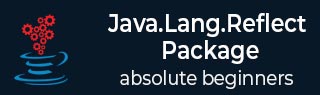
- java.lang.reflect Package Classes
- java.lang.reflect - Home
- java.lang.reflect - AccessibleObject
- java.lang.reflect - Array
- java.lang.reflect - Constructor<T>
- java.lang.reflect - Field
- java.lang.reflect - Method
- java.lang.reflect - Modifier
- java.lang.reflect - Proxy
- java.lang.reflect Package Extras
- java.lang.reflect - Interfaces
- java.lang.reflect - Exceptions
- java.lang.reflect - Error
- java.lang.reflect Useful Resources
- java.lang.reflect - Quick Guide
- java.lang.reflect - Useful Resources
- java.lang.reflect - Discussion
java.lang.reflect - Quick Guide
java.lang.reflect - AccessibleObject Class
Introduction
The java.lang.reflect.AccessibleObject class is the base class for Field, Method and Constructor objects. It provides the ability to flag a reflected object as suppressing default Java language access control checks when it is used. The access checks for public, default (package) access, protected, and private members are performed when Fields, Methods or Constructors are used to set or get fields, to invoke methods, or to create and initialize new instances of classes, respectively. Setting the accessible flag in a reflected object permits sophisticated applications with sufficient privilege, such as Java Object Serialization or other persistence mechanisms, to manipulate objects in a manner that would normally be prohibited.
Class declaration
Following is the declaration for java.lang.reflect.AccessibleObject class −
public class AccessibleObject extends Object implements AnnotatedElement
Constructors
Sr.No. | Constructor & Description |
---|---|
1 | protected AccessibleObject()
Constructor: only used by the Java Virtual Machine. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | <T extends Annotation> T getAnnotation(Class<T> annotationClass)
Returns this element's annotation for the specified type if such an annotation is present, else null. |
2 | Annotation[] getAnnotations()
Returns all annotations present on this element. |
3 | Annotation[] getDeclaredAnnotations()
Returns all annotations that are directly present on this element. |
4 | boolean isAccessible()
Get the value of the accessible flag for this object. |
5 | boolean isAnnotationPresent(Class<? extends Annotation> annotationClass)
Returns true if an annotation for the specified type is present on this element, else false. |
6 | static void setAccessible(AccessibleObject[] array, boolean flag)
Convenience method to set the accessible flag for an array of objects with a single security check (for efficiency). |
7 | void setAccessible(boolean flag)
Set the accessible flag for this object to the indicated boolean value. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.Object
java.lang.reflect - Array Class
Introduction
The java.lang.reflect.Array class provides static methods to dynamically create and access Java arrays. Array permits widening conversions to occur during a get or set operation, but throws an IllegalArgumentException if a narrowing conversion would occur.
Class declaration
Following is the declaration for java.lang.reflect.Array class −
public final class Array extends Object
Class methods
Sr.No. | Method & Description |
---|---|
1 | static Object get(Object array, int index)
Returns the value of the indexed component in the specified array object. |
2 | static boolean getBoolean(Object array, int index)
Returns the value of the indexed component in the specified array object, as a boolean. |
3 | static byte getByte(Object array, int index)
Returns the value of the indexed component in the specified array object, as a byte. |
4 | static char getChar(Object array, int index)
Returns the value of the indexed component in the specified array object, as a char. |
5 | static double getDouble(Object array, int index)
Returns the value of the indexed component in the specified array object, as a double. |
6 | static float getFloat(Object array, int index)
Returns the value of the indexed component in the specified array object, as a float. |
7 | static int getInt(Object array, int index)
Returns the value of the indexed component in the specified array object, as an int. |
8 | static int getLength(Object array)
Returns the length of the specified array object, as an int. |
9 | static long getLong(Object array, int index)
Returns the value of the indexed component in the specified array object, as a long. |
10 | static short getShort(Object array, int index)
Returns the value of the indexed component in the specified array object, as a short. |
11 | static Object newInstance(Class<?> componentType, int... dimensions)
Creates a new array with the specified component type and dimensions. |
12 | static Object newInstance(Class<?> componentType, int length)
Creates a new array with the specified component type and length. |
13 | static void set(Object array, int index, Object value)
