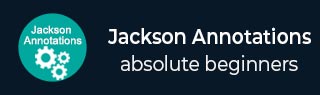
- Jackson Annotations Tutorial
- Jackson - Home
- Serialization Annotations
- Jackson - @JsonAnyGetter
- Jackson - @JsonGetter
- @JsonPropertyOrder
- Jackson - @JsonRawValue
- Jackson - @JsonValue
- Jackson - @JsonRootName
- Jackson - @JsonSerialize
- Deserialization Annotations
- Jackson - @JsonCreator
- Jackson - @JacksonInject
- Jackson - @JsonAnySetter
- Jackson - @JsonSetter
- Jackson - @JsonDeserialize
- @JsonEnumDefaultValue
- Property Inclusion Annotations
- @JsonIgnoreProperties
- Jackson - @JsonIgnore
- Jackson - @JsonIgnoreType
- Jackson - @JsonInclude
- Jackson - @JsonAutoDetect
- Type Handling Annotations
- Jackson - @JsonTypeInfo
- Jackson - @JsonSubTypes
- Jackson - @JsonTypeName
- General Annotations
- Jackson - @JsonProperty
- Jackson - @JsonFormat
- Jackson - @JsonUnwrapped
- Jackson - @JsonView
- @JsonManagedReference
- @JsonBackReference
- Jackson - @JsonIdentityInfo
- Jackson - @JsonFilter
- Miscellaneous
- Custom Annotation
- MixIn Annotations
- Disable Annotation
- Jackson Annotations Resources
- Jackson - Quick Guide
- Jackson - Useful Resources
- Jackson - Discussion
Jackson Annotations - @JsonAnySetter
@JsonAnySetter allows a setter method to use Map which is then used to deserialize the additional properties of JSON in the similar fashion as other properties.
Example @JsonAnySetter
import java.io.IOException; import java.util.HashMap; import java.util.Map; import com.fasterxml.jackson.annotation.JsonAnySetter; import com.fasterxml.jackson.databind.ObjectMapper; public class JacksonTester { public static void main(String args[]){ ObjectMapper mapper = new ObjectMapper(); String jsonString = "{\"RollNo\" : \"1\",\"Name\" : \"Mark\"}"; try { Student student = mapper.readerFor(Student.class).readValue(jsonString); System.out.println(student.getProperties().get("Name")); System.out.println(student.getProperties().get("RollNo")); } catch (IOException e) { e.printStackTrace(); } } } class Student { private Map<String, String> properties; public Student(){ properties = new HashMap<>(); } public Map<String, String> getProperties(){ return properties; } @JsonAnySetter public void add(String property, String value){ properties.put(property, value); } }
Output
Mark 1
Advertisements