
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Constants
- C - Literals
- C - Escape sequences
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming Mock Test
This section presents you various set of Mock Tests related to C Programming Framework. You can download these sample mock tests at your local machine and solve offline at your convenience. Every mock test is supplied with a mock test key to let you verify the final score and grade yourself.
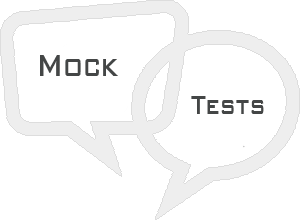
C Programming Mock Test IV
Q 1 - What actually get pass when you pass an array as a function argument?
A - First value of elements in array
Answer : B
Explanation
By passing the name of an array as a function argument; the name contains the base address of an array and the base address (array value) get updated in the main function.
Q 2 - In the given below code, the function fopen()uses "r" to open the file “source.txt” in binary mode for which purpose?
#include<stdio.h> int main () { FILE *fp; fp = fopen("source.txt", "r"); return 0; }
Answer : A
Explanation
To open a file in C programming, we can use library function fopen(). In the given above code, fopen() function is opening a file “source.txt” for reading. Here, “r” stands for reading. If, fopen() function does not find any file for reading, returns NULL
#include<stdio.h> int main () { FILE *fp; fp = fopen("source.txt", "r"); return 0; }
Q 3 - In DOS, how many bytes exist for near, far and huge pointers?
Answer : C
Explanation
In DOS, numbers of byte exist for near pointer = 2, far pointer = 4 and huge pointer = 4.
In Windows and Linux, numbers of byte exist for near pointer = 4, far pointer = 4 and huge pointer = 4.
Q 4 - fgets() function is safer than gets() because in fgets() function you can specify the size of the buffer into which the supplied string will be stored.
Answer : A
Explanation
Both functions retrive and store a string from console or file, but fgets() functions are more safer to use then gets() because gets() doesn't facilitate to detail the length of the buffer to store the string in and fgets() facilitates to specify a maximum string length.
char *fgets(char *s, int size, FILE *stream); char *gets(char *s);
Q 5 - Which scanf() statement will you use to scan a float value (a) and double value (b)?
Float a; Double b;
Answer : D
Explanation
In C Programming, the scanf() can be used to read a formatted string from the stdin stream, where as; scanf() uses %lf to read a double value and %f to read a floating-point number in fixed decimal format.
int scanf(const char *format, ...)
Q 6 - Choose the correct statement that is a combination of these two statements,
Statement 1: char *p; Statement 2: p = (char*) malloc(100);
B - char *p = (char*)malloc(100);
Answer : B
Explanation
ptr = (data type *)malloc(size);
The given above code is a prototype of malloc() function, where ptr indicates the pointer.
char *p = (char*)malloc(100);
In this code, “*p” is a pointer of type char and malloc() function allocates the memory for char.
Q 7 - Which of the following header file can be used to define the NULL macro?
A - stdio.h, locale.h, stddef.h, stdlib.h, string.h,
B - stddef.h, locale.h, math.h, stdlib.h, string.h,
Answer : A
Explanation
In C library, NULL Macro is the value of a null pointer constant. It may be defined as ((void*)0), 0 or 0L depending on the compiler merchant.
Q 8 - In the given below code, the P2 is
Typedef int *ptr; ptr p1, p2;
Answer : B
Explanation
Ptr is an alias to int*.
Q 9 - In the following code, what is 'P'?
Typedef char *charp; const charp P;
Answer : A
Explanation
Const charp P;
Although, the code itself indicates the keyword "const", so that; P is a constant.
Q 10 - What is x in the following program?
#include<stdio.h> int main () { typedef char (*(*arrfptr[3])())[10]; arrfptr x return 0; }
Answer : C
Explanation
Here, x is an array of three function pointers
#include<stdio.h> int main () { typedef char (*(*arrfptr[3])())[10]; arrfptr x return 0; }
Q 11 - What will be the resultant of the given below program?