Sets the value of the indexed component of the specified array object to the specified new value. |
14 | static void setBoolean(Object array, int index, boolean z)
Sets the value of the indexed component of the specified array object to the specified boolean value. |
15 | static void setByte(Object array, int index, byte b)
Sets the value of the indexed component of the specified array object to the specified byte value. |
16 | static void setChar(Object array, int index, char c)
Sets the value of the indexed component of the specified array object to the specified char value. |
17 | static void setDouble(Object array, int index, double d)
Sets the value of the indexed component of the specified array object to the specified double value. |
18 | static void setFloat(Object array, int index, float f)
Sets the value of the indexed component of the specified array object to the specified float value. |
19 | static void setInt(Object array, int index, int i)
Sets the value of the indexed component of the specified array object to the specified int value. |
20 | static void setLong(Object array, int index, long l)
Sets the value of the indexed component of the specified array object to the specified long value. |
21 | static void setShort(Object array, int index, short s)
Sets the value of the indexed component of the specified array object to the specified short value. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.Object
java.lang.reflect - Constructor<T> Class
Introduction
The java.lang.reflect.Constructor class provides information about, and access to, a single constructor for a class. Constructor permits widening conversions to occur when matching the actual parameters to newInstance() with the underlying constructor's formal parameters, but throws an IllegalArgumentException if a narrowing conversion would occur.
Class declaration
Following is the declaration for java.lang.reflect.Constructor class −
public final class Constructor<T> extends AccessibleObject implements GenericDeclaration, Member
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean equals(Object obj)
Compares this Constructor against the specified object. |
2 | <T extends Annotation> T getAnnotation(Class<T> annotationClass)
Returns this element's annotation for the specified type if such an annotation is present, else null. |
3 | Annotation[] getDeclaredAnnotations()
Returns all annotations that are directly present on this element. |
4 | Class<T> getDeclaringClass()
Returns the Class object representing the class that declares the constructor represented by this Constructor object. |
5 | Class<?>[] getExceptionTypes()
Returns an array of Class objects that represent the types of exceptions declared to be thrown by the underlying constructor represented by this Constructor object. |
6 | Type[] getGenericExceptionTypes()
Returns an array of Type objects that represent the exceptions declared to be thrown by this Constructor object. |
7 | Type[] getGenericParameterTypes()
Returns an array of Type objects that represent the formal parameter types, in declaration order, of the method represented by this Constructor object. |
8 | int getModifiers()
Returns the Java language modifiers for the constructor represented by this Constructor object, as an integer. |
9 | String getName()
Returns the name of this constructor, as a string. |
10 | Annotation[][] getParameterAnnotations()
Returns an array of arrays that represent the annotations on the formal parameters, in declaration order, of the method represented by this Constructor object. |
11 | Class<?>[] getParameterTypes()
Returns an array of Class objects that represent the formal parameter types, in declaration order, of the constructor represented by this Constructor object. |
12 | int hashCode()
Returns a hashcode for this Constructor. |
13 | boolean isSynthetic()
Returns true if this constructor is a synthetic constructor; returns false otherwise. |
14 | boolean isVarArgs()
Returns true if this constructor was declared to take a variable number of arguments; returns false otherwise. |
15 | T newInstance(Object... initargs)
Uses the constructor represented by this Constructor object to create and initialize a new instance of the constructor's declaring class, with the specified initialization parameters. |
16 | String toGenericString()
Returns a string describing this Constructor, including type parameters. |
17 | String toString()
Returns a string describing this Constructor. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.reflect.AccessibleObject
- java.lang.Object
java.lang.reflect - Field Class
Introduction
The java.lang.reflect.Field class provides information about, and dynamic access to, a single field of a class or an interface. The reflected field may be a class (static) field or an instance field. A Field permits widening conversions to occur during a get or set access operation, but throws an IllegalArgumentException if a narrowing conversion would occur.
