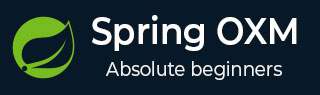
- Spring OXM Tutorial
- Spring OXM - Home
- Spring OXM - Overview
- Spring OXM - Environment Setup
- Spring OXM & JAXB
- Spring OXM - Create Project
- Spring OXM - Update Project JAXB2
- Spring OXM - Test JAXB2
- Spring OXM & XStream
- Spring OXM - Update Project
- Spring OXM - Test XStream
- Spring OXM & Castor
- Spring OXM - Update Project
- Spring OXM - Test Castor
- Spring OXM Useful Resources
- Spring OXM - Quick Guide
- Spring OXM - Useful Resources
- Spring OXM - Discussion
Spring OXM - Quick Guide
Spring OXM - Overview
The Spring Framework provides Object/XML or O/X mapping using global marshaller/unmarshaller interfaces and allows to switch O/X mapping frameworks easily. This process of converting an object to XML is called XML Marshalling/Serialization and conversion from XML to object is called XML Demarshalling/Deserialization.
Spring framework provides a Marshaller and UnMarshaller interfaces where Marshaller interface is responsible to marshall an object to XML and UnMarshaller interface deserializes the xml to an object. Following are the key benefits of using Spring OXM framework.
Easy Configuration − Using spring bean context factory, creation of marshaller/unmarshaller is quite easy and is configurable without worrying about O/X libraries structures like JAXB Context, JiBX binding factories etc. A marsaller/unmarshaller can be configured as any other bean.
Consistent Interfacing − Marshaller and UnMarshaller are global interfaces. These interfaces provides an abstraction layer over other O/X mapping frameworks and allows to switch between them without changing the code or with little code change.
Consistent Exception Handling − All underlying exceptions are mapped to a XmlMappingException as root exception. Thus developers need not to worry about underlying O/X mapping tool own exception hiearchy.
Marshaller
Marshaller is an interface with single method marshal.
public interface Marshaller { /** * Marshals the object graph with the given root into the provided Result. */ void marshal(Object graph, Result result) throws XmlMappingException, IOException; }
Where graph is any object to be marshalled and result is a tagging interface to represent the XML output. Following are the available types −
javax.xml.transform.dom.DOMResult − Represents org.w3c.dom.Node.
javax.xml.transform.sax.SAXResult − Represents org.xml.sax.ContentHandler.
javax.xml.transform.stream.StreamResult − Represents java.io.File, java.io.OutputStream, or java.io.Writer.
UnMarshaller
UnMarshaller is an interface with single method unmarshal.
public interface UnMarshaller { /** * Unmarshals the given provided Source into an object graph. */ Object unmarshal(Source source) throws XmlMappingException, IOException; }
Where source is a tagging interface to represent the XML input. Following are the available types −
javax.xml.transform.dom.DOMSource − Represents org.w3c.dom.Node.
javax.xml.transform.sax.SAXSource − Represents org.xml.sax.InputSource, and org.xml.sax.XMLReader.
javax.xml.transform.stream.StreamSource − Represents java.io.File, java.io.InputStream, or java.io.Reader.
Spring OXM - Environment Setup
This chapter will guide you on how to prepare a development environment to start your work with Spring Framework. It will also teach you how to set up JDK, Maven and Eclipse on your machine before you set up Spring Framework −
Setup Java Development Kit (JDK)
You can download the latest version of SDK from Oracle's Java site − Java SE Downloads. You will find instructions for installing JDK in downloaded files, follow the given instructions to install and configure the setup. Finally set PATH and JAVA_HOME environment variables to refer to the directory that contains java and javac, typically java_install_dir/bin and java_install_dir respectively.
If you are running Windows and have installed the JDK in C:\jdk-11.0.11, you would have to put the following line in your C:\autoexec.bat file.
set PATH=C:\jdk-11.0.11;%PATH% set JAVA_HOME=C:\jdk-11.0.11
Alternatively, on Windows NT/2000/XP, you will have to right-click on My Computer, select Properties → Advanced → Environment Variables. Then, you will have to update the PATH value and click the OK button.
