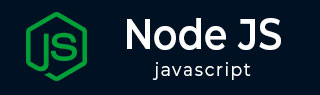
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - querystring.unescape() Method
The querystring module of node.js provides utilities for parsing and formatting URL query strings. The Node.js querystring.unescape() method decodes the URL percent-encoded characters in the provided string and returns a normal string. This method removes the % symbols from the provided string and returns a normal string.
The Node.js querystring.unescape() method is used by the querystring.parse() method and is not used directly. By default, the querystring.unescape() method tries to use the JavaScript built-in decodeURIComponent() method to decode.
Syntax
Following is the syntax of the Node.js querystring.unescape() method −
querystring.unescape(str)
Parameters
This method accepts four parameters. Those are described below.
str − This parameter will hold a URL percent−encoded characters which will be decoded into a normal string.
Return Value
This method will decode the given URL percent−encoded characters and returns a normal string.
Example
If we pass a URL percent−encoded characters to Node.js querystring.unescape() method, it will return a Normal string excluding the URL percent−encoded characters.
In the following example, we are encoding a URL percent−encoded string using Node.js querystring.unescape() method.
const querystring = require('node:querystring'); var result = "Articles%20Node.js%20Tutorialspoin"; console.log(querystring.escape(result)); var unescaped = result; console.log(querystring.unescape(unescaped));
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:querystring'at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a03fcfc3513/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
As we can see in the output below, the Node.js querystring.unescape() method returned a normal string.
Articles Node.js Tutorialspoint
Example
The Node.js decodeURIComponent() method will return a Normal string without the URL percent-encoded characters if we pass it a string of URL percent−encoded characters.
The below example demonstrates the usage of the Node.js decodeURIComponent() method.
const querystring = require('node:querystring'); let string = "Articles%20Nodejs%20Tutorialspoint"; let result = decodeURIComponent(string); console.log(result)
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:querystring' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a03fcfc3513/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30)at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − If we compile and run the above program in local, the Node.js decodeURIComponent() method returns a normal string.
Articles Nodejs Tutorialspoint
Example
If you pass a string including URL percent-encoded characters to both the Node.js querystring.unescape() method and Node.js decodeURIComponent() method, they return the same normal string.
In the following example, we are trying to decode the URL percent-encoded characters by Node.js querystring.unescape() and Node.js decodeURIComponent() methods
const querystring = require('node:querystring'); let string = "Articles%20Nodejs%20Tutorialspoint"; let unescaped = querystring.unescape(string) let decodedURI = decodeURIComponent(string); console.log("The result of querystring() method: " + unescaped); console.log("The result of decodeURIComponent() method: " + decodedURI) if (unescaped === decodedURI){ console.log("Both the results are same."); } else{ console.log("Both the results are not same"); }
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:querystring' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a03fcfc3513/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12)at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
After executing the above program, both the methods returned the same string.
The result of querystring() method: Articles Nodejs Tutorialspoint the result of decodeURIComponent() method: Articles Nodejs Tutorialspoint Both the results are same.
To Continue Learning Please Login