Class declaration
Following is the declaration for java.lang.reflect.Field class −
public final class Field extends AccessibleObject implements Member
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean equals(Object obj)
Compares this Field against the specified object. |
2 | Object get(Object obj)
Returns the value of the field represented by this Field, on the specified object. |
3 | <T extends Annotation> T getAnnotation(Class<T> annotationClass)
Returns this element's annotation for the specified type if such an annotation is present, else null. |
4 | boolean getBoolean(Object obj)
Gets the value of a static or instance boolean field. |
5 | byte getByte(Object obj)
Gets the value of a static or instance byte field. |
6 | char getChar(Object obj)
Gets the value of a static or instance field of type char or of another primitive type convertible to type char via a widening conversion. |
7 | Annotation[] getDeclaredAnnotations()
Returns all annotations that are directly present on this element. |
8 | Class<?> getDeclaringClass()
Returns the Class object representing the class or interface that declares the field represented by this Field object. |
9 | double getDouble(Object obj)
Gets the value of a static or instance field of type double or of another primitive type convertible to type double via a widening conversion. |
10 | float getFloat(Object obj)
Gets the value of a static or instance field of type float or of another primitive type convertible to type float via a widening conversion. |
11 | Type getGenericType()
Returns a Type object that represents the declared type for the field represented by this Field object. |
12 | int getInt(Object obj)
Gets the value of a static or instance field of type int or of another primitive type convertible to type int via a widening conversion. |
13 | long getLong(Object obj)
Gets the value of a static or instance field of type long or of another primitive type convertible to type long via a widening conversion. |
14 | int getModifiers()
Returns the Java language modifiers for the field represented by this Field object, as an integer. |
15 | String getName()
RReturns the name of the field represented by this Field object. |
16 | short getShort(Object obj)
Gets the value of a static or instance field of type short or of another primitive type convertible to type short via a widening conversion. |
17 | Class<?> getType()
Returns a Class object that identifies the declared type for the field represented by this Field object. |
18 | int hashCode()
Returns a hashcode for this Field. |
19 | boolean isEnumConstant()
Returns true if this field represents an element of an enumerated type; returns false otherwise. |
20 | boolean isSynthetic()
This method returns true if the field represented by the current object is synthetic, else it returns false. |
21 | void setBoolean(Object obj, boolean z)
Sets the value of a field as a boolean on the specified object. |
22 | void setByte(Object obj, byte b)
Sets the value of a field as a byte on the specified object. |
23 | void setChar(Object obj, char c)
Sets the value of a field as a char on the specified object. |
24 | void setDouble(Object obj, double d)
Sets the value of a field as a double on the specified object. |
25 | void setFloat(Object obj, float f)
Sets the value of a field as a float on the specified object. |
26 | void setInt(Object obj, int i)
Sets the value of a field as an int on the specified object. |
27 | void setLong(Object obj, long l)
Sets the value of a field as a long on the specified object. |
28 | void setShort(Object obj, short s)
Sets the value of a field as a short on the specified object. |
29 | String toGenericString()
Returns a string describing this Field, including its generic type. |
30 | String toString()
Returns a string describing this Field. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.reflect.AccessibleObject
- java.lang.Object
java.lang.reflect - Method Class
Introduction
The java.lang.reflect.Method class provides information about, and access to, a single method on a class or interface. The reflected method may be a class method or an instance method (including an abstract method). A Method permits widening conversions to occur when matching the actual parameters to invoke with the underlying method's formal parameters, but it throws an IllegalArgumentException if a narrowing conversion would occur.