#include<stdio.h> #include<stdarg.h> Void fun(char *msg, ...); int main () { fun("IndiaMAX", 1, 4, 7, 11, 0); return 0; } void fun(char *msg, ...) { va_list ptr;{ int num; va_start(ptr, msg); num = va_arg(ptr, int); num = va_arg(ptr, int); printf("%d", num); }
Answer : C
Explanation
va_list ptr is an argument pointer that traverse through the variable arguments passed in the function, where, va_start points 'ptr' to the first argument (IndiaMix). In each call to the va_arg, ptr shift to the next variable argument and after two calls ptr ends up at '7' and return the number '4'.
#include<stdio.h> #include<stdarg.h> Void fun(char *msg, ...); int main () { fun("IndiaMAX", 1, 4, 7, 11, 0); return 0; } void fun(char *msg, ...) { va_list ptr;{ int num; va_start(ptr, msg); num = va_arg(ptr, int); num = va_arg(ptr, int); printf("%d", num); }
Q 12 - The correct order of evaluation for the expression “z = x + y * z / 4 % 2 – 1”
Answer : D
Explanation
* / % holds highest priority than + - . All with left to right associativity.
Q 13 - In C, what is the correct hierarchy of arithmetic operations?
Answer : C
Explanation
In C, there are 5 arithmetic operators (+, -, *, /, and %) that can be used in performing arithmetic operations.
Q 14 - To print a double value which format specifier can be used?
Answer : B
Explanation
Double value can be printed using %lf format specifier.
Q 15 - Which files will get closed through the fclose() in the following program?
#include<stdio.h> int main () { FILE *fs, *ft, *fp; fp = fopen("ABC", "r"); fs = fopen("ACD", "r"); ft = fopen("ADF", "r"); fclose(fp, fs, ft); return 0; }
Answer : D
Explanation
The syntax of fclose() function is wrong; it should be int fclose(FILE *stream); closes the stream. Here, fclose(fp, fs, ft); is using separator(,) which returns error by saying that extra parameter in call to fclose() function.
#include<stdio.h> int main () { FILE *fs, *ft, *fp; fp = fopen("ABC", "r"); fs = fopen("ACD", "r"); ft = fopen("ADF", "r"); fclose(fp, fs, ft); return 0; }
Q 16 - Which of the following statement shows the correct implementation of nested conditional operation by finding greatest number out of three numbers?
Answer : A
Explanation
Syntax is exp1?exp2:exp3. Any ‘exp’ can be a valid expression.
Q 17 - Choose the correct order from given below options for the calling function of the code “a = f1(23, 14) * f2(12/4) + f3();”?
Answer : D
Explanation
Evaluation order is implementation dependent.
Q 18 - What will be the output of the following program?
#include<stdio.h> int main() { const int i = 0; printf("%d\n", i++); return 0; }
Answer : D
Explanation
It is because ++needs a value and a const variable can’t be modified.
#include<stdio.h> int main() { const int i = 0; printf("%d\n", i++); return 0; }
Q 19 - An operation with only one operand is called unary operation.
B - An operation with two operand is called unary operation
C - An operation with unlimited operand is called unary operation
Answer : A
Explanation
Unary operator acts on single expression.
Q 20 - Choose the correct order of evaluation,
A - Relational Arithmetic Logical Assignment
B - Arithmetic Relational Logical Assignment
Answer : B
Explanation
As per the operators preference.
Q 21 - Which printf() statement will you use to print out a (float value) and b (double value)?
Float a = 3.14; Double b = 3.14;
Answer : A
Explanation
%f can be use to print out float value and %lf can be use to print out double value.
Q 22 - To print a float value which format specifier can be used?
Answer : A
Explanation
%f can be use to print out float value and %lf can be use to print out double value.
