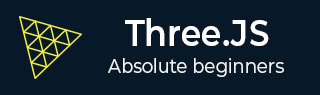
- Three.js Tutorial
- Three.js - Home
- Three.js - Introduction
- Three.js - Installation
- Three.js - Hello Cube App
- Three.js - Renderer and Responsiveness
- Three.js - Responsive Design
- Three.js - Debug and Stats
- Three.js - Cameras
- Three.js - Controls
- Three.js - Lights & Shadows
- Three.js - Geometries
- Three.js - Materials
- Three.js - Textures
- Three.js - Drawing Lines
- Three.js - Animations
- Three.js - Creating Text
- Three.js - Loading 3D Models
- Three.js - Libraries and Plugins
- Three.js Useful Resources
- Three.js - Quick Guide
- Three.js - Useful Resources
- Three.js - Discussion
Three.js - Hemisphere Light
It is a special light for creating natural lighting. If you look at the lighting outside, you'll see that the lights don't come from a single direction. Earth reflects part of the sunlight, and the atmosphere scatters the other parts. The result is a very soft light coming from lots of directions. In Three.js, we can create something similar using THREE.HemisphereLight.
const light = new THREE.HemisphereLight(color, groundColor, intensity)
The first argument sets the color of the sky, and the second color sets the color reflected from the floor. And the last argument is its intensity.
It is often used along with some other lights, which can cast shadows for the best outdoor lighting effect.
Example
Check out the following example.
hemisphere.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="ie=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Three.js - HemisphereLight</title> <style> * { margin: 0; padding: 0; box-sizing: border-box; font-family: -applesystem, BlinkMacSystemFont, 'Segoe UI', Roboto, Oxygen, Ubuntu, Cantarell, 'Open Sans', 'Helvetica Neue', sans-serif; } html, body { height: 100vh; width: 100vw; } #threejs-container { position: block; width: 100%; height: 100%; } </style> <script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/dat-gui/0.7.7/dat.gui.js"></script> </head> <body> <div id="container"></div> <script type="module"> // Adding hemisphere light in Three.js // It gives the lighting of physical world // GUI const gui = new dat.GUI() // sizes let width = window.innerWidth let height = window.innerHeight // scene const scene = new THREE.Scene() scene.background = new THREE.Color(0x262626) // camera const camera = new THREE.PerspectiveCamera(60, width / height, 0.1, 1000) camera.position.set(0, 0, 10) const camFolder = gui.addFolder('Camera') camFolder.add(camera.position, 'z', 10, 80, 1) camFolder.open() // lights const ambientLight = new THREE.AmbientLight(0xffffff, 0.5) scene.add(ambientLight) const skyColor = 0xb1e1ff // light blue const groundColor = 0xb97a20 // brownish const light = new THREE.HemisphereLight(skyColor, groundColor) light.position.set(0, 5, 0) scene.add(light) const helper = new THREE.HemisphereLightHelper(light, 5) scene.add(helper) // light controls const lightColor = { color: light.color.getHex(), groundColor: light.groundColor.getHex() } const lightFolder = gui.addFolder('Light') lightFolder .addColor(lightColor, 'color') .name('Light Color')const hemisphereLightFolder = gui.addFolder('Hemisphere Light') hemisphereLightFolder .addColor(lightColor, 'groundColor') .name('ground Color') .onChange(() => { light.groundColor.set(lightColor.groundColor) }) hemisphereLightFolder.add(light.position, 'x', -100, 100, 0.01) hemisphereLightFolder.add(light.position, 'y', -100, 100, 0.01) hemisphereLightFolder.add(light.position, 'z', -100, 100, 0.01) hemisphereLightFolder.open() // cube console.log('cube') const geometry = new THREE.BoxGeometry(2, 2, 2) const material = new THREE.MeshStandardMaterial({ color: 0x87ceeb }) const materialFolder = gui.addFolder('Material') materialFolder.add(material, 'wireframe') materialFolder.open() const cube = new THREE.Mesh(geometry, material) cube.position.set(0, 0.5, 0) cube.castShadow = true cube.receiveShadow = true scene.add(cube) // responsiveness window.addEventListener('resize', () => { width = window.innerWidth height = window.innerHeight camera.aspect = width / height camera.updateProjectionMatrix() renderer.setSize(window.innerWidth, window.innerHeight) renderer.render(scene, camera) }) // renderer const renderer = new THREE.WebGL1Renderer() renderer.setSize(window.innerWidth, window.innerHeight) renderer.shadowMap.enabled = true renderer.shadowMap.type = THREE.PCFSoftShadowMap renderer.setPixelRatio(Math.min(window.devicePixelRatio, 2)) // animation function animate() { requestAnimationFrame(animate) cube.rotation.x += 0.005 cube.rotation.y += 0.01 renderer.render(scene, camera) } // rendering the scene const container = document.querySelector('#container') container.append(renderer.domElement) renderer.render(scene, camera) animate() </script> </body> </html>
Output
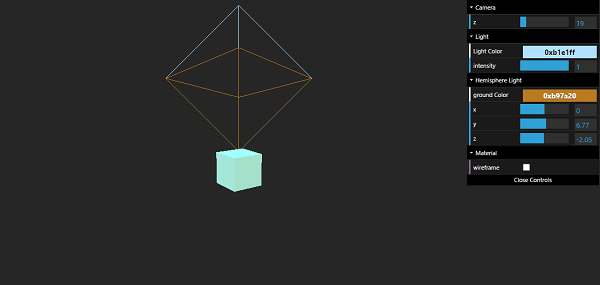
threejs_lights_and_shadows.htm
Advertisements