Class declaration
Following is the declaration for java.lang.reflect.Method class −
public final class Method<T> extends AccessibleObject implements GenericDeclaration, Member
Class methods
Sr.No. | Method & Description |
---|---|
1 | boolean equals(Object obj)
Compares this Method against the specified object. |
2 | <T extends Annotation> T getAnnotation(Class<T> annotationClass)
Returns this element's annotation for the specified type if such an annotation is present, else null. |
3 | Annotation[] getDeclaredAnnotations()
Returns all annotations that are directly present on this element. |
4 | Class<T> getDeclaringClass()
Returns the Class object representing the class that declares the method represented by this Method object. |
5 | Object getDefaultValue()
Returns the default value for the annotation member represented by this Method instance. |
6 | Class<?>[] getExceptionTypes()
Returns an array of Class objects that represent the types of exceptions declared to be thrown by the underlying constructor represented by this Constructor object. |
7 | Type[] getGenericExceptionTypes()
Returns an array of Type objects that represent the exceptions declared to be thrown by this Constructor object. |
8 | Type[] getGenericParameterTypes()
Returns an array of Type objects that represent the formal parameter types, in declaration order, of the method represented by this Constructor object. |
9 | Type getGenericReturnType()
Returns a Type object that represents the formal return type of the method represented by this Method object. |
10 | int getModifiers()
Returns the Java language modifiers for the method represented by this Method object, as an integer. |
11 | String getName()
Returns the name of this method, as a string. |
12 | Annotation[][] getParameterAnnotations()
Returns an array of arrays that represent the annotations on the formal parameters, in declaration order, of the method represented by this Method object. |
13 | Class<?>[] getParameterTypes()
Returns an array of Class objects that represent the formal parameter types, in declaration order, of the constructor represented by this Method object. |
14 | Class<?> getReturnType()
Returns a Class object that represents the formal return type of the method represented by this Method object. |
15 | int hashCode()
Returns a hashcode for this Constructor. |
16 | Object invoke(Object obj, Object... args)
Invokes the underlying method represented by this Method object, on the specified object with the specified parameters. |
17 | boolean isBridge()
Returns true if this method is a bridge method; returns false otherwise. |
18 | boolean isSynthetic()
Returns true if this method is a synthetic method; returns false otherwise. |
19 | boolean isVarArgs()
Returns true if this method was declared to take a variable number of arguments; returns false otherwise. |
20 | String toGenericString()
Returns a string describing this Method, including type parameters. |
21 | String toString()
Returns a string describing this Method. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.reflect.AccessibleObject
- java.lang.Object
java.lang.reflect - Modifier Class
Introduction
The java.lang.reflect.Modifier class provides static methods and constants to decode class and member access modifiers. The sets of modifiers are represented as integers with distinct bit positions representing different modifiers. The values for the constants representing the modifiers are taken from the tables in sections 4.1, 4.4, 4.5, and 4.7 of The Java Virtual Machine Specification.
Class declaration
Following is the declaration for java.lang.reflect.Modifier class −
public class Modifier extends Object
Fields
Following are the fields for java.lang.reflect.Modifier class −
static int ABSTRACT − The int value representing the abstract modifier.
static int FINAL − The int value representing the final modifier.
static int INTERFACE − The int value representing the interface modifier.
static int NATIVE − The int value representing the native modifier.
static int PRIVATE − The int value representing the private modifier.
static int PROTECTED − The int value representing the protected modifier.
static int PUBLIC − The int value representing the public modifier.
static int STATIC − The int value representing the static modifier.
static int STRICT − The int value representing the strictfp modifier.
static int SYNCHRONIZED − The int value representing the synchronized modifier.
static int TRANSIENT − The int value representing the transient modifier.
static int VOLATILE − The int value representing the volatile modifier.
Constructors
Sr.No. | Constructor & Description |
---|---|
1 | Modifier()
Default Constructor. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | static int classModifiers()
Return an int value OR-ing together the source language modifiers that can be applied to a class. |
2 | static int constructorModifiers()
Return an int value OR-ing together the source language modifiers that can be applied to a constructor. |
3 | static int fieldModifiers()
Return an int value OR-ing together the source language modifiers that can be applied to a field. |
4 | static int interfaceModifiers()
Return an int value OR-ing together the source language modifiers that can be applied to an interface. |
5 | static boolean isAbstract(int mod)
Return true if the integer argument includes the abstract modifier, false otherwise. |
6 | static boolean isFinal(int mod)
Return true if the integer argument includes the final modifier, false otherwise. |
7 | static boolean isInterface(int mod)
Return true if the integer argument includes the interface modifier, false otherwise. |
8 | static boolean isNative(int mod)
Return true if the integer argument includes the native modifier, false otherwise. |
9 | static boolean isPrivate(int mod)
Return true if the integer argument includes the private modifier, false otherwise. |
10 | static boolean isProtected(int mod)
Return true if the integer argument includes the protected modifier, false otherwise. |
11 | static boolean isPublic(int mod)
Return true if the integer argument includes the public modifier, false otherwise. |
12 | static boolean isStatic(int mod)
Return true if the integer argument includes the static modifier, false otherwise. |
13 | static boolean isStrict(int mod)
Return true if the integer argument includes the strictfp modifier, false otherwise. |
14 | static boolean isSynchronized(int mod)
Return true if the integer argument includes the synchronized modifier, false otherwise. |
15 | static boolean isTransient(int mod)
Return true if the integer argument includes the transient modifier, false otherwise. |
16 | static boolean isVolatile(int mod)
Return true if the integer argument includes the volatile modifier, false otherwise. |
17 | static int methodModifiers()
Return an int value OR-ing together the source language modifiers that can be applied to a method. |
18 | static String toString(int mod)
Return a string describing the access modifier flags in the specified modifier. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.Object
java.lang.reflect - Proxy Class
Introduction
The java.lang.reflect.Proxy class provides static methods for creating dynamic proxy classes and instances, and it is also the superclass of all dynamic proxy classes created by those methods.
