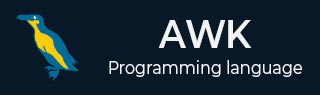
- AWK Tutorial
- AWK - Home
- AWK - Overview
- AWK - Environment
- AWK - Workflow
- AWK - Basic Syntax
- AWK - Basic Examples
- AWK - Built in Variables
- AWK - Operators
- AWK - Regular Expressions
- AWK - Arrays
- AWK - Control Flow
- AWK - Loops
- AWK - Built in Functions
- AWK - User Defined Functions
- AWK - Output Redirection
- AWK - Pretty Printing
- AWK Useful Resources
- AWK - Quick Guide
- AWK - Useful Resources
- AWK - Discussion
AWK - Pretty Printing
So far we have used AWK's print and printf functions to display data on standard output. But printf is much more powerful than what we have seen before. This function is borrowed from the C language and is very helpful while producing formatted output. Below is the syntax of the printf statement −
Syntax
printf fmt, expr-list
In the above syntax fmt is a string of format specifications and constants. expr-list is a list of arguments corresponding to format specifiers.
Escape Sequences
Similar to any string, format can contain embedded escape sequences. Discussed below are the escape sequences supported by AWK −
New Line
The following example prints Hello and World in separate lines using newline character −
Example
[jerry]$ awk 'BEGIN { printf "Hello\nWorld\n" }'
On executing this code, you get the following result −
Output
Hello World
Horizontal Tab
The following example uses horizontal tab to display different field −
Example
[jerry]$ awk 'BEGIN { printf "Sr No\tName\tSub\tMarks\n" }'
On executing the above code, you get the following result −
Output
Sr No Name Sub Marks
Vertical Tab
The following example uses vertical tab after each filed −
Example
[jerry]$ awk 'BEGIN { printf "Sr No\vName\vSub\vMarks\n" }'
On executing this code, you get the following result −
Output
Sr No Name Sub Marks
Backspace
The following example prints a backspace after every field except the last one. It erases the last number from the first three fields. For instance, Field 1 is displayed as Field, because the last character is erased with backspace. However, the last field Field 4 is displayed as it is, as we did not have a \b after Field 4.
Example
[jerry]$ awk 'BEGIN { printf "Field 1\bField 2\bField 3\bField 4\n" }'
On executing this code, you get the following result −
Output
Field Field Field Field 4
Carriage Return
In the following example, after printing every field, we do a Carriage Return and print the next value on top of the current printed value. It means, in the final output, you can see only Field 4, as it was the last thing to be printed on top of all the previous fields.
Example
[jerry]$ awk 'BEGIN { printf "Field 1\rField 2\rField 3\rField 4\n" }'
On executing this code, you get the following result −
Output
Field 4
Form Feed
The following example uses form feed after printing each field.
Example
[jerry]$ awk 'BEGIN { printf "Sr No\fName\fSub\fMarks\n" }'
On executing this code, you get the following result −
Output
Sr No Name Sub Marks
Format Specifier
As in C-language, AWK also has format specifiers. The AWK version of the printf statement accepts the following conversion specification formats −
%c
It prints a single character. If the argument used for %c is numeric, it is treated as a character and printed. Otherwise, the argument is assumed to be a string, and the only first character of that string is printed.
Example
[jerry]$ awk 'BEGIN { printf "ASCII value 65 = character %c\n", 65 }'
Output
On executing this code, you get the following result −
ASCII value 65 = character A
%d and %i
It prints only the integer part of a decimal number.
Example
[jerry]$ awk 'BEGIN { printf "Percentags = %d\n", 80.66 }'
On executing this code, you get the following result −
Output
Percentags = 80
%e and %E
It prints a floating point number of the form [-]d.dddddde[+-]dd.
Example
[jerry]$ awk 'BEGIN { printf "Percentags = %E\n", 80.66 }'
On executing this code, you get the following result −
Output
Percentags = 8.066000e+01
The %E format uses E instead of e.
Example
[jerry]$ awk 'BEGIN { printf "Percentags = %e\n", 80.66 }'
On executing this code, you get the following result −
Output
Percentags = 8.066000E+01
%f
It prints a floating point number of the form [-]ddd.dddddd.
