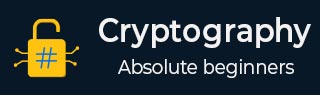
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - ROT13 Algorithm
Till now, you have learnt about reverse cipher and Caesar cipher algorithms. Now, let us discuss the ROT13 algorithm and its implementation.
So we are going to discuss the ROT13 cryptography algorithm. Rotate 13 Places is referred to as ROT13. It is a special case of the Caesar cipher encryption algorithm. It is among the simplest methods of encryption. As the algorithm is the same for both encryption and decryption, it is a symmetric key encryption technique.
Because the encryption key displays the letters A through Z as the numbers 0 through 25, the cipher text differs by 13 spaces from the plaintext letter. As an example, A turns into N, B into O, and so on. Because the encryption and decryption processes are identical, they can be done programatically.
Explanation of ROT13 Algorithm
ROT13 cipher refers to the abbreviated form Rotate by 13 places. In this particular Caesar Cipher, shift is always 13. The message is encrypted or decrypted by shifting each letter thirteen places.
Let us see the below image to know how ROT13 algorithm works −

Every letter has been moved 13 spaces to the right, as you can see. As an example, "B" will become "O," "D" will become "Q," and so on.
Features of ROT13
Here are some main features of ROT13 −
Since ROT13 is symmetric, both encryption and decryption use the same algorithm and key.
It works for each of the alphabetic letters, substituting each one with the letter 13 positions forward (or back, based on the decryption) in the alphabet.
The rotation value of 13 is fixed in ROT13.
When applying ROT13, if the rotation goes beyond 'Z', it comes back to the beginning of the alphabet 'A'.
ROT13 is very easy to implement and use.
It provides no security against any determined attacker, as it is easily cracked with simple frequency analysis.
Implementation using Python
So we can implement this algorithm in different ways −
Using For Loop and ord() function
We will first encrypt the given message and generate an ROT13 code using a for loop. In this program, the for loop will be used to iterate over each character in the given text. If the character is a letter, we will keep it (uppercase or lowercase) and shift it by thirteen places. Non-alphabetic characters will remain unchanged. Also we will use the ord() function of Python which is used to change a single Unicode character into its integer representation.
Example
Below is a simple Python code for ROT13 cryptography. See the code below −
# Encryption Function def rot13_encrypt(text): encrypted_text = '' for char in text: if char.isalpha(): shifted = ord(char) + 13 if char.islower(): if shifted > ord('z'): shifted -= 26 else: if shifted > ord('Z'): shifted -= 26 encrypted_text += chr(shifted) else: encrypted_text += char return encrypted_text # Decryption Function def rot13_decrypt(text): decrypted_text = '' for char in text: if char.isalpha(): shifted = ord(char) - 13 # Decryption involves shifting back by 13 if char.islower(): if shifted < ord('a'): shifted += 26 else: if shifted < ord('A'): shifted += 26 decrypted_text += chr(shifted) else: decrypted_text += char return decrypted_text # function execution message = "Hello, Tutorialspoint!" encrypted_msg = rot13_encrypt(message) print("The Encrypted message:", encrypted_msg) decrypted_msg = rot13_decrypt( encrypted_msg) print("The Decrypted message:", decrypted_msg)
Following is the output of the above example −
Input/Output
The Encrypted message: Uryyb, Ghgbevnyfcbvag! The Decrypted message: Hello, Tutorialspoint!
Using list comprehension
In this example, list comprehension is used to perform the ROT13 encryption. Using the list we will iterate over each character in the input text using the for char in the text part at the end. For each character in the input text we will use a conditional expression to find the encrypted value.
Example
Below is a simple Python code for the ROT13 algorithm. See the program below −
# Encryption function def rot13_encrypt(text): encrypted_text = ''.join([chr(((ord(char) - 65 + 13) % 26) + 65) if 'A' <= char <= 'Z' else chr(((ord(char) - 97 + 13) % 26) + 97) if 'a' <= char <= 'z' else char for char in text]) return encrypted_text # Decryption function def rot13_decrypt(text): decrypted_text = ''.join([chr(((ord(char) - 65 - 13) % 26) + 65) if 'A' <= char <= 'Z' else chr(((ord(char) - 97 - 13) % 26) + 97) if 'a' <= char <= 'z' else char for char in text]) return decrypted_text # Function execution message = "Hello, Everyone!" encrypted_msg = rot13_encrypt(message) print("The Encrypted message:", encrypted_msg) decrypted_msg = rot13_decrypt( encrypted_msg) print("The Decrypted message:", decrypted_msg)
Following is the output of the above example −
Input/Output
The Encrypted message: Uryyb, Rirelbar! The Decrypted message: Hello, Everyone!
Using Dictionary
In this example we are going to use two dictionaries to implement the program for ROT13. So the first dictionary will map uppercase letters to their index in the alphabet. And the second dictionary will map shifted indices back to uppercase letters, from 'Z' to 'A'. The encryption function sends the resultant index back to a letter using the second dictionary after identifying each letter's index using the first dictionary and adding the shift value. And in the decryption function we will reverse the process.