Class declaration
Following is the declaration for java.lang.reflect.Proxy class −
public class Proxy extends Object implements Serializable
Fields
Following are the fields for java.lang.reflect.Proxy class −
protected InvocationHandler h − the invocation handler for this proxy instance.
Constructors
Sr.No. | Constructor & Description |
---|---|
1 | protected Proxy(InvocationHandler h)
Constructs a new Proxy instance from a subclass (typically, a dynamic proxy class) with the specified value for its invocation handler. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | static InvocationHandler getInvocationHandler(Object proxy)
Returns the invocation handler for the specified proxy instance. |
2 | static Class<?> getProxyClass(ClassLoader loader, Class<?>... interfaces)
Returns the java.lang.Class object for a proxy class given a class loader and an array of interfaces. |
3 | static boolean isProxyClass(Class<?> cl)
Returns true if and only if the specified class was dynamically generated to be a proxy class using the getProxyClass method or the newProxyInstance method. |
4 | static Object newProxyInstance(ClassLoader loader, Class<?>[] interfaces, InvocationHandler h)
Returns an instance of a proxy class for the specified interfaces that dispatches method invocations to the specified invocation handler. |
Methods inherited
This class inherits methods from the following classes −
- java.lang.Object
java.lang.reflect - Interfaces
Introduction
The java.lang.reflect Interfaces contains the interfaces which are used to obtain reflective information about classes and objects.
Interface Summary
Sr.No. | Interface & Description |
---|---|
1 | AnnotatedElement Represents an annotated element of the program currently running in this VM. |
2 | GenericArrayType GenericArrayType represents an array type whose component type is either a parameterized type or a type variable. |
3 | GenericDeclaration A common interface for all entities that declare type variables. |
4 | InvocationHandler InvocationHandler is the interface implemented by the invocation handler of a proxy instance. |
5 | Member Member is an interface that reflects identifying information about a single member (a field or a method) or a constructor. |
6 | ParameterizedType ParameterizedType represents a parameterized type such as Collection<String>. |
7 | Type Type is the common superinterface for all types in the Java programming language. |
8 | List<E> This is an ordered collection (also known as a sequence). |
9 | TypeVariable<D extends GenericDeclaration> TypeVariable is the common superinterface for type variables of kinds. |
10 | WildcardType WildcardType represents a wildcard type expression, such as ?, ? extends Number, or ? super Integer. |
java.lang.reflect - Exceptions
Introduction
The java.lang.reflect Exceptions contains the exceptions which can occur during reflection operations.
Exceptions Summary
Sr.No. | Exception & Description |
---|---|
1 | InvocationTargetException InvocationTargetException is a checked exception that wraps an exception thrown by an invoked method or constructor. |
2 | MalformedParameterizedTypeException Thrown when a semantically malformed parameterized type is encountered by a reflective method that needs to instantiate it. |
3 | UndeclaredThrowableException Thrown by a method invocation on a proxy instance if its invocation handler's invoke method throws a checked exception (a Throwable that is not assignable to RuntimeException or Error) that is not assignable to any of the exception types declared in the throws clause of the method that was invoked on the proxy instance and dispatched to the invocation handler. |
java.lang.reflect - Error
Introduction
The java.lang.reflect Error contains the error which can occur during reflection operations.
Errors Summary
Sr.No. | Error & Description |
---|---|
1 | GenericSignatureFormatError Thrown when a syntactically malformed signature attribute is encountered by a reflective method that needs to interpret the generic signature information for a type, method or constructor. |