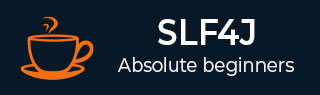
- SLF4J Tutorial
- SLF4J - Home
- SLF4J - Overview
- SLF4J - Logging Frameworks
- SLF4J Vs Log4j
- SLF4J - Environment Setup
- SLF4J - Referenced API
- SLF4J - Hello world
- SLF4J - Error Messages
- SLF4J - Parameterized logging
- SLF4J - Migrator
- SLF4J - Profiling
- SLF4J Useful Resources
- SLF4J - Quick Guide
- SLF4J - Useful Resources
- SLF4J - Discussion
SLF4J - Hello world
In this chapter, we will see a simple basic logger program using SLF4J. Follow the steps described below to write a simple logger.
Step 1 - Create an object of the slf4j.Logger interface
Since the slf4j.Logger is the entry point of the SLF4J API, first, you need to get/create its object
The getLogger() method of the LoggerFactory class accepts a string value representing a name and returns a Logger object with the specified name.
Logger logger = LoggerFactory.getLogger("SampleLogger");
Step 2 - Log the required message
The info() method of the slf4j.Logger interface accepts a string value representing the required message and logs it at the info level.
logger.info("Hi This is my first SLF4J program");
Example
Following is the program that demonstrates how to write a sample logger in Java using SLF4J.
import org.slf4j.Logger; import org.slf4j.LoggerFactory; public class SLF4JExample { public static void main(String[] args) { //Creating the Logger object Logger logger = LoggerFactory.getLogger("SampleLogger"); //Logging the information logger.info("Hi This is my first SLF4J program"); } }
Output
On running the following program initially, you will get the following output instead of the desired message.
SLF4J: Failed to load class "org.slf4j.impl.StaticLoggerBinder". SLF4J: Defaulting to no-operation (NOP) logger implementation SLF4J: See http://www.slf4j.org/codes.html#StaticLoggerBinder for further details.
Since we have not set the classpath to any binding representing a logging framework, as mentioned earlier in this tutorial, SLF4J defaulted to a no-operation implementation. So, to see the message you need to add the desired binding in the project classpath. Since we are using eclipse, set build path for respective JAR file or, add its dependency in the pom.xml file.
For example, if we need to use JUL (Java.util.logging framework), we need to set build path for the jar file slf4j-jdk14-x.x.jar. And if we want to use log4J logging framework, we need to set build path or, add dependencies for the jar files slf4j-log4j12-x.x.jar and log4j.jar.
After adding the binding representing any of the logging frameworks except slf4j-nopx.x.jar to the project (classpath), you will get the following output.
Dec 06, 2018 5:29:44 PM SLF4JExample main INFO: Hi Welcome to Tutorialspoint