To drop a collection, you can use collection.drop() method to drop a collection.
MongoClient.connect(url, function(error, client) { // Connect to the database const database = client.db('myDb'); // drop the collection database.collection('sampleCollection').drop(function(error, status) { if (error) throw error; if (status) { console.log("Collection deleted"); } }); });
Example
Try the following example to drop a mongodb collection −
Copy and paste the following example as mongodb_example.js −
const MongoClient = require('mongodb').MongoClient; // Prepare URL const url = "mongodb://localhost:27017/"; // make a connection to the database MongoClient.connect(url, function(error, client) { if (error) throw error; console.log("Connected!"); // Connect to the database const database = client.db('myDb'); // Create the collection database.collection('sampleCollection').drop(function(error, status) { if (error) throw error; if (status) { console.log("Collection deleted."); } }); // close the connection client.close(); });
Output
Execute the mysql_example.js script using node and verify the output.
node mongodb_example.js Connected! Collection deleted.
Advertisements
To Continue Learning Please Login