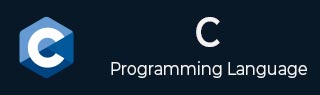
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Character Pointers and Functions in C
There is no string data type in C. An array of "char" type is considered as a string in C. Hence, a pointer of a char type array represents a string. This char pointer can then be passed as an argument to a function for processing the string.
The library functions in the header file "string.h" processes the string with char pointer parameters.
A string is declared as an array as follows −
char arr[] = "Hello";
The string is a NULL terminated array of characters. The last element in the above array is a NULL character (\0).
Declare a pointer of char type and assign it the address of the character at the 0th position −
char *ptr = &arr[0];
Remember that the name of the array itself is the address of 0th element.
char *ptr = arr;
A string may be declared using a pointer instead of an array variable (no square brackets).
char *ptr = "Hello";
This causes the string to be stored in the memory, and its address stored in ptr. We can traverse the string by incrementing the ptr.
while(*ptr != '\0'){ printf("%c", *ptr); ptr++; }
Example 1
Here is the full program code −
#include <stdio.h> int main(){ char arr[] = "Character Pointers and Functions in C"; char *ptr = arr; while(*ptr != '\0'){ printf("%c", *ptr); ptr++; } }
Output
Run the code and check its output −
Character Pointers and Functions in C
Example 2
Alternatively, pass ptr to printf() with %s format to print the string.
#include <stdio.h> int main(){ char arr[] = "Character Pointers and Functions in C"; char *ptr = arr; printf("%s", ptr); }
Output
On running this code, you will get the same output −
Character Pointers and Functions in C
The "string.h" header files defines a number of library functions that perform string processing such as finding the length of a string, copying a string and comparing two strings. These functions use char pointer arguments.
The strlen() Function
The strlen() function returns the length, i.e. the number of characters in a string. The prototype of strlen() function is as follows −
int strlen(char *)
Example 1
The following code shows how you can print the length of a string −
#include <stdio.h> #include <string.h> int main(){ char *ptr = "Hello"; printf("Given string: %s \n", ptr); printf("Length of the string: %d", strlen(ptr)); return 0; }
Output
When you run this code, it will produce the following output −
Given string: Hello Length of the string: 5
Example 2
Effectively, the strlen() function computes the string length as per the user-defined function str_len() as shown below −
#include <stdio.h> #include <string.h> int str_len(char *); int main(){ char *ptr = "Welcome to Tutorialspoint"; int length = str_len(ptr); printf("Given string: %s \n", ptr); printf("Length of the string: %d", length); return 0; } int str_len(char *ptr){ int i = 0; while(*ptr != '\0'){ i++; ptr++; } return i; }
Output
When you run this code, it will produce the following output −
Given string: Welcome to Tutorialspoint Length of the string: 25
The strcpy() Function
The assignment operator ( = ) is not used to assign a string value to a string variable, i.e., a char pointer. Instead, we need to use the strcpy() function with the following prototype −
char * strcpy(char * dest, char * source);
Example 1
The following example shows how you can use the strcpy() function −
#include <stdio.h> #include <string.h> int main(){ char *ptr = "How are you doing?"; char *ptr1; strcpy(ptr1, ptr); printf("%s", ptr1); return 0; }
Output
The strcpy() function returns the pointer to the destination string ptr1.
How are you doing?
Example 2
Internally, the strcpy() function implements the following logic in the user-defined str_cpy() function −
#include <stdio.h> #include <string.h> void str_cpy(char *d, char *s); int main(){ char *ptr = "Using the strcpy() Function"; char *ptr1; str_cpy(ptr1, ptr); printf("%s", ptr1); return 0; } void str_cpy(char *d, char *s){ int i; for(i = 0; s[i] != '\0'; i++) d[i] = s[i]; d[i] = '\0'; }
Output
When you runt his code, it will produce the following output −
Using the strcpy() Function
The function copies each character from the source string to the destination till the NULL character "\0" is reached. After the loop, it adds a "\0" character at the end of the destination array.
The strcmp() Function
The usual comparison operators (<, >, <=, >=, ==, and !=) are not allowed to be used for comparing two strings. Instead, we need to use strcmp() function from the "string.h" header file. The prototype of this function is as follows −
int strcmp(char *str1, char *str2)
The strcmp() function has three possible return values −
- When both strings are found to be identical, it returns "0".
- When the first not-matching character in str1 has a greater ASCII value than the corresponding character in str2, the function returns a positive integer. It implies that str1 appears after str2 in alphabetical order, as in a dictionary.
- When the first not-matching character in str1 has a lesser ASCII value than the corresponding character in str2, the function returns a negative integer. It implies that str1 appears before str2 in alphabetical order, as in a dictionary.
Example 1
The following example demonstrates how you can use the strcmp() function in a C program −
#include <stdio.h> #include <string.h> int main(){ char *s1 = "BASK"; char *s2 = "BALL"; int ret = strcmp(s1, s2); if (ret == 0) printf("Both strings are identical\n"); else if (ret > 0) printf("The first string appears after the second string \n"); else printf("The first string appears before the second string \n"); return 0; }
Output
Run the code and check its output −
The first string appears after the second string
Change s1 to BACK and run the code again. Now, you will get the following output −
The first string appears before the second string
Example 2
You can obtain a similar result using the user-defined function str_cmp(), as shown in the following code −
#include <stdio.h> #include <string.h> int str_cmp(char *str1, char *str2); int main(){ char *s1 = "The Best C Programming Tutorial Available Online"; char *s2 = "The Best C Programming Tutorial Available Online"; int ret = str_cmp(s1, s2); if (ret == 0) printf("Both strings are identical\n"); else if (ret > 0) printf("The first string appears after the second string\n"); else printf("The first string appears before the second string\n"); return 0; } int str_cmp(char *str1, char *str2) { while (*str1 != '\0' && *str2 != '\0') { if (*str1 != *str2) { return *str1 - *str2; } str1++; str2++; } // If both strings are equal, return 0 return 0; }
Output
When you run this code, it will produce the following output −
Both strings are identical
The str_cmp() function compares the characters at the same index in a string till the characters in either string are exhausted or the characters are equal.
At the time of detecting unequal characters at the same index, the difference in their ASCII values is returned. It returns "0" when the loop is terminated.
To Continue Learning Please Login