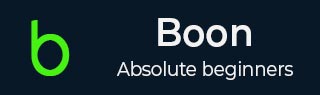
- Boon Tutorial
- Boon - Home
- Boon - Overview
- Boon - Environment Setup
- Parsing JSON
- Boon - To Object
- Boon - To Map
- Boon - Sources
- Generating JSON
- Boon - From Object
- Boon - From Map
- Date Handling
- Boon - Long To Date
- Boon - String To Date
- Boon - Generating Date
- Annotations
- Boon - @JsonIgnore
- Boon - @JsonInclude
- Boon - @JsonViews
- Boon - @JsonProperty
- Boon Useful Resources
- Boon - Quick Guide
- Boon - Useful Resources
- Boon - Discussion
Boon - To Object
ObjectMapper is the main actor class of Boon library. ObjectMapper class provides functionality for reading and writing JSON, either to and from basic POJOs (Plain Old Java Objects), or to and from a general-purpose JSON Tree Model (JsonNode), as well as related functionality for performing conversions.
It is also highly customizable to work both with different styles of JSON content, and to support more advanced Object concepts such as polymorphism and Object identity.
Example
Following example is using ObjectMapper class to parse a JSON string to a Student Object.
import org.boon.json.JsonFactory; import org.boon.json.ObjectMapper; public class BoonTester { public static void main(String args[]){ ObjectMapper mapper = JsonFactory.create(); String jsonString = "{\"name\":\"Mahesh\", \"age\":21}"; Student student = mapper.readValue(jsonString, Student.class); System.out.println(student); } } class Student { private String name; private int age; public Student(){} public String getName() { return name; } public void setName(String name) { this.name = name; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String toString(){ return "Student [ name: "+name+", age: "+ age+ " ]"; } }
Output
The output is mentioned below −
Student [ name: Mahesh, age: 21 ]
Advertisements