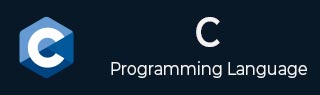
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
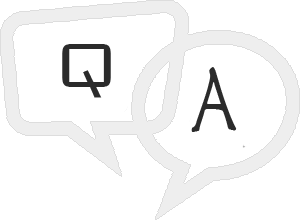
Q 1 - What is the output of the below code snippet?
#include<stdio.h> main() { for()printf("Hello"); }
Answer : D
Explanation
Compiler error, semi colons need to appear though the expressions are optional for the ‘for’ loop.
Q 2 - What is the output of the following program?
#include<stdio.h> main() { float t = 2; switch(t) { case 2: printf("Hi"); default: printf("Hello"); } }
Answer : D
Explanation
Error, switch expression can’t be float value.
Q 3 - Linker generates ___ file.
Answer : B
Explanation
(b). Linker links the object code of your program and library code to produce executable.
Q 4 - Choose the invalid predefined macro as per ANSI C.
Answer : D
Explanation
There is no macro define with the name __C++__, but __cplusplus is defined by ANSI)
Q 5 - What is the output of the following program?
#include<stdio.h> main() { #undef NULL char *s = "Hello"; while(*s != NULL) { printf("%c", *s++); } }
B - Compile error: there is no macro called “undef”
Answer : D
Explanation
NULL is equivalent to ‘\0’ in value. Statement *s++ prints the character first and increments the address later.
Q 6 - Choose the correct program that round off x value (a float value) to an int value to return the output value 4,
A - float x = 3.6;
int y = (int)(x + 0.5); printf ("Result = %d\n", y );
B - float x = 3.6;
int y = int(x + 0.5); printf ("Result = %d\n", y );
C - float x = 3.6;
int y = (int)x + 0.5 printf ("Result = %d\n", y );
D - float x = 3.6;
int y = (int)((int)x + 0.5) printf ("Result = %d\n", y );
Answer : A
Explanation
#include <math.h> #include <stdio.h> int main() { float x = 3.6; int y = (int)(x + 0.5); printf ("Result = %d\n", y ); return 0; }
Q 7 - The given below program allocates the memory, what function will you use to free the allocated memory?
#include<stdio.h> #include<stdlib.h> #define MAXROW 4 # define MAXCOL 5 int main () { int **p, i, j p = (int **) malloc(MAXROW * sizeof(int*)); return 0; }
Answer : B
Explanation
free() is the function in C language to release the allocated memory by any dynamic memory allocating built in library function.
#include<stdio.h> #include<stdlib.h> #define MAXROW 4 # define MAXCOL 5 int main () { int **p, i, j p = (int **) malloc(MAXROW * sizeof(int*)); return 0; }
Q 8 - The return keyword used to transfer control from a function back to the calling function.
Answer : A
Explanation
In C, the return function stops the execution of a function and returns control with value to the calling function. Execution begins in the calling function by instantly following the call.
Q 9 - Which files will get closed through the fclose() in the following program?
#include<stdio.h> int main () { FILE *fs, *ft, *fp; fp = fopen("ABC", "r"); fs = fopen("ACD", "r"); ft = fopen("ADF", "r"); fclose(fp, fs, ft); return 0; }
Answer : D
Explanation
The syntax of fclose() function is wrong; it should be int fclose(FILE *stream); closes the stream. Here, fclose(fp, fs, ft); is using separator(,) which returns error by saying that extra parameter in call to fclose() function.
#include<stdio.h> int main () { FILE *fs, *ft, *fp; fp = fopen("ABC", "r"); fs = fopen("ACD", "r"); ft = fopen("ADF", "r"); fclose(fp, fs, ft); return 0; }
Q 10 - If, the given below code finds the length of the string then what will be the length?
#include<stdio.h> int xstrlen(char *s) { int length = 0; while(*s!='\0') {length++; s++;} return (length); } int main() { char d[] = "IndiaMAX"; printf("Length = %d\n", xstrlen(d)); return 0; }
Answer : B
Explanation
Here, *s is char pointer that holds character string. To print whole string we use printf("%s",s) by using base address. s contains base address (&s[0]) and printf will print characters until '\0' occurs. *s only gives first character of input string, but s++ will increase the base address by 1 byte. When *s=='\0' encountered, it will terminate loop.
#include<stdio.h> int xstrlen(char *s) { int length = 0; while(*s!='\0') {length++; s++;} return (length); } int main() { char d[] = "IndiaMAX"; printf("Length = %d\n", xstrlen(d)); return 0; }
To Continue Learning Please Login