Q 23 - Choose the correct unary operators in C – a) !, b) ~, c) ^&, d) ++
Answer : A
Explanation
In C, these are the unary operators,
Logical NOT = ! Address-of = & Cast Operator = ( ) Pointer dereference = * Unary Plus = + Increment = ++ Unary negation = –
Q 24 - What will be the output of the following program?
#include<stdio.h> int main() { const int x = 5; const int *ptrx; ptrx = &x; *ptrx = 10; printf("%d\n", x); return 0; }
Answer : D
Explanation
The above program will return error
#include<stdio.h> int main() { const int x = 5; const int *ptrx; ptrx = &x; *ptrx = 10; printf("%d\n", x); return 0; }
Q 25 - What do the following statement defines?
int *ptr[10];
A - ptr is a pointer to an array of 10 integer pointers.
B - ptr is a array of 10 pointers to integers
Answer : B
Explanation
square parenthesis signify as array at declaration and type is int*, so array of integer pointers.
Q 26 - What is the role of "r+" on the file "NOTES.TXT" in the given below code?
#include<stdio.h> int main () { FILE *fp; fp = fopen("NOTES.TXT", "r+"); return 0; }
A - "r+" open the file "NOTES.TXT" file for reading
B - "r+" open the file "NOTES.TXT" file for writing
C - "r+" open the file "NOTES.TXT" file for appending
D - "r+" open the file "NOTES.TXT" file for reading & writing both
Answer : D
Explanation
fopen() function is used to open a file and r++ enable the file for reading and writing.
#include<stdio.h> int main () { FILE *fp; fp = fopen("NOTES.TXT", "r+"); return 0; }
Q 27 - In the given below code, what will be return by the function get ()?
#include<stdio.h> int get(); int main() { const int x = get(); printf("%d", x); return 0; } int get() { return 40; }
Answer : A
Explanation
Firstly, “int get()” which is a get() function prototype returns an integer value without any parameters.
Secondly, const int x = get(); The constant variable x is declared as an integer data type and initialized with the value of get(). Hence, the value of get() is 40, printf("%d", x); will print the value of x, that means; 40. So, the program output will be 40.
#include<stdio.h> int get(); int main() { const int x = get(); printf("%d", x); return 0; } int get() { return 40; }
Q 28 - During preprocessing, the code “#include<stdio.h>” gets replaced by the contents of the file stdio.h.
B - During linking the code “#include<stdio.h>” replaces by stdio.h
C - During execution the code “#include<stdio.h>” replaces by stdio.h
D - During editing the code “#include<stdio.h>” replaces by stdio.h
Answer : A
Explanation
Preprocessing enlarges and boosts the C programming language by replacing preprocessing directive “#include<stdio.h>” with the content of the file stdio.h.
Q 29 - What value strcmp() function returns when two strings are the same?
Answer : A
Explanation
The C library function strcmp() compares two strings with each other and return the value accordingly.
int strcmp(const char *str1, const char *str2)
Comparison happens between first string (str1) with second string (str2).
By comparing two strings, the values return by the function strcmp() are,
- If, str1 is less than str2 then Return value < 0
- If, str2 is less than str1 then Return value > 0
- If, str1 is equal to str2 then Return value = 0
Q 30 - What will be the output of the given below program in TurboC
#include<stdio.h> int fun(int **ptr); int main() { int i = 10, j = 20; const int *ptr = &i; printf(" i = %5X", ptr); printf(" ptr = %d", *ptr); ptr = &j; printf(" j = %5X", ptr); printf(" ptr = %d", *ptr); return 0; }
A - i= FFE6 ptr=30 j=FFE4 ptr=36
B - i= FFE0 ptr=04 j=FFE1 ptr=30
Answer : C
Explanation
First the pointer holds I’s address and changes later to hold j’s address. Hence printing the 10 & 20 respectively.
