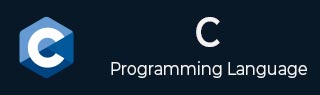
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C Programming - Online Quiz
Following quiz provides Multiple Choice Questions (MCQs) related to C Programming Framework. You will have to read all the given answers and click over the correct answer. If you are not sure about the answer then you can check the answer using Show Answer button. You can use Next Quiz button to check new set of questions in the quiz.
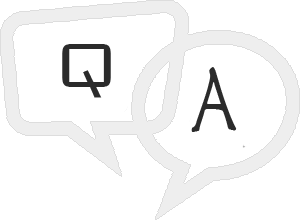
Q 1 - What is the output of the following program?
#include<stdio.h> void f() { static int i; ++i; printf("%d", i); } main() { f(); f(); f(); }
Answer : D
Explanation
1 2 3, A static local variables retains its value between the function calls and the default value is 0.
Q 2 - What is the output of the following program?
#include<stdio.h> main() { int a[] = {1,2}, *p = a; printf("%d", p[1]); }
Answer : B
Explanation
2, as ‘p’ holds the base address then we can access array using ‘p’ just like with ‘a’
Q 3 - What is the output of the following program?
#include<stdio.h> main() { union abc { int x; char ch; }var; var.ch = 'A'; printf("%d", var.x); }
Answer : C
Explanation
65, as the union variables share common memory for all its elements, x gets ‘A’ whose ASCII value is 65 and is printed.
Q 4 - What is the output of the following program?
#include<stdio.h> main() { int a[] = {10, 20, 30}; printf("%d", *a+1); }
Answer : C
Explanation
*a refers to 10 and adding a 1 to it gives 11.
Q 5 - What is the output of the following program?
#include<stdio.h> main() { int x; float y; y = x = 7.5; printf("x=%d y=%f", x, y); }
Answer : A
Explanation
‘x’ gets the integral value from 7.5 which is 7 and the same is initialized to ‘y’.
Q 6 - In Windows & Linux, how many bytes exist for near, far and huge pointers?
Answer : B
Explanation
In DOS, numbers of byte exist for near pointer = 2, far pointer = 4 and huge pointer = 4.
In Windows and Linux, numbers of byte exist for near pointer = 4, far pointer = 4 and huge pointer = 4.
Q 7 - A bitwise operator “&” can turn-off a particular bit into a number.
Answer : A
Explanation
The bitwise AND operator “&” compares each bit of the first operand with the corresponding bit of the second operand. During comparison, if both operands bits are 1, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0.
Q 8 - What do you mean by “int (*ptr)[10]”
A - ptr is an array of pointers to 10 integers
B - ptr is a pointer to an array of 10 integers
Answer : B
Explanation
Explanation: with or without the brackets surrounding the *p, still the declaration says it’s an array of pointer to integers.
Q 9 - Choose the correct unary operators in C – a) !, b) ~, c) ^&, d) ++
Answer : A
Explanation
In C, these are the unary operators,
Logical NOT = ! Address-of = & Cast Operator = ( ) Pointer dereference = * Unary Plus = + Increment = ++ Unary negation = –
Q 10 - Choose the function that is most appropriate for reading in a multi-word string?
Answer : D
Explanation
gets (); = Collects a string of characters terminated by a new line from the standard input stream stdin
To Continue Learning Please Login