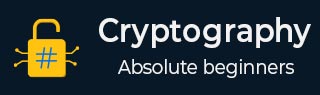
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Encryption of Simple Substitution Cipher
In the previous chapter we saw what exactly a simple Substitution Cipher is, How it works, Basic implementation and its drawbacks. Now we will see different ways to implement the simple substitution cipher with the help of Python, Java and c++.
Implementation in Python
So we can implement the encryption of simple substitution cipher using different methods like −
Using a specified shift value
Using a Dictionary Mapping
Using ASCII Values Directly
Therefore, we will try to provide a detailed concept of each of these approaches in the sections below so that you may better grasp simple substitution cipher encryption.
Approach 1: Using a specified shift value
In a substitution cipher, each letter in the plaintext is replaced by a letter some fixed number of positions down the alphabet. The main concept used in this approach is that we will encrypt the plaintext using a specified shift value. Shift is the number of positions each letter should be shifted in the alphabet.
Here is the implementation of this approach using Python −
Example
# encryption function def substitution_encrypt(plain_text, shift): encrypted_text = "" for char in plain_text: # if the character is a letter if char.isalpha(): # find the ASCII value of the character ascii_val = ord(char) # if the character is uppercase or lowercase if char.isupper(): # shift the character within the range (65-90) shifted_ascii = ((ascii_val - 65 + shift) % 26) + 65 else: # shift the character within the range (97-122) shifted_ascii = ((ascii_val - 97 + shift) % 26) + 97 # change back to a character encrypted_char = chr(shifted_ascii) encrypted_text += encrypted_char else: encrypted_text += char return encrypted_text #our plain text example plaintext = "Hello, everyone!" shift = 5 encrypted_text = substitution_encrypt(plaintext, shift) print("Plaintext: ", plaintext) print("Encrypted text:", encrypted_text)
Following is the output of the above example −
Input/Output
Plaintext: Hello, everyone! Encrypted text: Mjqqt, jajwdtsj!
Approach 2: Using a Dictionary Mapping
Now we are going to use dictionary mapping to implement a simple substitution cipher encryption. So we create a "map" or "dictionary", with the help of it we will match each letter of the alphabet to another letter. For example, 'a' can be mapped to 'b', 'b' can be mapped to 'c', and so on. Then we will use this map to replace each letter in the plaintext with its respective mapped letter. If a letter is not found in the map (like punctuation or numbers), we keep it unchanged.
Below is the Python implementation for the simple substitution cipher encryption using dictionary mapping. See the program below −
Example
# encryption function def substitution_encrypt(plain_text, key): # a dictionary mapping for substitution mapping = {chr(97 + i): key[i] for i in range(26)} # Encrypt the plaintext encrypted_text = ''.join(mapping.get(char.lower(), char) for char in plain_text) return encrypted_text # our plain text example plaintext = "Hello, dear friend!" key = "bcdefghijklmnopqrstuvwxyza" # substitution key encrypted_text = substitution_encrypt(plaintext, key) print("Plaintext: ", plaintext) print("Encrypted text:", encrypted_text)
Following is the output of the above example −
Input/Output
Plaintext: Hello, dear friend! Encrypted text: ifmmp, efbs gsjfoe!
Approach 3: Using ASCII Values Directly
As you may know, every character or letter has a unique number related to it in computers called the "ASCII value". In this approach, we will directly manipulate these ASCII values to shift each letter in the plaintext. For example, 'a' might have an ASCII value of 97, so if we shift it by 5, it becomes 102, which is the letter 'f'.
