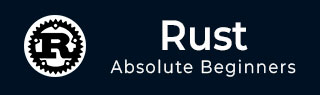
- Rust Tutorial
- Rust - Home
- Rust - Introduction
- Rust - Environment Setup
- Rust - HelloWorld Example
- Rust - Data Types
- Rust - Variables
- Rust - Constant
- Rust - String
- Rust - Operators
- Rust - Decision Making
- Rust - Loop
- Rust - Functions
- Rust - Tuple
- Rust - Array
- Rust - Ownership
- Rust - Borrowing
- Rust - Slices
- Rust - Structure
- Rust - Enums
- Rust - Modules
- Rust - Collections
- Rust - Error Handling
- Rust - Generic Types
- Rust - Input Output
- Rust - File Input/ Output
- Rust - Package Manager
- Rust - Iterator and Closure
- Rust - Smart Pointers
- Rust - Concurrency
- Rust Useful Resources
- Rust - Quick Guide
- Rust - Useful Resources
- Rust - Discussion
Rust - Arithmetic Operators
Assume the values in variables a and b are 10 and 5 respectively.
Sr.No | Operator | Description | Example |
---|---|---|---|
1 | +(Addition) | returns the sum of the operands | a+b is 15 |
2 | -(Subtraction) | returns the difference of the values | a-b is 5 |
3 | * (Multiplication) | returns the product of the values | a*b is 50 |
4 | / (Division) | performs division operation and returns the quotient | a / b is 2 |
5 | % (Modulus) | performs division operation and returns the remainder | a % b is 0 |
NOTE − The ++ and -- operators are not supported in Rust.
Illustration
fn main() { let num1 = 10 ; let num2 = 2; let mut res:i32; res = num1 + num2; println!("Sum: {} ",res); res = num1 - num2; println!("Difference: {} ",res) ; res = num1*num2 ; println!("Product: {} ",res) ; res = num1/num2 ; println!("Quotient: {} ",res); res = num1%num2 ; println!("Remainder: {} ",res); }
Output
Sum: 12 Difference: 8 Product: 20 Quotient: 5 Remainder: 0
rust_operators.htm
Advertisements