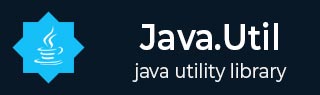
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java TreeSet remove() Method
Description
The Java TreeSet remove(Object o) method is used to remove the specified element from this set if it is present.
Declaration
Following is the declaration for java.util.TreeSet.remove() method.
public boolean remove(Object o)
Parameters
o − This is the object to be removed from this set, if present
Return Value
The method call returns true if this set contained the specified element.
Exception
ClassCastException − This is thrown if the specified object cannot be compared with the elements currently in this set.
NullPointerException − This is thrown if the specified element is null and this set uses natural ordering, or its comparator does not permit null elements.
Removing an Element of a TreeSet of Integer Example
The following example shows the usage of Java TreeSet remove() method to remove an entry from the treeset. We've created a TreeSet object of Integer. Then few entries are added using add() method. Using remove() method, an element is removed and tree is printed.
package com.tutorialspoint; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet<Integer> treeset = new TreeSet<>(); // adding in the tree set treeset.add(1); treeset.add(13); treeset.add(17); treeset.add(2); // removing 17 from the set System.out.println("Remove 17: "+treeset.remove(17)); // displaying the Tree set data System.out.println("Tree set : " + treeset); } }
Output
Let us compile and run the above program, this will produce the following result.
Remove 17: true Tree set : [1, 2, 13]
Removing an Element of a TreeSet of String Example
The following example shows the usage of Java TreeSet remove() method to remove an entry from the treeset. We've created a TreeSet object of String. Then few entries are added using add() method. Using remove() method, an element is removed and tree is printed.
package com.tutorialspoint; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet<String> treeset = new TreeSet<>(); // adding in the tree set treeset.add("A"); treeset.add("C"); treeset.add("B"); treeset.add("D"); // removing B from the set System.out.println("Remove B: "+treeset.remove("B")); // displaying the Tree set data System.out.println("Tree set : " + treeset); } }
Output
Let us compile and run the above program, this will produce the following result.
Remove B: true Tree set : [A, C, D]
Removing an Element of a TreeSet of Object Example
The following example shows the usage of Java TreeSet remove() method to remove an entry from the treeset. We've created a TreeSet object of Student. Then few entries are added using add() method. Using remove() method, an element is removed and tree is printed.
package com.tutorialspoint; import java.util.TreeSet; public class TreeSetDemo { public static void main(String[] args) { // creating a TreeSet TreeSet <Student>treeset = new TreeSet<>(); // adding in the tree set treeset.add(new Student(2, "Julie")); treeset.add(new Student(1, "Robert")); treeset.add(new Student(3, "Adam")); treeset.add(new Student(4, "Julia")); // removing Adam from the set System.out.println("Remove Adam: "+treeset.remove(new Student(3, "Adam"))); // displaying the Tree set data System.out.println("Tree set : " + treeset); } } class Student implements Comparable<Student> { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } @Override public int compareTo(Student student) { return this.rollNo - student.rollNo; } }
Output
Let us compile and run the above program, this will produce the following result.
Remove Adam: true Tree set : [[ 1, Robert ], [ 2, Julie ], [ 4, Julia ]]
To Continue Learning Please Login