Example
Following is a simple Python program of ROT13 algorithm in which will use two dictionaries. Check the code below −
# Dictionary to lookup the index dictionary1 = {'A': 1, 'B': 2, 'C': 3, 'D': 4, 'E': 5, 'F': 6, 'G': 7, 'H': 8, 'I': 9, 'J': 10, 'K': 11, 'L': 12, 'M': 13, 'N': 14, 'O': 15, 'P': 16, 'Q': 17, 'R': 18, 'S': 19, 'T': 20, 'U': 21, 'V': 22, 'W': 23, 'X': 24, 'Y': 25, 'Z': 26} # Dictionary to lookup alphabets dictionary2 = {0: 'Z', 1: 'A', 2: 'B', 3: 'C', 4: 'D', 5: 'E', 6: 'F', 7: 'G', 8: 'H', 9: 'I', 10: 'J', 11: 'K', 12: 'L', 13: 'M', 14: 'N', 15: 'O', 16: 'P', 17: 'Q', 18: 'R', 19: 'S', 20: 'T', 21: 'U', 22: 'V', 23: 'W', 24: 'X', 25: 'Y'} # Encryption Function def encrypt(msg, shift): cipher = '' for letter in msg: # check for space if letter != ' ': num = (dictionary1[letter] + shift) % 26 cipher += dictionary2[num] else: cipher += ' ' return cipher # Decryption Function def decrypt(msg, shift): decipher = '' for letter in msg: # checks for space if letter != ' ': num = (dictionary1[letter] - shift + 26) % 26 decipher += dictionary2[num] else: decipher += ' ' return decipher msg = "Hey Tutorialspoint" shift = 13 result = encrypt(msg.upper(), shift) print("The Encrypted message: ", result) msg = "URL GHGBEVNYFCBVAG" shift = 13 result = decrypt(msg.upper(), shift) print("The Decrypted message: ", result)
Following is the output of the above example −
Input/Output
The Encrypted message: URL GHGBEVNYFCBVAG The Decrypted message: HEY TUTORIALSPOINT
Implementation using C++
To implement the ROT13 algorithm we will use C++ programming langugage. Using the rot13Func function we will encrypt the given string message. Each letter in the given input text is iterated over. To get the matching ROT13 character, it alters the character by 13 positions if it is an alphabet letter. The main function calls the rot13Func function and outputs the encrypted text.
Example
#include <iostream> #include <string> using namespace std; // Function to perform rot13Func encryption string rot13Func(string text) { for (char& c : text) { if (isalpha(c)) { char base = islower(c) ? 'a' : 'A'; c = (c - base + 13) % 26 + base; } } return text; } int main() { string plaintext = "Hello this world is so beautiful"; cout << "The Plaintext Message is: " << plaintext << endl; string encrypted_text = rot13Func(plaintext); cout << "Encrypted text: " << encrypted_text << endl; return 0; }
Following is the output of the above example −
Input/Output
The Plaintext Message is: Hello this world is so beautiful Encrypted text: Uryyb guvf jbeyq vf fb ornhgvshy
Implementation using Java
In this implementation we are going to use Java programming langugage to create ROT13 algorithm. Using a string text as input, the rot13Func method encrypts it using ROT13. Every character in the input text is iterated over. To get the matching ROT13 character, it alters the character by 13 positions if it is an alphabet letter. After receiving input message, the main method calls the rot13 function and outputs the encrypted text.
Example
public class ROT13Class { // Function to perform ROT13 encryption public static String rot13Func(String text) { StringBuilder result = new StringBuilder(); for (char c : text.toCharArray()) { if (Character.isLetter(c)) { char base = Character.isLowerCase(c) ? 'a' : 'A'; c = (char) (((c - base + 13) % 26) + base); } result.append(c); } return result.toString(); } public static void main(String[] args) { String plaintext = "The world is so beautiful!"; System.out.println("The Plain Text Message: " + plaintext); String encryptedText = rot13Func(plaintext); System.out.println("The Encrypted text: " + encryptedText); } }
Following is the output of the above example −
Input/Output
The Plain Text Message: The world is so beautiful! The Encrypted text: Gur jbeyq vf fb ornhgvshy!
Drawback
As the ROT13 cipher is really a special case application of the Caesar cipher, it is not very secure. Although the ROT13 cipher can be cracked by simply moving the letters 13 positions, the Caesar cipher can only be cracked via frequency analysis or by trying all 25 keys. It is therefore useless in real life.
Analysis of ROT13 Algorithm
ROT13 cipher algorithm is considered as special case of Caesar Cipher. It is not a very secure algorithm and can be broken easily with frequency analysis or by just trying possible 25 keys whereas ROT13 can be broken by shifting 13 places. Therefore, it does not include any practical use.
Summary
We have examined the Python cryptography ROT13 algorithm in this article. ROT13 is a quick and efficient method of encrypting a given message by moving each letter in the alphabet by 13 positions. It is mostly used for basic encryption tasks. We have implemented different ways in this chapter so you can now encrypt and decrypt messages using the ROT13 algorithm in Python.
To Continue Learning Please Login