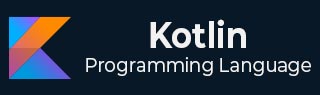
- Kotlin Tutorial
- Kotlin - Home
- Kotlin - Overview
- Kotlin - Environment Setup
- Kotlin - Architecture
- Kotlin - Basic Syntax
- Kotlin - Comments
- Kotlin - Keywords
- Kotlin - Variables
- Kotlin - Data Types
- Kotlin - Operators
- Kotlin - Booleans
- Kotlin - Strings
- Kotlin - Arrays
- Kotlin - Ranges
- Kotlin - Functions
- Kotlin Control Flow
- Kotlin - Control Flow
- Kotlin - if...Else Expression
- Kotlin - When Expression
- Kotlin - For Loop
- Kotlin - While Loop
- Kotlin - Break and Continue
- Kotlin Collections
- Kotlin - Collections
- Kotlin - Lists
- Kotlin - Sets
- Kotlin - Maps
- Kotlin Objects and Classes
- Kotlin - Class and Objects
- Kotlin - Constructors
- Kotlin - Inheritance
- Kotlin - Abstract Classes
- Kotlin - Interface
- Kotlin - Visibility Control
- Kotlin - Extension
- Kotlin - Data Classes
- Kotlin - Sealed Class
- Kotlin - Generics
- Kotlin - Delegation
- Kotlin - Destructuring Declarations
- Kotlin - Exception Handling
- Kotlin Useful Resources
- Kotlin - Quick Guide
- Kotlin - Useful Resources
- Kotlin - Discussion
Kotlin - Variables
Variables are an important part of any programming. They are the names you give to computer memory locations which are used to store values in a computer program and later you use those names to retrieve the stored values and use them in your program.
Kotlin variables are created using either var or val keywords and then an equal sign = is used to assign a value to those created variables.
Syntax
Following is a simple syntax to create two variables and then assign them different values:
var name = "Zara Ali" var age = 19 var height = 5.2
Examples
Once a variable is created and assigned a value, later we can access its value using its name as follows:
fun main() { var name = "Zara Ali" var age = 19 println(name) println(age) }
When you run the above Kotlin program, it will generate the following output:
Zara Ali 19
Let's see one more example where we will access variable values using dollar sign $:
fun main() { var name = "Zara Ali" var age = 19 println("Name = $name") println("Age = $age") }
When you run the above Kotlin program, it will generate the following output:
Name = Zara Ali Age = 19
Let's see one more example to display the variable values without using a dollar sign as below:
fun main() { var name = "Zara Ali" var age = 19 println("Name = " + name) println("Age = " + age) }
When you run the above Kotlin program, it will generate the following output:
Name = Zara Ali Age = 19
Kotlin Mutable Variables
Mutable means that the variable can be reassigned to a different value after initial assignment. To declare a mutable variable, we use the var keyword as we have used in the above examples:
fun main() { var name = "Zara Ali" var age = 19 println("Name = $name") println("Age = $age") name = "Nuha Ali" age = 11 println("Name = $name") println("Age = $age") }
When you run the above Kotlin program, it will generate the following output:
Name = Zara Ali Age = 19 Name = Nuha Ali Age = 11
Kotlin Read-only Variables
A read-only variable can be declared using val (instead of var) and once a value is assigned, it can not be re-assigned.
fun main() { val name = "Zara Ali" val age = 19 println("Name = $name") println("Age = $age") name = "Nuha Ali" // Not allowed, throws an exception age = 11 println("Name = $name") println("Age = $age") }
When you run the above Kotlin program, it will generate the following output:
main.kt:8:4: error: val cannot be reassigned name = "Nuha Ali" // Not allowed, throws an exception ^ main.kt:9:4: error: val cannot be reassigned age = 11 ^
Read-only vs Mutable
The Mutable variables will be used to define variables, which will keep charging their values based on different conditions during program execution.
You will use Read-only variable to define different constant values i.e. the variables which will retain their value throughout of the program.
Kotlin Variable Types
Kotlin is smart enough to recognise that "Zara Ali" is a string, and that 19 is a number variable. However, you can explicitly specify a variable type while creating it:
fun main() { var name: String = "Zara Ali" var age: Int = 19 println("Name = $name") println("Age = $age") name = "Nuha Ali" age = 11 println("Name = $name") println("Age = $age") }
When you run the above Kotlin program, it will generate the following output:
Name = Zara Ali Age = 19 Name = Nuha Ali Age = 11
Soon we will learn more about different data types available in Kotlin which can be used to create different type of variables.
Kotlin Variable Naming Rules
There are certain rules to be followed while naming the Kotlin variables:
Kotlin variable names can contain letters, digits, underscores, and dollar signs.
Kotlin variable names should start with a letter or underscores
Kotlin variables are case sensitive which means Zara and ZARA are two different variables.
Kotlin variable can not have any white space or other control characters.
Kotlin variable can not have names like var, val, String, Int because they are reserved keywords in Kotlin.
Quiz Time (Interview & Exams Preparation)
Q 1 - Which of the following statements is correct about Kotlin Variables:
A - Kotlin variables are used to store the information during program execution.
B - Kotlin variables can be read-only (Not changeable) and mutable (Changeable)
Answer : D
Explanation
All the given three statements are correct for Kotlin variables.
Q 2 - Identify which line of the following program will raise an error:
var name = "Zara Ali" val age = 19 name = "Nuha Ali" age = 11
Answer : D
Explanation
At the last line we are trying to change the value of a read-only variable, so it will raise and exception at this line.
Q 3 - Which one is a wrong variable name in Kotlin
Answer : E
Explanation
As per given rules to define a variable name in Kotlin, all the give variable names are invalid
Q 4 - Which of the following statment is not correct about Kotlin variable
A - Kotlin variables are case sensitive.
B - Kotlin can differentiate between different datatypes without explicitly specifying them.
C - Kotlin variables can be accessed in multiple ways.
D - Kotlin variable name can use any Kotlin reserved keyword
Answer : D
Explanation
Last statement is not correct because we can not make use of a reserved keyword to name a Kotlin variable. Rest of the statements are correct.