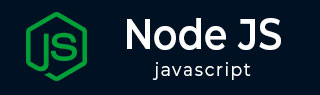
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - querystring.parse() Method
Using the Node.js querystring.parse() method, a URL query string will be parsed as an object with the key and pair values from the given URL query. The returned object of this method doesn't inherit the prototypes from the JavaScript. This means the object methods of JavaScript and others are not defined and will not work.
The query string's percent-encoded characters will by default be assumed to use UTF-8 encoding. The decodeURIComponent option has to be specified to decode alternative character encoding.
Syntax
Following is the syntax of the Node.js querystring.parse() method −
querystring.parse(str[, sep[, eq[, options]]])
Parameters
This method accepts four parameters. Those are described below.
str − This parameter specifies the URL query string to parse.
sep − This parameter describes a substring which is used to define the keys and values pairs in the query string. The default value is '&'.
eq − This parameter describes a substring which is used to define the keys and values in the query string. The default value is '='.
options − This is an object which will allow to modify the behavior of the method. The following properties of this object are described below −
decodeURIComponent − This is a function that is used to decode percent-encoded characters in the query string. By default, it is querystring.unescape().
maxKeys − This property specifies the maximum number of keys to be parsed. The key counting will be infinite if we specify as 0. By default, it is 1000.
Return Value
This method will return a parsed URL query string into a collection of key and value pairs.
Example
If we pass a URL string (str) to querystring.parse() method, it returns an object that has the key and value pairs parsed form the provided URL string.
In the following example, we are passing a URL string to the Node.js querystring.parse() method.
const querystring = require('node:querystring'); let urlQuery = "name=varma1&role=writer&tech=nodejs&permission=true"; console.log(querystring.parse(urlQuery));
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:querystring' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a03fcfc3513/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
After executing the above program, the Node.js querystring.parse() method returns an object with key and value pairs.
[Object: null prototype] { name: 'varma1', role: 'writer', tech: 'nodejs', permission: 'true' }
Example
The Node.js querystring.parse() method will not behave properly if the provided values in sep and eq parameters are not matching with the delimitation of specified URL string. If matches, it will return the object with key and value pairs.
In the program below, we are passing && to the sep parameter and = to eq parameter of the Node.js querystring.parse() method.
const querystring = require('node:querystring'); let urlQuery = "name=varma1&&role=writer&&tech=nodejs&&permission=true"; console.log(querystring.parse(urlQuery, "&&", "="));
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:querystring' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a03fcfc3513/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
If you execute the above program, the output is displayed as follows.
[Object: null prototype] { name: 'varma1', role: 'writer', tech: 'nodejs', permission: 'true' }
Example
If the sep and eq values supplied do not match the delimitation of the specified URL string, the Node.js querystring.parse() method will not behave as intended. If matches, it will return the object with key and value pairs.
In the program below, we are passing & to the sep parameter and − to eq parameter of the Node.js querystring.parse() method.
const querystring = require('node:querystring'); let urlQuery = "User-john&role−softwareeng&tech−HTML&permission−false"; console.log(querystring.parse(urlQuery, "&", "−"));
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:querystring' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a03fcfc3513/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
If you execute the above program, the output is displayed as follows.
[Object: null prototype] { User: 'john', role: 'softwareeng', tech: 'HTML', permission: 'false' }
Example
The Node.js querystring.parse() method will return the key and value pairs up to the maximum keys specified in the maxkeys parameter.
In the below example, we are executing two scenarios. In the first scenario, we are passing 1 to the maxkeys parameter. In second scenario, the maxkeys is set to 2.
const { url } = require('node:inspector'); const querystring = require('node:querystring'); let QueryString = "User−john&role−softwareeng&tech−HTML&permission−false"; console.log(querystring.parse(QueryString, "&", "−", { maxKeys: 1})); console.log(querystring.parse(QueryString, "&", "−", { maxKeys: 2}));
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:inspector' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a03fcfc3513/main.js:1:79) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
If you execute the above program, the output is displayed as follows.
[Object: null prototype] { User: 'john' } [Object: null prototype] { User: 'john', role: 'softwareeng' }
Example
If we provide 0 to the maxkeys parameter, the key counting limitations will be limitless.
In the program below, we are passing 0 to maxkeys parameter of the Node.js querystring.parse() method.
const { url } = require('node:inspector'); const querystring = require('node:querystring'); let QueryString = "User−Kasyap&role−Manager&Exp−eightyrs&permission−true"; console.log(querystring.parse(QueryString, "&", "−", { maxKeys: 0}));
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:inspector' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a03fcfc3513/main.js:1:79) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
If you execute the above program, the output is displayed as follows.
[Object: null prototype] { User: 'Kasyap', role: 'Manager', Exp: 'eightyrs', permission: 'true' }
To Continue Learning Please Login