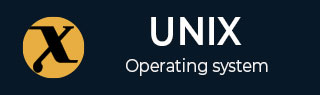
- Unix / Linux for Beginners
- Unix / Linux - Home
- Unix / Linux - What is Linux?
- Unix / Linux - Getting Started
- Unix / Linux - File Management
- Unix / Linux - Directories
- Unix / Linux - File Permission
- Unix / Linux - Environment
- Unix / Linux - Basic Utilities
- Unix / Linux - Pipes & Filters
- Unix / Linux - Processes
- Unix / Linux - Communication
- Unix / Linux - The vi Editor
- Unix / Linux Shell Programming
- Unix / Linux - Shell Scripting
- Unix / Linux - What is Shell?
- Unix / Linux - Using Variables
- Unix / Linux - Special Variables
- Unix / Linux - Using Arrays
- Unix / Linux - Basic Operators
- Unix / Linux - Decision Making
- Unix / Linux - Shell Loops
- Unix / Linux - Loop Control
- Unix / Linux - Shell Substitutions
- Unix / Linux - Quoting Mechanisms
- Unix / Linux - IO Redirections
- Unix / Linux - Shell Functions
- Unix / Linux - Manpage Help
- Advanced Unix / Linux
- Unix / Linux - Regular Expressions
- Unix / Linux - File System Basics
- Unix / Linux - User Administration
- Unix / Linux - System Performance
- Unix / Linux - System Logging
- Unix / Linux - Signals and Traps
Unix / Linux - Special Variables
In this chapter, we will discuss in detail about special variable in Unix. In one of our previous chapters, we understood how to be careful when we use certain nonalphanumeric characters in variable names. This is because those characters are used in the names of special Unix variables. These variables are reserved for specific functions.
For example, the $ character represents the process ID number, or PID, of the current shell −
$echo $$
The above command writes the PID of the current shell −
29949
The following table shows a number of special variables that you can use in your shell scripts −
Sr.No. | Variable & Description |
---|---|
1 |
$0 The filename of the current script. |
2 |
$n These variables correspond to the arguments with which a script was invoked. Here n is a positive decimal number corresponding to the position of an argument (the first argument is $1, the second argument is $2, and so on). |
3 |
$# The number of arguments supplied to a script. |
4 |
$* All the arguments are double quoted. If a script receives two arguments, $* is equivalent to $1 $2. |
5 |
$@ All the arguments are individually double quoted. If a script receives two arguments, $@ is equivalent to $1 $2. |
6 |
$? The exit status of the last command executed. |
7 |
$$ The process number of the current shell. For shell scripts, this is the process ID under which they are executing. |
8 |
$! The process number of the last background command. |
Command-Line Arguments
The command-line arguments $1, $2, $3, ...$9 are positional parameters, with $0 pointing to the actual command, program, shell script, or function and $1, $2, $3, ...$9 as the arguments to the command.
Following script uses various special variables related to the command line −
#!/bin/sh echo "File Name: $0" echo "First Parameter : $1" echo "Second Parameter : $2" echo "Quoted Values: $@" echo "Quoted Values: $*" echo "Total Number of Parameters : $#"
Here is a sample run for the above script −
$./test.sh Zara Ali File Name : ./test.sh First Parameter : Zara Second Parameter : Ali Quoted Values: Zara Ali Quoted Values: Zara Ali Total Number of Parameters : 2
Special Parameters $* and $@
There are special parameters that allow accessing all the command-line arguments at once. $* and $@ both will act the same unless they are enclosed in double quotes, "".
Both the parameters specify the command-line arguments. However, the "$*" special parameter takes the entire list as one argument with spaces between and the "$@" special parameter takes the entire list and separates it into separate arguments.
We can write the shell script as shown below to process an unknown number of commandline arguments with either the $* or $@ special parameters −
#!/bin/sh for TOKEN in $* do echo $TOKEN done
Here is a sample run for the above script −
$./test.sh Zara Ali 10 Years Old Zara Ali 10 Years Old
Note − Here do...done is a kind of loop that will be covered in a subsequent tutorial.
Exit Status
The $? variable represents the exit status of the previous command.
Exit status is a numerical value returned by every command upon its completion. As a rule, most commands return an exit status of 0 if they were successful, and 1 if they were unsuccessful.
Some commands return additional exit statuses for particular reasons. For example, some commands differentiate between kinds of errors and will return various exit values depending on the specific type of failure.
Following is the example of successful command −
$./test.sh Zara Ali File Name : ./test.sh First Parameter : Zara Second Parameter : Ali Quoted Values: Zara Ali Quoted Values: Zara Ali Total Number of Parameters : 2 $echo $? 0 $