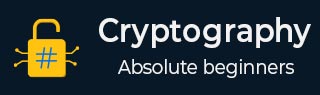
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Reverse Cipher
What is a Reverse Cipher ?
A message can be encrypted by printing it in reverse order using a Reverse Cipher algorithm. So we can encrypt the words "Hi this is Robot" is encrypted to "toboR is siht iH". Simply reverse the reversed message to get the original message if you want to decode it. The process for encryption and decryption are the same.
So as we can see, the reverse cipher is a very weak cipher. It is visible from the ciphertext that it is just in reverse order.
But, we will utilize the Reverse Cipher program as our initial encryption program because its code is simple to understand.

Algorithm
Here's the algorithm for the Reverse Cipher for encryption and decryption both −
Encryption Algorithm
A plain text message will be the input of the encryption technique, and a ciphered text message will be the output. So, the following are the steps −
Step 1: Take input as a plain text message as input.
Step 2: Then reverse the order of characters in the plain text message to create the cipher text message.
Step 3: Return the cipher text message as output.
Decryption Algorithm
Additionally, a cipher text message will be the input of the decryption method, and a plain text message will be the result. So the steps for this algorithm is as follows −
Step 1: Take input as a cipher text message as input.
Step 2: After that reverse the order of characters in the cipher text message to get the original plain text message.
Step 3: Lastly return the plain text message as output.
Example
The reverse cipher is among the most basic types of ciphers. A plain text can be encrypted by simply reversing it's character order. The cipher text is the outcome. For example, the reverse cipher is used to encrypt the message "HAT" as "TAH." Using the reverse cipher, the message "HELLO WORLD" is encrypted as "DLROW OLLEH." Reversing the sequence is the only difference between the methods used to encrypt and decode a message. Thus, the message "TAH" would be decoded as "HAT". Decrypting the message "DLROW OLLEH" would be the "HELLO WORLD."
The reverse cipher's strength is how simple it is to use and comprehend. Children may use it to send their friends "secret messages." The reverse cipher does not perform particularly well if the purpose of encryption is to make our communication secure enough that a third party cannot read it, as it is a simple cipher to break.
Implementations
So we can implement this algorithm in different ways by using different modules of functions of Python. Let us see these ways one by one in the below sections −
Using Python
Using C++
Using Java
Using Python
As we have to implement the Reverse Cipher algorithm using Python so we will use python's string manipulation feature. We will use slicing to reverse the given plain text message inside the function.
Python's slicing functionality is used in the expression message[::-1] to change the message's character order.
In Python, reversing a string can be done by using the notation [::-1], which means to begin at the end of the string and move backward with a step size of -1.
Below is the implementation of Reverse Cipher using str manipulation of Python −
Example
def reverse_cipher(message): # Reverse the message using slicing reversed_message = message[::-1] return reversed_message # function execution message = "Hiii! this is a reverse cipher algorithm" encrypted_message = reverse_cipher(message) print("The Original Message: ", message) print("The Encrypted message:", encrypted_message)
Following is the output of the above example −
Input/Output
The Original Message: Hiii! this is a reverse cipher algorithm The Encrypted message: mhtirogla rehpic esrever a si siht !iiiH
Using C++
This Code is implemented in C++, basically shows how a reverse cipher works. It starts with defining the key message. After that, it encrypts the message using the encrypt function, which reverses the behaviour of the message, and stores the outcome in an encryptedMessage. The encrypted message is then decrypted using the decrypt function, which returns the characters to their original form and records the result in a decrypted message. In order to show how to utilise the reverse cypher method for encryption and decryption, it displays the original message, the encrypted message, and the decrypted message at the end.
Here is the implementation of Reverse Cipher using C++ −
Example
#include <iostream> #include <string> std::string encrypt(std::string plaintext) { std::string ciphertext = ""; for (int i = plaintext.length() - 1; i >= 0; i--) { ciphertext += plaintext[i]; } return ciphertext; } std::string decrypt(std::string ciphertext) { std::string plaintext = ""; for (int i = ciphertext.length() - 1; i >= 0; i--) { plaintext += ciphertext[i]; } return plaintext; } int main() { std::string message = "Hello, This is a Reverse Cipher example!"; std::string encryptedMessage = encrypt(message); std::string decryptedMessage = decrypt(encryptedMessage); std::cout << "Our Original Message: " << message << std::endl; std::cout << "The Encrypted message: " << encryptedMessage << std::endl; std::cout << "The Decrypted message: " << decryptedMessage << std::endl; return 0; }
Following is the output of the above example −
Input/Output
Our Original Message: Hello, This is a Reverse Cipher example! The Encrypted message: !elpmaxe rehpiC esreveR a si sihT ,olleH The Decrypted message: Hello, This is a Reverse Cipher example!
Using Java
The main function of the provided Java code is to use the ReverseCipher class to encrypt the message and implement the reverse cipher method. It begins by explaining the main message, "Hello, Tutorialspoint!" Then, it uses the encrypt method to encrypt the message, which converts the message. Then, it parses the given message, redirecting the characters back to the original message. Finally, it prints the original message, the encrypted message, and the decrypted message to the console, displaying the encryption and decryption process.
Following is the implementation of Reverse Cipher using Java −
Example
// Class for reverse cipher public class ReverseCipher { public static String encrypt(String plaintext) { StringBuilder ciphertext = new StringBuilder(); for (int i = plaintext.length() - 1; i >= 0; i--) { ciphertext.append(plaintext.charAt(i)); } return ciphertext.toString(); } public static String decrypt(String ciphertext) { StringBuilder plaintext = new StringBuilder(); for (int i = ciphertext.length() - 1; i >= 0; i--) { plaintext.append(ciphertext.charAt(i)); } return plaintext.toString(); } public static void main(String[] args) { String message = "Hello, Tutorialspoint!"; String encryptedMsg = encrypt(message); String decryptedMsg = decrypt(encryptedMsg); System.out.println("The Original message is: " + message); System.out.println("The Encrypted message is: " + encryptedMsg); System.out.println("The Decrypted message is: " + decryptedMsg); } }
Following is the output of the above example −
Input/Output
The Original message is: Hello, Tutorialspoint! The Encrypted message is: !tniopslairotuT ,olleH The Decrypted message is: Hello, Tutorialspoint!
Features
Below are some feature of reverse cipher algorithm −
The Reverse Cipher is used to change a plaintext string into ciphertext by reversing it according to the given pattern.
Both encryption and decryption work in the same way.
In the decryption process the user only needs to reverse the cipher text for getting the plain text.
Drawback
The big drawback of the reverse cipher algorithm is that it is very weak. So the hacker can easily break the ciphertext to know the actual or original message. Hence, reverse cipher is not considered a good option to maintain a secure communication channel.
So Reverse Cipher can be used for educational purposes or for very basic encryption needs. And this algorithm is not suitable for securing confidential information or communication channels as it is vulnerable.Summary
Overall Reverse Cipher is a very simple cryptography algorithm or method with the help of it the characters of a message are reversed to create the ciphertext. As it is easy to understand and implement. It is also very weak in terms of security. Both encryption and decryption have the same process of reversing the text.
But, this algorithm is not a reliable way for securing very sensitive information. As it is easy to crack and hack which means it is very vulnerable. So, it can be used for educational purposes.
To Continue Learning Please Login