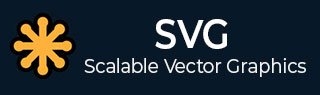
- SVG Tutorial
- SVG - Home
- SVG - Overview
- SVG - Shapes
- SVG - Text
- SVG - Stroke
- SVG - Filters
- SVG - Patterns
- SVG - Gradients
- SVG - Interactivity
- SVG - Linking
- SVG Demo
- SVG - Loaders
- SVG - Dialog
- SVG - Icons
- SVG - Clock
- SVG - Drag
- SVG - Key point
- SVG - Maps
- SVG - amChart
- SVG - Graph
- SVG - Flat Surface Shade
- SVG - Image Filter
- SVG - Text Effects
- SVG - Text With CSS Effects
- SVG - Arrow Effects
- SVG - Brand Effects
- SVG - Gooey Effects
- SVG - Gradients Effects
- SVG - Playful Effects
- SVG - Scroll Effects
- SVG - Side Show Effects
- SVG - Tab Effects
- SVG - Raphael.js Effects
- SVG - Velocity.js Effects
- SVG - Walkway.js Effects
- SVG - zPath.js Effects
- SVG - Vague.js Effects
- SVG - Transformation Effects
- SVG - Full Screen Overlay Effects
- SVG - Lazylinepainter.js Effects
- SVG - Demo Game
- SVG - Real Time SVG AD
- SVG Useful Resources
- SVG - Questions and Answers
- SVG - Quick Guide
- SVG - Useful Resources
- SVG - Discussion
SVG - Interactivity
SVG images can be made responsive to user actions. SVG supports pointer events, keyboard events and document events. Consider the following example.
Example
testSVG.htm<html> <title>SVG Interactivity</title> <body> <h1>Sample Interactivity</h1> <svg width="600" height="600"> <script type="text/JavaScript"> <![CDATA[ function showColor() { alert("Color of the Rectangle is: "+ document.getElementById("rect1").getAttributeNS(null,"fill")); } function showArea(event){ var width = parseFloat(event.target.getAttributeNS(null,"width")); var height = parseFloat(event.target.getAttributeNS(null,"height")); alert("Area of the rectangle is: " +width +"x"+ height); } function showRootChildrenCount() { alert("Total Children: "+document.documentElement.childNodes.length); } ]]> </script> <g> <text x="30" y="50" onClick="showColor()">Click me to show rectangle color.</text> <rect id="rect1" x="100" y="100" width="200" height="200" stroke="green" stroke-width="3" fill="red" onClick="showArea(event)"/> <text x="30" y="400" onClick="showRootChildrenCount()"> Click me to print child node count.</text> </g> </svg> </body> </html>
Explaination
SVG supports JavaScript/ECMAScript functions. Script block is to be in CDATA block consider character data support in XML.
SVG elements support mouse events, keyboard events. We've used onClick event to call a javascript functions.
In javascript functions, document represents SVG document and can be used to get the SVG elements.
In javascript functions, event represents current event and can be used to get the target element on which event got raised.
Output
Open textSVG.htm in Chrome web browser. You can use Chrome/Firefox/Opera to view SVG image directly without any plugin. Internet Explorer 9 and higher also supports SVG image rendering. Click on each text and rectangle to see the result.