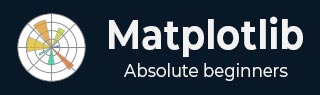
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Legends
In general, a legend in a graph provides a visual representation of the data depicted along the Y-axis, often referred to as the graph series. It is a box containing a symbol and a label for each series in the graph. A series could be a line in a line chart, a bar in a bar chart, and so on. The legend is helpful when you have multiple data series on the same plot, and you want to distinguish between them. In the following image, we can observe the legend in a plot (highlighted with a red color rectangle) −

Adding legend to a matplotlib plot
To add a legend to a Matplotlib plot, you typically use the matplotlib.pyplot.legend() function. This function is used to add a legend to the Axes, providing a visual guide for the elements in the plot.
Syntax
Following is the syntax of the function
matplotlib.pyplot.legend(*args, **kwargs)
The function can be called in different ways, depending on how you want to customize the legend.
Matplotlib automatically determines the elements to be added to the legend while call legend() without passing any extra arguments,. The labels for these elements are taken from the artists in the plot. You can specify these labels either at the time of creating the artist or by using the set_label() method.
Example 1
In this example, the legend takes the data from the label argument is used when creating the plot.
import matplotlib.pyplot as plt # Example data x = [1, 2, 3] y = [2, 4, 6] # Plotting the data with labels line, = plt.plot(x, y, label='Legend describing a single line') # Adding a legend plt.legend() # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
Output

Successfully Placed a legend on the Axes...
Example 2
Here is the alternative way of using the set_label() method on the artist.
import matplotlib.pyplot as plt # Example data x = [1, 2, 3] y = [2, 4, 6] # Plotting the data with labels line, = plt.plot(x, y) line.set_label('Place a legend via method') # Adding a legend plt.legend() # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
Output

Successfully Placed a legend on the Axes...
Manually adding the legend
You can pass an iterable of legend artists followed by an iterable of legend labels to explicitly control which artists have a legend entry.
Example 1
Here is an example of calling the legend() function by listing artists and labels.
import matplotlib.pyplot as plt # Example data x = [1, 2, 3] y1 = [2, 4, 6] y2 = [1, 3, 5] y3 = [3, 6, 9] # Plotting the data line1, = plt.plot(x, y1) line2, = plt.plot(x, y2) line3, = plt.plot(x, y3) # calling legend with explicitly listed artists and labels plt.legend([line1, line2, line3], ['Label 1', 'Label 2', 'Label 3']) # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
Output

Successfully Placed a legend on the Axes...
Example 2
Here is an example of calling the legend() function only by listing the artists. This approach is similar to the previous one but in this case, the labels are taken from the artists' label properties.
import matplotlib.pyplot as plt # Example data x = [1, 2, 3] y1 = [2, 4, 6] y2 = [1, 3, 5] # Plotting the data with labels line1, = plt.plot(x, y1, label='Label 1') line2, = plt.plot(x, y2, label='Label 2') # Adding a legend with explicitly listed artists plt.legend(handles=[line1, line2]) # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
Output

Successfully Placed a legend on the Axes...
Example 3
In this example, we first plot two sets of data, and then we add a legend by calling the legend() function with a list of strings representing the legend items.
import matplotlib.pyplot as plt # Example data x = [1, 2, 3, 4, 5, 6] y1 = [1, 4, 2, 6, 8, 5] y2 = [1, 5, 3, 7, 9, 6] # Plotting the data plt.plot(x, y1) plt.plot(x, y2) # Adding a legend for existing plot elements plt.legend(['First line', 'Second line'], loc='center') # Show the plot plt.show() print('Successfully Placed a legend on the Axes...')
Output

Successfully Placed a legend on the Axes...
Adding legend in subplots
To add the legends in each subplot, we can use the legend() function on each axes object of the figure.
Example 1
Here is an example that adds the legend to the each subplot of a matplotlib figure. This approach uses the legend() function on the axes object.
import numpy as np from matplotlib import pyplot as plt plt.rcParams["figure.figsize"] = [7, 3.50] plt.rcParams["figure.autolayout"] = True # Sample data x = np.linspace(-2, 2, 100) y1 = np.sin(x) y2 = np.cos(x) y3 = np.tan(x) # Create the figure with subplots f, axes = plt.subplots(3) # plot the data on each subplot and add lagend axes[0].plot(x, y1, c='r', label="sine") axes[0].legend(loc='upper left') axes[1].plot(x, y2, c='g', label="cosine") axes[1].legend(loc='upper left') axes[2].plot(x, y3, c='b', label="tan") axes[2].legend(loc='upper left') # Display the figure plt.show()
Output
On executing the above code we will get the following output −

Adding multiple legends in one axes
To draw multiple legends on the same axes in Matplotlib, we can use the axes.add_artist() method along with the legend() function.
Example 2
The following example demonstrates how to add multiple legends in one axes of the matplotlib figure.
from matplotlib import pyplot as plt plt.rcParams["figure.figsize"] = [7, 4] plt.rcParams["figure.autolayout"] = True # plot some data line1, = plt.plot([1, 2, 3], label="Line 1", linestyle='--') line2, = plt.plot([3, 2, 1], label="Line 2", linewidth=4) # Add first legend at upper right of the axes first_legend = plt.legend(handles=[line1], loc='upper right') # Get the current axes to add legend plt.gca().add_artist(first_legend) # Add second legend at lower right of the axes plt.legend(handles=[line2], loc='lower right') # Display the output plt.show()
Output
On executing the above code we will get the following output −

To Continue Learning Please Login