On Unix (Solaris, Linux, etc.), if the SDK is installed in /usr/local/jdk-11.0.11 and you use the C shell, you will have to put the following into your .cshrc file.
setenv PATH /usr/local/jdk-11.0.11/bin:$PATH setenv JAVA_HOME /usr/local/jdk-11.0.11
Alternatively, if you use an Integrated Development Environment (IDE) like Borland JBuilder, Eclipse, IntelliJ IDEA, or Sun ONE Studio, you will have to compile and run a simple program to confirm that the IDE knows where you have installed Java. Otherwise, you will have to carry out a proper setup as given in the document of the IDE.
Setup Eclipse IDE
All the examples in this tutorial have been written using Eclipse IDE. So we would suggest you should have the latest version of Eclipse installed on your machine.
To install Eclipse IDE, download the latest Eclipse binaries from www.eclipse.org/downloads. Once you download the installation, unpack the binary distribution into a convenient location. For example, in C:\eclipse on Windows, or /usr/local/eclipse on Linux/Unix and finally set PATH variable appropriately.
Eclipse can be started by executing the following commands on Windows machine, or you can simply double-click on eclipse.exe
%C:\eclipse\eclipse.exe
Eclipse can be started by executing the following commands on Unix (Solaris, Linux, etc.) machine −
$/usr/local/eclipse/eclipse
After a successful startup, if everything is fine then it should display the following result −
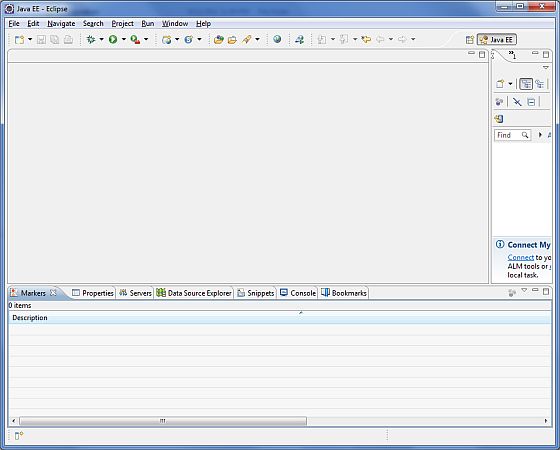
Set Maven
In this tutorial, we are using maven to run and build the spring based examples. Follow the Maven - Environment Setup to install maven.
Spring OXM - Create Project
Using eclipse, select File → New → Maven Project. Tick the Create a simple project (skip archetype selection) and click Next.
Enter the details, as shown below −
groupId − com.tutorialspoint
artifactId − springoxm
version − 0.0.1-SNAPSHOT
name − Spring OXM
description − Spring OXM Project
Click on Finish button and an new project will be created.
pom.xml
You can check the default content of pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>springoxm</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Spring OXM</name> <description>Spring OXM Project</description> </project>
Now as we've our project ready, let add following dependencies in pom.xml in next chapter.
Spring Core
Spring OXM
JAXB
Spring OXM - Update Project JAXB2
Update the content of pom.xml to have spring core, spring oxm and jaxb dependencies as shown below −
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>springoxm</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Spring OXM</name> <description>Spring OXM Project</description> <properties> <org.springframework.version>4.3.7.RELEASE</org.springframework.version> <org.hibernate.version>5.2.9.Final</org.hibernate.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${org.springframework.version}</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-oxm</artifactId> <version>${org.springframework.version}</version> <scope>compile</scope> </dependency> <dependency> <groupId>javax.xml.bind</groupId> <artifactId>jaxb-api</artifactId> <version>2.3.1</version> </dependency> <dependency> <groupId>org.glassfish.jaxb</groupId> <artifactId>jaxb-runtime</artifactId> <version>2.3.1</version> <scope>runtime</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${java.version}</source> <target>${java.version}</target> </configuration> </plugin> </plugins> </build> </project>
Create a class Student.java with O/X Annotation as shown below.
