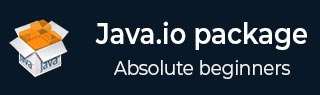
- Java.io package classes
- Java.io - Home
- Java.io - BufferedInputStream
- Java.io - BufferedOutputStream
- Java.io - BufferedReader
- Java.io - BufferedWriter
- Java.io - ByteArrayInputStream
- Java.io - ByteArrayOutputStream
- Java.io - CharArrayReader
- Java.io - CharArrayWriter
- Java.io - Console
- Java.io - DataInputStream
- Java.io - DataOutputStream
- Java.io - File
- Java.io - FileDescriptor
- Java.io - FileInputStream
- Java.io - FileOutputStream
- Java.io - FilePermission
- Java.io - FileReader
- Java.io - FileWriter
- Java.io - FilterInputStream
- Java.io - FilterOutputStream
- Java.io - FilterReader
- Java.io - FilterWriter
- Java.io - InputStream
- Java.io - InputStreamReader
- Java.io - LineNumberInputStream
- Java.io - LineNumberReader
- Java.io - ObjectInputStream
- Java.io - ObjectInputStream.GetField
- Java.io - ObjectOutputStream
- io - ObjectOutputStream.PutField
- Java.io - ObjectStreamClass
- Java.io - ObjectStreamField
- Java.io - OutputStream
- Java.io - OutputStreamWriter
- Java.io - PipedInputStream
- Java.io - PipedOutputStream
- Java.io - PipedReader
- Java.io - PipedWriter
- Java.io - PrintStream
- Java.io - PrintWriter
- Java.io - PushbackInputStream
- Java.io - PushbackReader
- Java.io - RandomAccessFile
- Java.io - Reader
- Java.io - SequenceInputStream
- Java.io - SerializablePermission
- Java.io - StreamTokenizer
- Java.io - StringBufferInputStream
- Java.io - StringReader
- Java.io - StringWriter
- Java.io - Writer
- Java.io package extras
- Java.io - Interfaces
- Java.io - Exceptions
- Java.io package Useful Resources
- Java.io - Discussion
Java - File exists() Method
Description
The Java File exists() method tests the existence of the file or directory defined by this abstract pathname.
Declaration
Following is the declaration for java.io.File.exists() method −
public boolean exists()
Parameters
NA
Return Value
The method returns boolean true, if and only if the file defined by the abstract pathname exists; else false.
Exception
NA
Example 1
The following example shows the usage of Java File exists() method. We've created a File reference. Then we're creating a File Object using test.txt which is not present in the current directory. Then we've created the file using createNewFile() method. Now using exists() method, we're checking if file is created or not. Then we're deleting the file if file exists and status is printed after checking it again using exists() method.
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new files f = new File("test.txt"); // create new file in the system f.createNewFile(); // tests if file exists bool = f.exists(); // prints System.out.println("File exists: "+bool); if(bool == true) { // delete() invoked f.delete(); System.out.println("delete() invoked"); } // tests if file exists bool = f.exists(); // prints System.out.print("File exists: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
Output
Let us compile and run the above program, this will produce the following result −
File exists: true delete() invoked File exists: false
Example 2
The following example shows the usage of Java File exists() method. We've created a File reference. Then we're creating a File Object using F:/test.txt which is present in the provided directory. Now using exists() method, we're checking if file is present.
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new files f = new File("F:/test.txt"); // tests if file exists bool = f.exists(); // prints System.out.println("File exists: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
Output
Let us compile and run the above program, this will produce the following result −
File exists: true
Example 3
The following example shows the usage of Java File exists() method. We've created a File reference. Then we're creating a File Object using F:/test directory which is present in the provided location. Now using exists() method, we're checking if directory is present.
package com.tutorialspoint; import java.io.File; public class FileDemo { public static void main(String[] args) { File f = null; boolean bool = false; try { // create new files f = new File("F:/test"); // tests if file exists bool = f.exists(); // prints System.out.println("Directory exists: "+bool); } catch(Exception e) { // if any error occurs e.printStackTrace(); } } }
Output
Let us compile and run the above program, this will produce the following result −
Directory exists: true