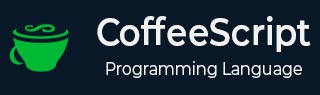
- CoffeeScript Tutorial
- CoffeeScript - Home
- CoffeeScript - Overview
- CoffeeScript - Environment
- CoffeeScript - command-line utility
- CoffeeScript - Syntax
- CoffeeScript - Data Types
- CoffeeScript - Variables
- CoffeeScript - Operators and Aliases
- CoffeeScript - Conditionals
- CoffeeScript - Loops
- CoffeeScript - Comprehensions
- CoffeeScript - Functions
- CoffeeScript Object Oriented
- CoffeeScript - Strings
- CoffeeScript - Arrays
- CoffeeScript - Objects
- CoffeeScript - Ranges
- CoffeeScript - Splat
- CoffeeScript - Date
- CoffeeScript - Math
- CoffeeScript - Exception Handling
- CoffeeScript - Regular Expressions
- CoffeeScript - Classes and Inheritance
- CoffeeScript Advanced
- CoffeeScript - Ajax
- CoffeeScript - jQuery
- CoffeeScript - MongoDB
- CoffeeScript - SQLite
- CoffeeScript Useful Resources
- CoffeeScript - Quick Guide
- CoffeeScript - Useful Resources
- CoffeeScript - Discussion
CoffeeScript - Strings
The String object lets you work with a series of characters. As in most of the programming languages, the Strings in CoffeeScript are declared using quotes as −
my_string = "Hello how are you" console.log my_string
On compiling, it will generate the following JavaScript code.
// Generated by CoffeeScript 1.10.0 (function() { var my_string; my_string = "Hello how are you"; console.log(my_string); }).call(this);
String Concatenation
We can concatenate two strings using the "+" symbol as shown below.
new_string = "Hello how are you "+"Welcome to Tutorialspoint" console.log new_String
On compiling, it will generate the following JavaScript code.
// Generated by CoffeeScript 1.10.0 (function() { var new_string; new_string = "Hello how are you " + "Welcome to Tutorialspoint"; console.log(new_String); }).call(this);
If you execute the above example, you can observe the concatenated String as shown below.
Hello how are you Welcome to Tutorialspoint
String Interpolation
CoffeeScript also provides a feature known as String interpolation to include variables in stings. This feature of CoffeeScript was inspired from Ruby language.
String interpolation was done using the double quotes "", a hash tag # and a pair of curly braces { }. The String is declared in double quotes and the variable that is to be interpolated is wrapped within the curly braces which are prefixed by a hash tag as shown below.
name = "Raju" age = 26 message ="Hello #{name} your age is #{age}" console.log message
On compiling the above example, it generates the following JavaScript. Here you can observe the String interpolation is converted into normal concatenation using the + symbol.
// Generated by CoffeeScript 1.10.0 (function() { var age, message, name; name = "Raju"; age = 26; message = "Hello " + name + " your age is " + age; console.log(message); }).call(this);
If you execute the above CoffeeScript code, it gives you the following output.
Hello Raju your age is 26
The variable that is passed as #{variable} is interpolated only if the string is enclosed between double quotes " ". Using single quotes ' ' instead of double quotes produces the line as it is without interpolation. Consider the following example.
name = "Raju" age = 26 message ='Hello #{name} your age is #{age}' console.log message
If we use single quotes instead of double quotes in interpolation, you will get the following output.
Hello #{name} your age is #{age}
CoffeeScript allows multiple lines in Strings without concatenating them as shown below.
my_string = "hello how are you Welcome to tutorialspoint Have a nice day." console.log my_string
It generates the following output.
hello how are you Welcome to tutorialspoint Have a nice day.
JavaScript String Object
The String object of JavaScript lets you work with a series of characters. This object provides you a lot of methods to perform various operations on Stings.
Since we can use JavaScript libraries in our CoffeeScript code, we can use all those methods in our CoffeeScript programs.
String Methods
Following is the list of methods of the String object of JavaScript. Click on the name of these methods to get an example demonstrating their usage in CoffeeScript.
S.No. | Method & Description |
---|---|
1 | charAt()
Returns the character at the specified index. |
2 | charCodeAt()
Returns a number indicating the Unicode value of the character at the given index. |
3 | concat()
Combines the text of two strings and returns a new string. |
4 | indexOf()
Returns the index within the calling String object of the first occurrence of the specified value, or -1 if not found. |
5 | lastIndexOf()
Returns the index within the calling String object of the last occurrence of the specified value, or -1 if not found. |
6 | localeCompare()
Returns a number indicating whether a reference string comes before or after or is the same as the given string in sort order. |
7 | match()
Used to match a regular expression against a string. |
8 | search()
Executes the search for a match between a regular expression and a specified string. |
9 | slice()
Extracts a section of a string and returns a new string. |
10 | split()
Splits a String object into an array of strings by separating the string into substrings. |
11 | substr()
Returns the characters in a string beginning at the specified location through the specified number of characters. |
12 | toLocaleLowerCase()
The characters within a string are converted to lower case while respecting the current locale. |
13 | toLocaleUpperCase()
The characters within a string are converted to upper case while respecting the current locale. |
14 | toLowerCase()
Returns the calling string value converted to lower case. |
15 | toUpperCase()
Returns the calling string value converted to uppercase. |