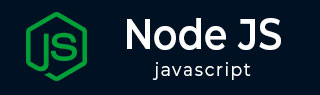
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - querystring.escape() Method
The Node.js querystring module provides utilities for parsing and formatting URL query strings. The querystring.escape() method will return a percent−encoded query string from a normal string. This method is almost similar to the encodeURIComponent function.
This method encodes a normal string to a URL query string by the % symbol. It will iterate through the given string and add the % symbol wherever it is needed. This method takes one parameter, which is the string to be encoded, and returns an encoded version of the same string as its output.
The encoding process replaces certain characters with their hexadecimal equivalents so that they are not interpreted by browsers or databases as part of the code. For example, "&" would become "%26" in an encoded form.
Syntax
Following is the syntax of the Node.js querystring.escape() method −
querystring.escape(str)
Parameters
str − This parameter holds a string that represents an encoded query string.
Return Value
This method returns a string that contains a query string that is produced by a given string.
Example
If we pass a normal string to the Node.js querystring.escape() method, it returns a percent-encoded query string.
In the given program, we are passing a normal string to the Node.js querystring.escape() method.
const querystring = require('node:querystring'); var result = "Articles Node.js Tutorialspoint"; console.log(querystring.escape(result));
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:querystring' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25)at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a038fade650/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
As we can see in the output below, the Node.js querystring.escape() method returned a percent-encoded query string.
Articles%20Node.js%20Tutorialspoint
Example
If we pass a normal string to the encodeURIComponent function, it returns a percent-encoded query string.
In the following example, we are passing a normal string to the encodeURIComponent function.
const querystring = require('node:querystring'); var encodeURIComponent_func = "Articles HTML Tutorialspoint"; console.log(encodeURIComponent(encodeURIComponent_func))
Output
internal/modules/cjs/loader.js:596 throw err; ^Error: Cannot find module 'node:querystring' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a038fade650/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
As we can see in the output, the encodeURIComponent function will return a percent-encoded query string.
Articles%20HTML%20Tutorialspoint
Example
If you pass a normal string to both the Node.js querystring.escape() method and encodeURIComponent() method, return a percent-encoded query string.
In the following example, we are trying to encode the provided string by Node.js querystring.escape() and encodeURIComponent() methods.
const querystring = require('node:querystring'); let string = "Articles Nodejs Tutorialspoint"; let escaped = querystring.escape(string) let encodedURI = encodeURIComponent(string); console.log("The result of querystring() method: " + escaped); console.log("The result of decodeURIComponent() method: " + encodedURI) if (escaped === encodedURI){ console.log("Both the results are same."); } else{ console.log("Both the results are not same"); }
Output
internal/modules/cjs/loader.js:596 throw err; ^ Error: Cannot find module 'node:querystring' at Function.Module._resolveFilename (internal/modules/cjs/loader.js:594:15) at Function.Module._load (internal/modules/cjs/loader.js:520:25) at Module.require (internal/modules/cjs/loader.js:650:17) at require (internal/modules/cjs/helpers.js:20:18) at Object.<anonymous> (/home/cg/root/63a038fade650/main.js:1:83) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Note − To see actual result, execute the above code in local.
After executing the above program, both methods returned the same string.
The result of querystring() method: Articles%20Nodejs%20Tutorialspoint The result of decodeURIComponent() method: Articles%20Nodejs%20Tutorialspoint Both the results are same.
To Continue Learning Please Login