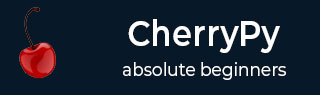
- CherryPy Tutorial
- CherryPy - Home
- CherryPy - Introduction
- CherryPy - Environment Setup
- CherryPy - Vocabulary
- Bulit-in Http Server
- CherryPy - ToolBox
- CherryPy - A Working Application
- CherryPy - Web Services
- CherryPy - Presentation Layer
- CherryPy - Use Of Ajax
- CherryPy - Demo Application
- CherryPy - Testing
- Deployment Of Application
- CherryPy Useful Resources
- CherryPy - Quick Guide
- CherryPy - Useful Resources
- CherryPy - Discussion
CherryPy - ToolBox
Within CherryPy, built-in tools offer a single interface to call the CherryPy library. The tools defined in CherryPy can be implemented in the following ways −
- From the configuration settings
- As a Python decorator or via the special _cp_config attribute of a page handler
- As a Python callable that can be applied from within any function
Basic Authentication Tool
The purpose of this tool is to provide basic authentication to the application designed in the application.
Arguments
This tool uses the following arguments −
Name | Default | Description |
---|---|---|
realm | N/A | String defining the realm value. |
users | N/A | Dictionary of the form − username:password or a Python callable function returning such a dictionary. |
encrypt | None | Python callable used to encrypt the password returned by the client and compare it with the encrypted password provided in the users dictionary. |
Example
Let us take an example to understand how it works −
import sha import cherrypy class Root: @cherrypy.expose def index(self): return """ <html> <head></head> <body> <a href = "admin">Admin </a> </body> </html> """ class Admin: @cherrypy.expose def index(self): return "This is a private area" if __name__ == '__main__': def get_users(): # 'test': 'test' return {'test': 'b110ba61c4c0873d3101e10871082fbbfd3'} def encrypt_pwd(token): return sha.new(token).hexdigest() conf = {'/admin': {'tools.basic_auth.on': True, tools.basic_auth.realm': 'Website name', 'tools.basic_auth.users': get_users, 'tools.basic_auth.encrypt': encrypt_pwd}} root = Root() root.admin = Admin() cherrypy.quickstart(root, '/', config=conf)
The get_users function returns a hard-coded dictionary but also fetches the values from a database or anywhere else. The class admin includes this function which makes use of an authentication built-in tool of CherryPy. The authentication encrypts the password and the user Id.
The basic authentication tool is not really secure, as the password can be encoded and decoded by an intruder.
Caching Tool
The purpose of this tool is to provide memory caching of CherryPy generated content.
Arguments
This tool uses the following arguments −
Name | Default | Description |
---|---|---|
invalid_methods | ("POST", "PUT", "DELETE") | Tuples of strings of HTTP methods not to be cached. These methods will also invalidate (delete) any cached copy of the resource. |
cache_Class | MemoryCache | Class object to be used for caching |
Decoding Tool
The purpose of this tool is to decode the incoming request parameters.
Arguments
This tool uses the following arguments −
Name | Default | Description |
---|---|---|
encoding | None | It looks for the content-type header |
Default_encoding | "UTF-8" | Default encoding to be used when none is provided or found. |
Example
Let us take an example to understand how it works −
import cherrypy from cherrypy import tools class Root: @cherrypy.expose def index(self): return """ <html> <head></head> <body> <form action = "hello.html" method = "post"> <input type = "text" name = "name" value = "" /> <input type = ”submit” name = "submit"/> </form> </body> </html> """ @cherrypy.expose @tools.decode(encoding='ISO-88510-1') def hello(self, name): return "Hello %s" % (name, ) if __name__ == '__main__': cherrypy.quickstart(Root(), '/')
The above code takes a string from the user and it will redirect the user to "hello.html" page where it will be displayed as “Hello” with the given name.
The output of the above code is as follows −
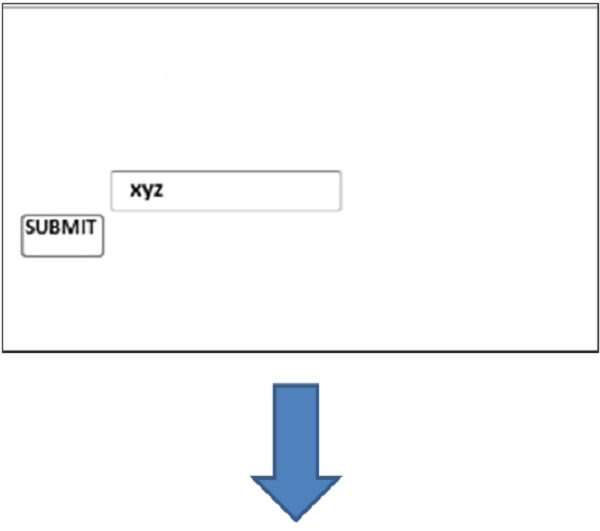
hello.html
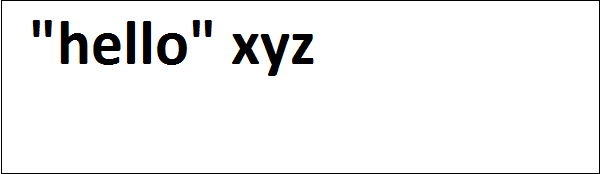
To Continue Learning Please Login