So here is a Python implementation for the simple substitution cipher encryption using the above approach −
Example
# encryption function def substitution_encrypt(plain_text, shift): encrypted_text = "" for char in plain_text: if char.isalpha(): # use ASCII values directly to shift characters ascii_val = ord(char) shifted_ascii = ascii_val + shift if char.isupper(): if shifted_ascii > 90: shifted_ascii -= 26 elif shifted_ascii < 65: shifted_ascii += 26 elif char.islower(): if shifted_ascii > 122: shifted_ascii -= 26 elif shifted_ascii < 97: shifted_ascii += 26 encrypted_text += chr(shifted_ascii) else: encrypted_text += char return encrypted_text # function execution plaintext = "Hello, my dear colleague!" shift = 3 encrypted_text = substitution_encrypt(plaintext, shift) print("PlainText: ", plaintext) print("Encrypted text:", encrypted_text)
Following is the output of the above example −
Input/Output
PlainText: Hello, my dear colleague! Encrypted text: Khoor, pb ghdu froohdjxh!
Implementation using Java
In this Java implementation, we will create a simple substitution cipher algorithm. Here we are using a concept in which we have to replace each letter in the plaintext with a respective letter from the encrypted alphabet. We will create a mapping between the regular alphabet and the encrypted alphabet with the help of a HashMap. Then, for each letter in the plaintext, we will see its respective encrypted letter from the mapping and add it to the ciphertext. If a character is not in the mapping (for example, punctuation or spaces), we will leave it unchanged in the ciphertext.
Example
So the implementation for simple substitution cipher using Java is as follows −
import java.util.HashMap; import java.util.Map; public class SSC { private static final String alphabet = "abcdefghijklmnopqrstuvwxyz"; private static final String encrypted_alphabet = "bcdefghijklmnopqrstuvwxyza"; private static final Map<Character, Character> encryptionMap = new HashMap<>(); static { for (int i = 0; i < alphabet.length(); i++) { encryptionMap.put(alphabet.charAt(i), encrypted_alphabet.charAt(i)); } } public static String encrypt(String plaintext) { StringBuilder ciphertext = new StringBuilder(); for (char c : plaintext.toLowerCase().toCharArray()) { if (encryptionMap.containsKey(c)) { ciphertext.append(encryptionMap.get(c)); } else { ciphertext.append(c); } } return ciphertext.toString(); } public static void main(String[] args) { String plaintext = "Hello Tutorialspoint"; String encryptedText = encrypt(plaintext); System.out.println("Our Plaintext: " + plaintext); System.out.println("The Encrypted text: " + encryptedText); } }
Following is the output of the above example −
Input/Output
Our Plaintext: Hello Tutorialspoint The Encrypted text: ifmmp uvupsjbmtqpjou
Implementation using C++
This is the implementation using C++ for the simple substitution cipher. So we will use the same approach which we have used in the above implementation.
Example
The implementation using C++ is as follows −
#include <iostream> #include <unordered_map> #include <string> using namespace std; class SSC { private: const string alphabet = "abcdefghijklmnopqrstuvwxyz"; const string encrypted_alphabet = "bcdefghijklmnopqrstuvwxyza"; unordered_map<char, char> encryptionMap; public: SSC() { for (int i = 0; i < alphabet.length(); i++) { encryptionMap[alphabet[i]] = encrypted_alphabet[i]; } } string encrypt(string plaintext) { string ciphertext = ""; for (char c : plaintext) { if (encryptionMap.find(tolower(c)) != encryptionMap.end()) { ciphertext += encryptionMap[tolower(c)]; } else { ciphertext += c; } } return ciphertext; } }; int main() { SSC cipher; string plaintext = "hello there how are you!"; string encryptedText = cipher.encrypt(plaintext); cout << "Our Plaintext: " << plaintext << endl; cout << "The Encrypted text: " << encryptedText << endl; return 0; }
Following is the output of the above example −
Input/Output
Our Plaintext: hello there how are you! The Encrypted text: ifmmp uifsf ipx bsf zpv!
Summary
In this chapter we have discussed different approaches to implement simple substitution cipher encryption. These methods provide various ways to achieve simple substitution cipher encryption in Python, Java, and C++, each with its own advantages and implementation details.
To Continue Learning Please Login