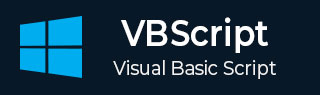
- VBScript Tutorial
- VBScript - Home
- VBScript - Overview
- VBScript - Syntax
- VBScript - Enabling
- VBScript - Placement
- VBScript - Variables
- VBScript - Constants
- VBScript - Operators
- VBScript - Decisions
- VBScript - Loops
- VBScript - Events
- VBScript - Cookies
- VBScript - Numbers
- VBScript - Strings
- VBScript - Arrays
- VBScript - Date
- VBScript Advanced
- VBScript - Procedures
- VBScript - Dialog Boxes
- VBScript - Object Oriented
- VBScript - Reg Expressions
- VBScript - Error Handling
- VBScript - Misc Statements
- VBScript Useful Resources
- VBScript - Questions and Answers
- VBScript - Quick Guide
- VBScript - Useful Resources
- VBScript - Discussion
VBScript - Error Handling
There are three types of errors in programming: (a) Syntax Errors, (b) Runtime Errors, and (c) Logical Errors.
Syntax errors
Syntax errors, also called parsing errors, occur at interpretation time for VBScript. For example, the following line causes a syntax error because it is missing a closing parenthesis −
<script type = "text/vbscript"> dim x,y x = "Tutorialspoint" y = Ucase(x </script>
Runtime errors
Runtime errors, also called exceptions, occur during execution, after interpretation. For example, the following line causes a runtime error because here syntax is correct but at runtime it is trying to call fnmultiply, which is a non-existing function −
<script type = "text/vbscript"> Dim x,y x = 10 y = 20 z = fnadd(x,y) a = fnmultiply(x,y) Function fnadd(x,y) fnadd = x+y End Function </script>
Logical errors
Logic errors can be the most difficult type of errors to track down. These errors are not the result of a syntax or runtime error. Instead, they occur when you make a mistake in the logic that drives your script and you do not get the result you expected. You cannot catch those errors, because it depends on your business requirement what type of logic you want to put in your program. For example, dividing a number by zero or a script that is written which enters into infinite loop.
Err Object
AAssume if we have a runtime error, then the execution stops by displaying the error message. As a developer, if we want to capture the error, then Error Object is used.
Example
In the below example, Err.Number gives the error number and Err.Description gives error description.
<script type = "text/vbscript"> Err.Raise 6 ' Raise an overflow error. MsgBox "Error # " & CStr(Err.Number) & " " & Err.Description Err.Clear ' Clear the error. </script>