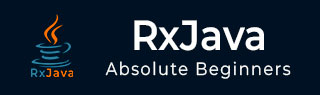
- RxJava Tutorial
- RxJava - Home
- RxJava - Overview
- RxJava - Environment Setup
- Observables
- RxJava - How Observable works
- RxJava - Creating Observables
- RxJava - Single Observable
- RxJava - MayBe Observable
- RxJava - Completable Observable
- RxJava - Using CompositeDisposable
- Operators
- RxJava - Creating Operators
- RxJava - Transforming Operators
- RxJava - Filtering Operators
- RxJava - Combining Operators
- RxJava - Utility Operators
- RxJava - Conditional Operators
- RxJava - Mathematical Operators
- RxJava - Connectable Operators
- Subjects
- RxJava - Subjects
- RxJava - PublishSubject
- RxJava - BehaviorSubject
- RxJava - ReplaySubject
- RxJava - AsyncSubject
- Schedulers
- RxJava - Schedulers
- RxJava - Trampoline Scheduler
- RxJava - NewThread Scheduler
- RxJava - Computation Scheduler
- RxJava - IO Scheduler
- RxJava - From Scheduler
- Miscellaneous
- RxJava - Buffering
- RxJava - Windowing
- RxJava Useful Resources
- RxJava - Quick Guide
- RxJava - Useful Resources
- RxJava - Discussion
RxJava - Creating Operators
Following are the operators which are used to create an Observable.
Sr.No. | Operator & Description |
---|---|
1 | Create Creates an Observable from scratch and allows observer method to call programmatically. |
2 | Defer Do not create an Observable until an observer subscribes. Creates a fresh observable for each observer. |
3 | Empty/Never/Throw Creates an Observable with limited behavior. |
4 | From Converts an object/data structure into an Observable. |
5 | Interval Creates an Observable emitting integers in sequence with a gap of specified time interval. |
6 | Just Converts an object/data structure into an Observable to emit the same or same type of objects. |
7 | Range Creates an Observable emitting integers in sequence of given range. |
8 | Repeat Creates an Observable emitting integers in sequence repeatedly. |
9 | Start Creates an Observable to emit the return value of a function. |
10 | Timer Creates an Observable to emit a single item after given delay. |
Creating Operator Example
Create the following Java program using any editor of your choice in, say, C:\> RxJava.
ObservableTester.java
import io.reactivex.Observable; //Using fromArray operator to create an Observable public class ObservableTester { public static void main(String[] args) { String[] letters = {"a", "b", "c", "d", "e", "f", "g"}; final StringBuilder result = new StringBuilder(); Observable<String> observable = Observable.fromArray(letters); observable .map(String::toUpperCase) .subscribe( letter -> result.append(letter)); System.out.println(result); } }
Verify the Result
Compile the class using javac compiler as follows −
C:\RxJava>javac ObservableTester.java
Now run the ObservableTester as follows −
C:\RxJava>java ObservableTester
It should produce the following output −
ABCDEFG