Student.java
package com.tutorialspoint.oxm.model; import javax.xml.bind.annotation.XmlAttribute; import javax.xml.bind.annotation.XmlElement; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Student{ String name; int age; int id; public String getName(){ return name; } @XmlElement public void setName(String name){ this.name = name; } public int getAge(){ return age; } @XmlElement public void setAge(int age){ this.age = age; } public int getId(){ return id; } @XmlAttribute public void setId(int id){ this.id = id; } }
Create applicationcontext.xml in src → main → resources with the following content.
applicationcontext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:oxm="http://www.springframework.org/schema/oxm" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/oxm http://www.springframework.org/schema/oxm/spring-oxm-3.0.xsd"> <oxm:jaxb2-marshaller id="jaxbMarshaller"> <oxm:class-to-be-bound name="com.tutorialspoint.oxm.model.Student"/> </oxm:jaxb2-marshaller> </beans>
Spring OXM - Test JAXB2
Create a main class OXMApplication.java with marshaller and unmarshaller objects. The objective of this class is to marshall a student object to student.xml using marshaller object and then unmarshall the student.xml to student object using unmarshaller object.
Example
OXMApplication.java
package com.tutorialspoint.oxm; import java.io.FileInputStream; import java.io.FileWriter; import java.io.IOException; import javax.xml.transform.stream.StreamResult; import javax.xml.transform.stream.StreamSource; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import org.springframework.oxm.Marshaller; import org.springframework.oxm.Unmarshaller; import org.springframework.oxm.XmlMappingException; import com.tutorialspoint.oxm.model.Student; public class OXMApplication { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("applicationcontext.xml"); Marshaller marshaller = (Marshaller)context.getBean("jaxbMarshaller"); Unmarshaller unmarshaller = (Unmarshaller)context.getBean("jaxbMarshaller"); // create student object Student student = new Student(); student.setAge(14); student.setName("Soniya"); try { marshaller.marshal(student, new StreamResult(new FileWriter("student.xml"))); System.out.println("Student marshalled successfully."); FileInputStream is = new FileInputStream("student.xml"); Student student1 = (Student)unmarshaller.unmarshal(new StreamSource(is)); System.out.println("Age: " + student1.getAge() + ", Name: " + student1.getName()); } catch(IOException | XmlMappingException ex) { ex.printStackTrace(); } } }
Output
Right click in the content area of the file in eclipse and then select Run as java application and verify the output.
Oct 10, 2021 8:48:12 PM org.springframework.context.support.ClassPathXmlApplicationContext prepareRefresh INFO: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@1e127982: startup date [Sun Oct 10 20:48:12 IST 2021]; root of context hierarchy Oct 10, 2021 8:48:12 PM org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions INFO: Loading XML bean definitions from class path resource [applicationcontext.xml] Oct 10, 2021 8:48:13 PM org.springframework.oxm.jaxb.Jaxb2Marshaller createJaxbContextFromClasses INFO: Creating JAXBContext with classes to be bound [class com.tutorialspoint.oxm.model.Student] Student marshalled successfully. Age: 14, Name: Soniya
Spring OXM - Update Project XStream
Update the content of pom.xml to have xstream dependencies as shown below −
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>springoxm</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Spring OXM</name> <description>Spring OXM Project</description> <properties> <org.springframework.version>4.3.7.RELEASE</org.springframework.version> <org.hibernate.version>5.2.9.Final</org.hibernate.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${org.springframework.version}</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-oxm</artifactId> <version>${org.springframework.version}</version> <scope>compile</scope> </dependency> <dependency> <groupId>com.thoughtworks.xstream</groupId> <artifactId>xstream</artifactId> <version>1.4.8</version> <scope>compile</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${java.version}</source> <target>${java.version}</target> </configuration> </plugin> </plugins> </build> </project>
Update the class Student.java as shown below.
