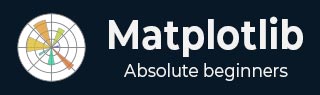
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Subplot Titles
What is Subplot Title?
Subplot titles in Matplotlib refer to the individual titles assigned to each subplot within a larger figure. When we have multiple subplots arranged in a grid such as a matrix of plots it's often beneficial to add titles to each subplot to provide context or describe the content of that specific subplot.
Setting subplot titles is a useful practice when creating multiple visualizations within a single figure by enhancing the readability and comprehension of our overall plots. We have the method namely set_title() for setting the title of the subplot.
By utilizing set_title() we can add descriptive titles to individual subplots within a figure allowing for better organization and comprehension of complex visualizations.
Purpose of Subplot title
Provide Context − Subplot titles offer descriptive information about the content of each subplot within a larger figure aiding in better understanding the visualizations.
Differentiate Subplots − Titles help distinguish between multiple plots by allowing viewers to identify and interpret each subplot's data or purpose easily.
Importance of Subplot title
Subplot titles help clarify the content or purpose of individual plots within a grid of subplots, especially when presenting multiple visualizations together.
They aid in quickly identifying the information presented in each subplot, improving the overall interpretability of the visualizations.
Syntax
The below is the syntax and parameters for setting up the subplot title.
ax.set_title('Title')
Where,
ax − It represents the axes object of the subplot for which the title is being set.
set_title() − The method used to set the title.
'Title' − It is the string representing the text of the title.
Subplots with title
In this example we are creating the subplots and setting up the title for each subplot by using the set_title() method available in the Matplotlib library.
Example
import matplotlib.pyplot as plt import numpy as np # Generating sample data x = np.linspace(0, 10, 100) y1 = np.sin(x) y2 = np.cos(x) # Creating subplots fig, (ax1, ax2) = plt.subplots(1, 2) # Plotting on the first subplot ax1.plot(x, y1) ax1.set_title('Sine Wave') # Plotting on the second subplot ax2.plot(x, y2) ax2.set_title('Cosine Wave') # Displaying the subplots plt.show()
Output

Example
Here in this example we are creating the subplots and adding the title to each subplot.
import matplotlib.pyplot as plt import numpy as np # Generating sample data x = np.linspace(0, 10, 50) y = np.sin(x) # Generating random data for scatter plot np.random.seed(0) x_scatter = np.random.rand(50) * 10 y_scatter = np.random.rand(50) * 2 - 1 # Random values between -1 and 1 # Creating subplots fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 4)) # 1 row, 2 columns # Line plot on the first subplot ax1.plot(x, y, color='blue', label='Line Plot') ax1.set_title('Line Plot') ax1.set_xlabel('X-axis') ax1.set_ylabel('Y-axis') ax1.legend() # Scatter plot on the second subplot ax2.scatter(x_scatter, y_scatter, color='red', label='Scatter Plot') ax2.set_title('Scatter Plot') ax2.set_xlabel('X-axis') ax2.set_ylabel('Y-axis') ax2.legend() # Displaying the subplots plt.show()
Output

To Continue Learning Please Login