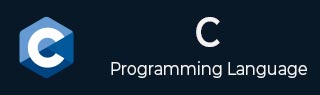
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Pragmas
- C - Preprocessor Operators
- C - Macros
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Pointer vs Array in C
Arrays and Pointers are two important language constructs in C, associated with each other in many ways. In many cases, the tasks that you perform with a pointer can also be performed with the help of an array.
However, there are certain conceptual differences between arrays and pointers. Read this chapter to understand their differences and comparative advantages and disadvantages.
Arrays in C
In a C program, an array is an indexed collection of elements of similar type, stored in adjacent memory locations.
To declare an array, we use the following syntax −
data_type arr_name [size];
The size should be a non-negative integer. For example −
int arr[5];
The array can be initialized along with the declaration, with the elements given as a comma-separated list inside the curly brackets. Mentioning its size is optional.
int arr[] = {1, 2, 3, 4, 5};
Each element in an array is characterized by a unique integral index, starting from "0". In C language, the lower bound of an array is always "0" and the upper bound is "size – 1".
Example of an Array
The following example shows how you can traverse an array with indexed subscripts −
#include <stdio.h> int main (){ /* an array with 5 elements */ int arr[5] = {10, 20, 30, 40, 50}; int i; /* output each array element's value */ printf("Array values with subscripts: \n"); for(i = 0; i < 5; i++){ printf("arr[%d]: %d\n", i, arr[i]); } return 0; }
Output
When you run this code, it will produce the following output −
Array values with subscripts: arr[0]: 10 arr[1]: 20 arr[2]: 30 arr[3]: 40 arr[4]: 50
Pointers in C
C allows you to access the memory location of a variable that has been randomly allocated by the compiler. The address−of operator (&) returns the address of the variable.
A variable that stores the address of another variable is called a pointer. The type of the pointer must be the same as the one whose address it stores.
To differentiate from the target variable type, the name of the pointer is prefixed with an asterisk (*). If we have an int variable, its pointer is declared as "int *".
int x = 5; int *y = &a;
Note: In case of an array, the address of its 0th element is assigned to the pointer.
int arr[] = {1, 2, 3, 4, 5}; int *ptr = &arr[0];
In fact, the name of the array itself resolves to the address of the 0th element.

Hence, we can as well write −
int *ptr = arr;
Since the elements of an array are placed in adjacent memory locations and the address of each subscript increments by 4 (in case of an int array), we can use this feature to traverse the array elements with the help of pointer as well.
Example of a Pointer
The following example shows how you can traverse an array with a pointer −
#include <stdio.h> int main (){ /* an array with 5 elements */ int arr[5] = {10, 20, 30, 40, 50}; int *x = arr; int i; /* output each array element's value */ printf("Array values with pointer: \n"); for(i = 0; i < 5; i++) { printf("arr[%d]: %d\n", i, *(x+i)); } return 0; }
Output
Run the code and check its output −
Array values with pointer arr[0]: 10 arr[1]: 20 arr[2]: 30 arr[3]: 40 arr[4]: 50
Difference between Arrays and Pointers in C
The following table highlights the important differences between arrays and pointers −
Array | Pointer |
---|---|
It stores the elements of a homogeneous data type in adjacent memory locations. | It stores the address of a variable, or an array |
An array is defined as a collection of similar datatypes. | A pointer is a variable that stores address of another variable. |
The number of variables that can be stored is decided by the size of the array. | A pointer can store the address of only a single variable. |
The initialization of arrays can be done while defining them. | Pointers cannot be initialized while defining them. |
The nature of arrays is static. | The nature of pointers is dynamic. |
Arrays cannot be resized according to the user's requirements. | Pointers can be resized at any point in time. |
The allocation of an array is done at compile time. | The allocation of the pointer is done at run time. |
To Continue Learning Please Login