#include<stdio.h> int fun(int **ptr); int main() { int i = 10, j = 20; const int *ptr = &i; printf(" i = %5X", ptr); printf(" ptr = %d", *ptr); ptr = &j; printf(" j = %5X", ptr); printf(" ptr = %d", *ptr); return 0; }
Q 31 - What will be the output of the given below code?
#include<stdio.h> int main() { const int *ptr = &i; char str[] = "Welcome"; s = str; while(*s) printf("%c", *s++); return 0; }
Answer : A
Explanation
Although, char str[] = "Welcome"; and s = str;, the program will print the value of s.
#include<stdio.h> int main() { const int *ptr = &i; char str[] = "Welcome"; s = str; while(*s) printf("%c", *s++); return 0; }
Q 32 - Which statement can print \n on the screen?
Answer : A
Explanation
Option A is the correct answer. In C programming language, "\n" is the escape sequence for printing a new line character. In printf("\\n"); statement, "\\" symbol will be printed as "\" and “n” will be known as a common symbol.
Q 33 - According to ANSI specification, how to declare main () function with command-line arguments?
A - int main(int argc, char *argv[])
B - int char main(int argc, *argv)
Answer : A
Explanation
Some time, it becomes necessary to deliver command line values to the C programming to execute the particular code when the code of the program is controlled from outside. Those command line values are called command line arguments. The command line arguments are handled by the main() function.
Declaration of main () with command-line argument is,
int main(int argc, char *argv[])
Where, argc refers to the number of arguments passed, and argv[] is a pointer array which points to each argument passed to the program.
Q 34 - In the given below code, what will be the value of a variable x?
#include<stdio.h> int main() { int y = 100; const int x = y; printf("%d\n", x); return 0; }
Answer : A
Explanation
Although, integer y = 100; and constant integer x is equal to y. here in the given above program we have to print the x value, so that it will be 100.
Q 35 - The library function strrchr() finds the first occurrence of a substring in another string.
Answer : B
Explanation
Strstr() finds the first occurrence of a substring in another string.
Q 36 - If, the given below code finds the length of the string then what will be the length?
#include<stdio.h> int xstrlen(char *s) { int length = 0; while(*s!='\0') {length++; s++;} return (length); } int main() { char d[] = "IndiaMAX"; printf("Length = %d\n", xstrlen(d)); return 0; }
Answer : B
Explanation
Here, *s is char pointer that holds character string. To print whole string we use printf("%s",s) by using base address. s contains base address (&s[0]) and printf will print characters until '\0' occurs. *s only gives first character of input string, but s++ will increase the base address by 1 byte. When *s=='\0' encountered, it will terminate loop.
#include<stdio.h> int xstrlen(char *s) { int length = 0; while(*s!='\0') {length++; s++;} return (length); } int main() { char d[] = "IndiaMAX"; printf("Length = %d\n", xstrlen(d)); return 0; }
Q 37 - The maximum combined length of the command-line arguments as well as the spaces between adjacent arguments is – a) 120 characters, b) 56 characters, c) Vary from one OS to another
Answer : D
Explanation
The maximum combined length of the command-line arguments and the spaces between adjacent arguments vary from one OS to another.
Q 38 - Choose the function that is most appropriate for reading in a multi-word string?
Answer : D
Explanation
gets (); = Collects a string of characters terminated by a new line from the standard input stream stdin
Q 39 - In the given below statement, what does the “arr” indicate?
char *arr[30];
A - arr is a array of function
B - arr is a array of 30 characters
Answer : D
Explanation
square parenthesis signify as array at declaration and type is char*, so array of character pointers.
Q 40 - In the given below statement, what does the “pf” indicate?
int (*pf)();
Answer : A
Explanation
pf is a pointer as well holds some functions reference.
Q 41 - extern int fun(); - The declaration indicates the presence of a global function defined outside the current module or in another file.
Answer : A
Explanation
Extern is used to resolve the scope of global identifier.
Q 42 - What is the output of the following program?
