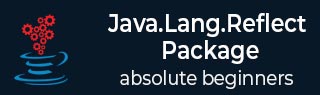
- java.lang.reflect Package Classes
- java.lang.reflect - Home
- java.lang.reflect - AccessibleObject
- java.lang.reflect - Array
- java.lang.reflect - Constructor<T>
- java.lang.reflect - Field
- java.lang.reflect - Method
- java.lang.reflect - Modifier
- java.lang.reflect - Proxy
- java.lang.reflect Package Extras
- java.lang.reflect - Interfaces
- java.lang.reflect - Exceptions
- java.lang.reflect - Error
- java.lang.reflect Useful Resources
- java.lang.reflect - Quick Guide
- java.lang.reflect - Useful Resources
- java.lang.reflect - Discussion
java.lang.reflect.Field.get() Method Example
Description
The java.lang.reflect.Field.get(Object obj) method returns the value of the field represented by this Field, on the specified object. The value is automatically wrapped in an object if it has a primitive type.
Declaration
Following is the declaration for java.lang.reflect.Field.get(Object obj) method.
public Object get(Object obj) throws IllegalArgumentException, IllegalAccessException
Parameters
obj − object from which the represented field's value is to be extracted.
Returns
the value of the represented field in object obj; primitive values are wrapped in an appropriate object before being returned.
Exceptions
IllegalAccessException − if this Field object is enforcing Java language access control and the underlying field is inaccessible.
IllegalArgumentException − if the specified object is not an instance of the class or interface declaring the underlying field (or a subclass or implementor thereof).
NullPointerException − if the specified object is null and the field is an instance field.
ExceptionInInitializerError − if the initialization provoked by this method fails.
Example
The following example shows the usage of java.lang.reflect.Field.get(Object obj) method.
package com.tutorialspoint; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.reflect.Field; public class FieldDemo { public static void main(String[] args) throws NoSuchFieldException, SecurityException, IllegalArgumentException, IllegalAccessException { SampleClass sampleObject = new SampleClass(); sampleObject.setSampleField("data"); Field field = SampleClass.class.getField("sampleField"); System.out.println(field.get(sampleObject)); } } @CustomAnnotation(name = "SampleClass", value = "Sample Class Annotation") class SampleClass { @CustomAnnotation(name="sampleClassField", value = "Sample Field Annotation") public String sampleField; public String getSampleField() { return sampleField; } public void setSampleField(String sampleField) { this.sampleField = sampleField; } } @Retention(RetentionPolicy.RUNTIME) @interface CustomAnnotation { public String name(); public String value(); }
Let us compile and run the above program, this will produce the following result −
data