Student.java
package com.tutorialspoint.oxm.model; public class Student { String name; int age; int id; public String getName(){ return name; } public void setName(String name){ this.name = name; } public int getAge(){ return age; } public void setAge(int age){ this.age = age; } public int getId(){ return id; } public void setId(int id){ this.id = id; } }
Update applicationcontext.xml in src → main → resources with the following content to use XStreamMarshaller. XStreamMarshaller object can be used for both marshalling and unmarshalling.
applicationcontext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:oxm="http://www.springframework.org/schema/oxm" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/oxm http://www.springframework.org/schema/oxm/spring-oxm-3.0.xsd"> <bean id="xstreamMarshaller" class="org.springframework.oxm.xstream.XStreamMarshaller"> <property name="annotatedClasses" value="com.tutorialspoint.oxm.model.Student"></property> </bean> </beans>
Spring OXM - Test XStream
Update the main class OXMApplication.java with marshaller and unmarshaller objects. The objective of this class is to marshall a student object to student.xml using marshaller object and then unmarshall the student.xml to student object using unmarshaller object.
Example
OXMApplication.java
package com.tutorialspoint.oxm; import java.io.FileInputStream; import java.io.FileWriter; import java.io.IOException; import javax.xml.transform.stream.StreamResult; import javax.xml.transform.stream.StreamSource; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import org.springframework.oxm.Marshaller; import org.springframework.oxm.Unmarshaller; import org.springframework.oxm.XmlMappingException; import com.tutorialspoint.oxm.model.Student; public class OXMApplication { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("applicationcontext.xml"); Marshaller marshaller = (Marshaller)context.getBean("xstreamMarshaller"); Unmarshaller unmarshaller = (Unmarshaller)context.getBean("xstreamMarshaller"); // create student object Student student = new Student(); student.setAge(14); student.setName("Soniya"); try { marshaller.marshal(student, new StreamResult(new FileWriter("student.xml"))); System.out.println("Student marshalled successfully."); FileInputStream is = new FileInputStream("student.xml"); Student student1 = (Student)unmarshaller.unmarshal(new StreamSource(is)); System.out.println("Age: " + student1.getAge() + ", Name: " + student1.getName()); } catch(IOException | XmlMappingException ex) { ex.printStackTrace(); } } }
Output
Right click in the content area of the file in eclipse and then select Run as java application and verify the output.
Oct 11, 2021 9:18:37 AM org.springframework.context.support.ClassPathXmlApplicationContext prepareRefresh INFO: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@29ca901e: startup date [Mon Oct 11 09:18:36 IST 2021]; root of context hierarchy Oct 11, 2021 9:18:37 AM org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions INFO: Loading XML bean definitions from class path resource [applicationcontext.xml] WARNING: An illegal reflective access operation has occurred WARNING: Illegal reflective access by com.thoughtworks.xstream.core.util.Fields (file:/C:/Users/intel/.m2/repository/com/thoughtworks/xstream/xstream/1.4.8/xstream-1.4.8.jar) to field java.util.TreeMap.comparator WARNING: Please consider reporting this to the maintainers of com.thoughtworks.xstream.core.util.Fields WARNING: Use --illegal-access=warn to enable warnings of further illegal reflective access operations WARNING: All illegal access operations will be denied in a future release Student marshalled successfully. Age: 14, Name: Soniya
Spring OXM - Update Project Castor
Update the content of pom.xml to have castor dependencies as shown below −
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.tutorialspoint</groupId> <artifactId>springoxm</artifactId> <version>0.0.1-SNAPSHOT</version> <name>Spring OXM</name> <description>Spring OXM Project</description> <properties> <org.springframework.version>4.3.7.RELEASE</org.springframework.version> <org.hibernate.version>5.2.9.Final</org.hibernate.version> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${org.springframework.version}</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-oxm</artifactId> <version>${org.springframework.version}</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.codehaus.castor</groupId> <artifactId>castor-core</artifactId> <version>1.4.1</version> </dependency> <dependency> <groupId>org.codehaus.castor</groupId> <artifactId>castor-xml</artifactId> <version>1.4.1</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${java.version}</source> <target>${java.version}</target> </configuration> </plugin> </plugins> </build> </project>
Add a mapping xml required for castor mapping to map Student class as mappings.xml under src → main → resources folder as shown below.
