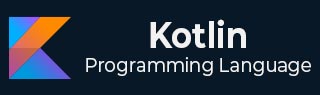
- Kotlin Tutorial
- Kotlin - Home
- Kotlin - Overview
- Kotlin - Environment Setup
- Kotlin - Architecture
- Kotlin - Basic Syntax
- Kotlin - Comments
- Kotlin - Keywords
- Kotlin - Variables
- Kotlin - Data Types
- Kotlin - Operators
- Kotlin - Booleans
- Kotlin - Strings
- Kotlin - Arrays
- Kotlin - Ranges
- Kotlin - Functions
- Kotlin Control Flow
- Kotlin - Control Flow
- Kotlin - if...Else Expression
- Kotlin - When Expression
- Kotlin - For Loop
- Kotlin - While Loop
- Kotlin - Break and Continue
- Kotlin Collections
- Kotlin - Collections
- Kotlin - Lists
- Kotlin - Sets
- Kotlin - Maps
- Kotlin Objects and Classes
- Kotlin - Class and Objects
- Kotlin - Constructors
- Kotlin - Inheritance
- Kotlin - Abstract Classes
- Kotlin - Interface
- Kotlin - Visibility Control
- Kotlin - Extension
- Kotlin - Data Classes
- Kotlin - Sealed Class
- Kotlin - Generics
- Kotlin - Delegation
- Kotlin - Destructuring Declarations
- Kotlin - Exception Handling
- Kotlin Useful Resources
- Kotlin - Quick Guide
- Kotlin - Useful Resources
- Kotlin - Discussion
Kotlin - When Expression
Consider a situation when you have large number of conditions to check. Though you can use if..else if expression to handle the situation, but Kotlin provides when expression to handle the situation in nicer way. Using when expression is far easy and more clean in comparison to writing many if...else if expressions. Kotlin when expression evaluates a section of code among many alternatives as explained in below example.
Kotlin when matches its argument against all branches sequentially until some branch condition is satisfied.
Kotlin when expression is similar to the switch statement in C, C++ and Java.
Example
fun main(args: Array<String>) { val day = 2 val result = when (day) { 1 -> "Monday" 2 -> "Tuesday" 3 -> "Wednesday" 4 -> "Thursday" 5 -> "Friday" 6 -> "Saturday" 7 -> "Sunday" else -> "Invalid day." } println(result) }
When you run the above Kotlin program, it will generate the following output:
Tuesday
Kotlin when as Statement
Kotlin when can be used either as an expression or as a statement, simply like a switch statement in Java. If it is used as an expression, the value of the first matching branch becomes the value of the overall expression.
Example
Let's write above example once again without using expression form:
fun main(args: Array<String>) { val day = 2 when (day) { 1 -> println("Monday") 2 -> println("Tuesday") 3 -> println("Wednesday") 4 -> println("Thursday") 5 -> println("Friday") 6 -> println("Saturday") 7 -> println("Sunday") else -> println("Invalid day.") } }
When you run the above Kotlin program, it will generate the following output:
Tuesday
Combine when Conditions
We can combine multiple when conditions into a single condition.
Example
fun main(args: Array<String>) { val day = 2 when (day) { 1, 2, 3, 4, 5 -> println("Weekday") else -> println("Weekend") } }
When you run the above Kotlin program, it will generate the following output:
Weekday
Range in when Conditions
Kotlin ranges are created using double dots .. and we can use them while checking when condition with the help of in operator.
Example
fun main(args: Array<String>) { val day = 2 when (day) { in 1..5 -> println("Weekday") else -> println("Weekend") } }
When you run the above Kotlin program, it will generate the following output:
Weekday
Expression in when Conditions
Kotlin when can use arbitrary expressions instead of a constant as branch condition.
Example
fun main(args: Array<String>) { val x = 20 val y = 10 val z = 10 when (x) { (y+z) -> print("y + z = x = $x") else -> print("Condition is not satisfied") } }
When you run the above Kotlin program, it will generate the following output:
y + z = x = 20
Kotlin when with block of code
Kotlin when braches can be put as block of code enclosed within curly braces.
Example
fun main(args: Array<String>) { val day = 2 when (day) { 1 -> { println("First day of the week") println("Monday") } 2 -> { println("Second day of the week") println("Tuesday") } 3 -> { println("Third day of the week") println("Wednesday") } 4 -> println("Thursday") 5 -> println("Friday") 6 -> println("Saturday") 7 -> println("Sunday") else -> println("Invalid day.") } }
When you run the above Kotlin program, it will generate the following output:
Second day of the week Tuesday
Quiz Time (Interview & Exams Preparation)
Q 1 - Which of the following is true about Kotlin when expression?
A - It is used to compare a single value against multiple conditions
B - Kotlin when expression can be used in place of if..else if expression
C - Kotlin when branches can be integer, string, array or ranges
Answer : D
Explanation
All the mentioned statements are correct about Kotlin when expression.
Answer : A
Explanation
Yes it is true that Kotlin when can be used as an expression as well as a statement.
Q 3 - Kotlin when is inspired by which of the following Java statement
Answer : A
Explanation
Kotlin when has very similar syntax and functionality like switch statement available in Java.