Example
[jerry]$ awk 'BEGIN { printf "Percentags = %f\n", 80.66 }'
On executing this code, you get the following result −
Output
Percentags = 80.660000
%g and %G
Uses %e or %f conversion, whichever is shorter, with non-significant zeros suppressed.
Example
[jerry]$ awk 'BEGIN { printf "Percentags = %g\n", 80.66 }'
Output
On executing this code, you get the following result −
Percentags = 80.66
The %G format uses %E instead of %e.
Example
[jerry]$ awk 'BEGIN { printf "Percentags = %G\n", 80.66 }'
On executing this code, you get the following result −
Output
Percentags = 80.66
%o
It prints an unsigned octal number.
Example
[jerry]$ awk 'BEGIN { printf "Octal representation of decimal number 10 = %o\n", 10}'
On executing this code, you get the following result −
Output
Octal representation of decimal number 10 = 12
%u
It prints an unsigned decimal number.
Example
[jerry]$ awk 'BEGIN { printf "Unsigned 10 = %u\n", 10 }'
On executing this code, you get the following result −
Output
Unsigned 10 = 10
%s
It prints a character string.
Example
[jerry]$ awk 'BEGIN { printf "Name = %s\n", "Sherlock Holmes" }'
On executing this code, you get the following result −
Output
Name = Sherlock Holmes
%x and %X
It prints an unsigned hexadecimal number. The %X format uses uppercase letters instead of lowercase.
Example
[jerry]$ awk 'BEGIN { printf "Hexadecimal representation of decimal number 15 = %x\n", 15 }'
On executing this code, you get the following result −
Output
Hexadecimal representation of decimal number 15 = f
Now let use %X and observe the result −
Example
[jerry]$ awk 'BEGIN { printf "Hexadecimal representation of decimal number 15 = %X\n", 15 }'
On executing this code, you get the following result −
Output
Hexadecimal representation of decimal number 15 = F
%%
It prints a single % character and no argument is converted.
Example
[jerry]$ awk 'BEGIN { printf "Percentags = %d%%\n", 80.66 }'
On executing this code, you get the following result −
Output
Percentags = 80%
Optional Parameters with %
With % we can use following optional parameters −
Width
The field is padded to the width. By default, the field is padded with spaces but when 0 flag is used, it is padded with zeroes.
Example
[jerry]$ awk 'BEGIN { num1 = 10; num2 = 20; printf "Num1 = %10d\nNum2 = %10d\n", num1, num2 }'
On executing this code, you get the following result −
Output
Num1 = 10 Num2 = 20
Leading Zeros
A leading zero acts as a flag, which indicates that the output should be padded with zeroes instead of spaces. Please note that this flag only has an effect when the field is wider than the value to be printed. The following example describes this −
Example
[jerry]$ awk 'BEGIN { num1 = -10; num2 = 20; printf "Num1 = %05d\nNum2 = %05d\n", num1, num2 }'
On executing this code, you get the following result −
Output
Num1 = -0010 Num2 = 00020
Left Justification
The expression should be left-justified within its field. When the input-string is less than the number of characters specified, and you want it to be left justified, i.e., by adding spaces to the right, use a minus symbol (–) immediately after the % and before the number.
In the following example, output of the AWK command is piped to the cat command to display the END OF LINE($) character.
Example
[jerry]$ awk 'BEGIN { num = 10; printf "Num = %-5d\n", num }' | cat -vte
On executing this code, you get the following result −
Output
Num = 10 $
Prefix Sign
It always prefixes numeric values with a sign, even if the value is positive.
Example
[jerry]$ awk 'BEGIN { num1 = -10; num2 = 20; printf "Num1 = %+d\nNum2 = %+d\n", num1, num2 }'
On executing this code, you get the following result −
Output
Num1 = -10 Num2 = +20
Hash
For %o, it supplies a leading zero. For %x and %X, it supplies a leading 0x or 0X respectively, only if the result is non-zero. For %e, %E, %f, and %F, the result always contains a decimal point. For %g and %G, trailing zeros are not removed from the result. The following example describes this −
Example
[jerry]$ awk 'BEGIN { printf "Octal representation = %#o\nHexadecimal representaion = %#X\n", 10, 10 }'
On executing this code, you get the following result −
Output
Octal representation = 012 Hexadecimal representation = 0XA