#include<stdio.h> main () { int i, j; for(i=5, j=1; i>j; i--, ++j) }
Answer : D
Explanation
5 1, 4 2- The condition fails when i=j=3.
#include<stdio.h> main () { int i, j; for(i=5, j=1; i>j; i--, ++j) }
Q 43 - What is the output of the following program?
#include<stdio.h> main () { int a=1, b=2, *p=&a, *q=&b, *r=p; p = q; q = r; printf("%d %d %d %d\n",a,b,*p,*q); }
Answer : A
Explanation
1 2 2 1, the pointers are swapped not the values.
#include<stdio.h> main () { int a=1, b=2, *p=&a, *q=&b, *r=p; p = q; q = r; printf("%d %d %d %d\n",a,b,*p,*q); }
Q 44 - What is the output of the following program?
#include<stdio.h> void g(void) { } main () { void (*f)(void); f = g; f(); }
B - Calling f(); is invalid it should be (*f)();
Answer : A
Explanation
Hello, every line is a valid statement.
#include<stdio.h> void g(void) { } main () { void (*f)(void); f = g; f(); }
Q 45 - What is the output of the following program?
#include<stdio.h> int f(int i) { } main () { printf("%d",f(f(f(f(f(1)))))); }
Answer : C
Explanation
1, the return value is post increment expression as it always receives 1 and returns 1.
#include<stdio.h> int f(int i) { } main () { printf("%d",f(f(f(f(f(1)))))); }
Q 46 - What is the output of the following program?
#include<stdio.h> main () { static int i = 1; if(i--) { printf("%d ",i); main(); } }
Answer : A
Explanation
0, static variable retains its value between the function calls.
#include<stdio.h> main () { static int i = 1; if(i--) { printf("%d ",i); main(); } }
Q 47 - What is the output of the following program?
#include<stdio.h> main () { printf(); }
A - Program compiles as printf is designed to receive variable number of arguments.
C - printf is not a built in library function
D - Semicolon need to be removed while calling printf with no parameters.
Answer : B
Explanation
(b), printfs need arguments.
Q 48 - Does the following program compiles?
#include “stdio.h”
A - It fails as there is no main() function
B - It fails as header file is enclosed in double quotes
C - It compiles and executes to produce no displayable output
Answer : D
Explanation
(d), It compiles successfully and can’t be executed. Use gcc –c option to compile the same with command line compiler (UNIX/Linux) or just compile without build in an IDE.
Q 49 - What is the output of the following program?
#include<stdio.h> main () { int *p = NULL; #undef NULL if(p==NULL) printf("NULL"); else printf("Nill"); }
Answer : C
Explanation
Compile error, as the macro is undefined NULL is considered as undeclared.
#include<stdio.h> main () { int *p = NULL; #undef NULL if(p==NULL) printf("NULL"); else printf("Nill"); }
Q 50 - What is the output of the following program?
main() { puts(__DATE__); }
C - Compile error, says __DATE__ in undeclared.
D - Compile error: Need to include ‘stdio.h’ as __DATE__ in defined in it.
Answer : B
Explanation
Prints date, __DATE__ is a compiler defined macro.
Answer Sheet
Question Number | Answer Key |
---|---|
1 | B |
2 | A |
3 | C |
4 | A |
5 | D |
6 | B |
7 | A |
8 | B |
9 | A |
10 | C |
11 | C |
12 | D |
13 | C |
14 | B |
15 | D |
16 | A |
17 | D |
18 | D |
19 | A |
20 | B |
21 | A |
22 | A |
23 | A |
24 | D |
25 | B |
26 | D |
27 | A |
28 | A |
29 | A |
30 | C |
31 | A |
32 | A |
33 | A |
34 | A |
35 | B |
36 | B |
37 | D |
38 | D |
39 | D |
40 | A |
41 | A |
42 | D |
43 | A |
44 | A |
45 | C |
46 | A |
47 | B |
48 | D |
49 | C |
50 | B |