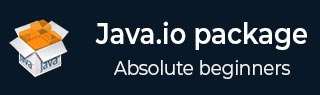
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java.io.DataInputStream Class
Introduction
The Java.io.DataInputStream class lets an application read primitive Java data types from an underlying input stream in a machine-independent way.Following are the important points about DataInputStream −
An application uses a data output stream to write data that can later be read by a data input stream.
DataInputStream is not necessarily safe for multithreaded access. Thread safety is optional and is the responsibility of users of methods in this class.
Class declaration
Following is the declaration for Java.io.DataInputStream class −
public class DataInputStream extends FilterInputStream implements DataInput
Field
Following are the fields for Java.io.DataInputStream class −
protected InputStream in − This is the input stream to be filtered.
Class constructors
Sr.No. | Constructor & Description |
---|---|
1 | DataInputStream(InputStream in) This creates a DataInputStream that uses the specified underlying InputStream. |
Class methods
Sr.No. | Method & Description |
---|---|
1 | int read(byte[] b)
This method reads some number of bytes from the contained input stream and stores them into the buffer array b |
2 | int read(byte[] b, int off, int len)
This method reads up to len bytes of data from the contained input stream into an array of bytes. |
3 | boolean readBoolean()
This method reads one input byte and returns true if that byte is nonzero, false if that byte is zero. |
4 | byte readByte()
This method reads and returns one input byte. |
5 | char readChar()
This method reads two input bytes and returns a char value. |
6 | double readDouble()
This method reads eight input bytes and returns a double value. |
7 | float readFloat()
This method reads four input bytes and returns a float value. |
8 | void readFully(byte[] b)
This method reads some bytes from an input stream and stores them into the buffer array b. |
9 | void readFully(byte[] b, int off, int len)
This method reads len bytes from an input stream. |
10 | int readInt()
This method reads four input bytes and returns an int value. |
11 | long readLong()
This method reads eight input bytes and returns a long value. |
12 | short readShort()
This method reads two input bytes and returns a short value. |
13 | int readUnsignedByte()
This method reads one input byte, zero-extends it to type int, and returns the result, which is therefore in the range 0 through 255. |
14 | int readUnsignedShort()
This method reads two input bytes and returns an int value in the range 0 through 65535. |
15 | String readUTF()
This method reads in a string that has been encoded using a modified UTF-8 format. |
16 | static String readUTF(DataInput in)
This method reads from the stream in a representation of a Unicode character string encoded in modified UTF-8 format; this string of characters is then returned as a String. |
17 | int skipBytes(int n)
This method makes an attempt to skip over n bytes of data from the input stream, discarding the skipped bytes. |
Methods inherited
This class inherits methods from the following classes −
- Java.io.FilterInputStream
- Java.io.Object