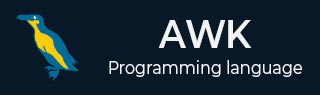
- AWK Tutorial
- AWK - Home
- AWK - Overview
- AWK - Environment
- AWK - Workflow
- AWK - Basic Syntax
- AWK - Basic Examples
- AWK - Built in Variables
- AWK - Operators
- AWK - Regular Expressions
- AWK - Arrays
- AWK - Control Flow
- AWK - Loops
- AWK - Built in Functions
- AWK - User Defined Functions
- AWK - Output Redirection
- AWK - Pretty Printing
- AWK Useful Resources
- AWK - Quick Guide
- AWK - Useful Resources
- AWK - Discussion
AWK - Arrays
AWK has associative arrays and one of the best thing about it is – the indexes need not to be continuous set of number; you can use either string or number as an array index. Also, there is no need to declare the size of an array in advance – arrays can expand/shrink at runtime.
Its syntax is as follows −
Syntax
array_name[index] = value
Where array_name is the name of array, index is the array index, and value is any value assigning to the element of the array.
Creating Array
To gain more insight on array, let us create and access the elements of an array.
Example
[jerry]$ awk 'BEGIN { fruits["mango"] = "yellow"; fruits["orange"] = "orange" print fruits["orange"] "\n" fruits["mango"] }'
On executing this code, you get the following result −
Output
orange yellow
In the above example, we declare the array as fruits whose index is fruit name and the value is the color of the fruit. To access array elements, we use array_name[index] format.
Deleting Array Elements
For insertion, we used assignment operator. Similarly, we can use delete statement to remove an element from the array. The syntax of delete statement is as follows −
Syntax
delete array_name[index]
The following example deletes the element orange. Hence the command does not show any output.
Example
[jerry]$ awk 'BEGIN { fruits["mango"] = "yellow"; fruits["orange"] = "orange"; delete fruits["orange"]; print fruits["orange"] }'
Multi-Dimensional arrays
AWK only supports one-dimensional arrays. But you can easily simulate a multi-dimensional array using the one-dimensional array itself.
For instance, given below is a 3x3 two-dimensional array −
100 200 300 400 500 600 700 800 900
In the above example, array[0][0] stores 100, array[0][1] stores 200, and so on. To store 100 at array location [0][0], we can use the following syntax −
Syntax
array["0,0"] = 100
Though we gave 0,0 as index, these are not two indexes. In reality, it is just one index with the string 0,0.
The following example simulates a 2-D array −
Example
[jerry]$ awk 'BEGIN { array["0,0"] = 100; array["0,1"] = 200; array["0,2"] = 300; array["1,0"] = 400; array["1,1"] = 500; array["1,2"] = 600; # print array elements print "array[0,0] = " array["0,0"]; print "array[0,1] = " array["0,1"]; print "array[0,2] = " array["0,2"]; print "array[1,0] = " array["1,0"]; print "array[1,1] = " array["1,1"]; print "array[1,2] = " array["1,2"]; }'
On executing this code, you get the following result −
Output
array[0,0] = 100 array[0,1] = 200 array[0,2] = 300 array[1,0] = 400 array[1,1] = 500 array[1,2] = 600
You can also perform a variety of operations on an array such as sorting its elements/indexes. For that purpose, you can use assort and asorti functions