mappings.xml
<?xml version="1.0"?> <!DOCTYPE mapping PUBLIC "-//EXOLAB/Castor Mapping DTD Version 1.0//EN" "http://castor.org/mapping.dtd"> <mapping> <class name="com.tutorialspoint.oxm.model.Student" auto-complete="true" > <map-to xml="Student"/> <field name="id" type="integer"> <bind-xml name="id" node="attribute"></bind-xml> </field> <field name="name"> <bind-xml name="name"></bind-xml> </field> <field name="age"> <bind-xml name="age" type="int"></bind-xml> </field> </class> </mapping>
Update applicationcontext.xml in src → main → resources with the following content to use CastorMarshaller. CastorMarshaller object can be used for both marshalling and unmarshalling.
applicationcontext.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:oxm="http://www.springframework.org/schema/oxm" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/oxm http://www.springframework.org/schema/oxm/spring-oxm-3.0.xsd"> <bean id="castorMarshaller" class="org.springframework.oxm.castor.CastorMarshaller"> <property name="targetClass" value="com.tutorialspoint.oxm.model.Student"></property> <property name="mappingLocation" value="mappings.xml"></property> </bean> </beans>
Spring OXM - Test Castor
Update the main class OXMApplication.java with marshaller and unmarshaller objects. The objective of this class is to marshall a student object to student.xml using marshaller object and then unmarshall the student.xml to student object using unmarshaller object.
Example
OXMApplication.java
package com.tutorialspoint.oxm; import java.io.FileInputStream; import java.io.FileWriter; import java.io.IOException; import javax.xml.transform.stream.StreamResult; import javax.xml.transform.stream.StreamSource; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import org.springframework.oxm.Marshaller; import org.springframework.oxm.Unmarshaller; import org.springframework.oxm.XmlMappingException; import com.tutorialspoint.oxm.model.Student; public class OXMApplication { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("applicationcontext.xml"); Marshaller marshaller = (Marshaller)context.getBean("castorMarshaller"); Unmarshaller unmarshaller = (Unmarshaller)context.getBean("castorMarshaller"); // create student object Student student = new Student(); student.setAge(14); student.setName("Soniya"); try { marshaller.marshal(student, new StreamResult(new FileWriter("student.xml"))); System.out.println("Student marshalled successfully."); FileInputStream is = new FileInputStream("student.xml"); Student student1 = (Student)unmarshaller.unmarshal(new StreamSource(is)); System.out.println("Age: " + student1.getAge() + ", Name: " + student1.getName()); } catch(IOException | XmlMappingException ex) { ex.printStackTrace(); } } }
Output
Right click in the content area of the file in eclipse and then select Run as java application and verify the output.
Oct 11, 2021 9:45:34 AM org.springframework.context.support.ClassPathXmlApplicationContext prepareRefresh INFO: Refreshing org.springframework.context.support.ClassPathXmlApplicationContext@6adede5: startup date [Mon Oct 11 09:45:34 IST 2021]; root of context hierarchy Oct 11, 2021 9:45:35 AM org.springframework.beans.factory.xml.XmlBeanDefinitionReader loadBeanDefinitions INFO: Loading XML bean definitions from class path resource [applicationcontext.xml] WARNING: An illegal reflective access operation has occurred WARNING: Illegal reflective access by org.exolab.castor.xml.BaseXercesOutputFormat (file:/C:/Users/intel/.m2/repository/org/codehaus/castor/castor-xml/1.4.1/castor-xml-1.4.1.jar) to method com.sun.org.apache.xml.internal.serialize.OutputFormat.setMethod(java.lang.String) WARNING: Please consider reporting this to the maintainers of org.exolab.castor.xml.BaseXercesOutputFormat WARNING: Use --illegal-access=warn to enable warnings of further illegal reflective access operations. WARNING: All illegal access operations will be denied in a future release Student marshalled successfully. Age: 14, Name: Soniya