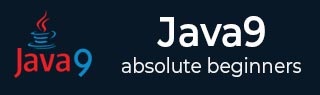
- Java 9 Tutorial
- Java 9 - Home
- Java 9 - Overview
- Java 9 - Environment Setup
- Java 9 - Module System
- Java 9 - REPL (JShell)
- Java 9 - Improved JavaDocs
- Java 9 - Multirelease JAR
- Java 9 - Collection Factory Methods
- Java 9 - Private Interface Methods
- Java 9 - Process API Improvements
- Java 9 - Stream API Improvements
- Try With Resources improvement
- Enhanced @Deprecated Annotation
- Inner Class Diamond Operator
- Optional Class Improvements
- Java 9 - Multiresolution Image API
- CompletableFuture API Improvements
- Java 9 - Miscellaneous Features
- java9 Useful Resources
- Java 9 - Questions and Answers
- Java 9 - Quick Guide
- Java 9 - Useful Resources
- Java 9 - Discussion
Java 9 - Process API Improvements
In Java 9 Process API which is responsible to control and manage operating system processes has been improved considerably. ProcessHandle Class now provides process's native process ID, start time, accumulated CPU time, arguments, command, user, parent process, and descendants. ProcessHandle class also provides method to check processes' liveness and to destroy processes. It has onExit method, the CompletableFuture class can perform action asynchronously when process exits.
Tester.java
import java.time.ZoneId; import java.util.stream.Stream; import java.util.stream.Collectors; import java.io.IOException; public class Tester { public static void main(String[] args) throws IOException { ProcessBuilder pb = new ProcessBuilder("notepad.exe"); String np = "Not Present"; Process p = pb.start(); ProcessHandle.Info info = p.info(); System.out.printf("Process ID : %s%n", p.pid()); System.out.printf("Command name : %s%n", info.command().orElse(np)); System.out.printf("Command line : %s%n", info.commandLine().orElse(np)); System.out.printf("Start time: %s%n", info.startInstant().map(i -> i.atZone(ZoneId.systemDefault()) .toLocalDateTime().toString()).orElse(np)); System.out.printf("Arguments : %s%n", info.arguments().map(a -> Stream.of(a).collect( Collectors.joining(" "))).orElse(np)); System.out.printf("User : %s%n", info.user().orElse(np)); } }
Output
You will see the following output.
Process ID : 5800 Command name : C:\Windows\System32\notepad.exe Command line : Not Present Start time: 2017-11-04T21:35:03.626 Arguments : Not Present User: administrator
Advertisements