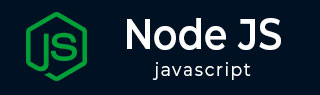
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - assert.strictEqual() Function
The assert module provides a set of assertion functions for verifying invariants. The Node.js assert.strictEqual() function is an inbuilt function of the assert module of Node.js.
The Node.js strictEqual() function is used to determine if two values are equal using a stricter comparison than the == operator. This function compares both the data type and value of two variables or objects and returns true only if they are exactly the same. Also, comparing NaN (Not-A-Number) to any other value, including itself, returns false.
This function will test equality on both of its parameters actual and expected. Whenever both the parameters are similar, the function doesn't throw AssertionError. If both the parameters are not similar, then it will throw an AssertionError to the output. This function is an alias of Node.js assert.deepStrictEqaul() function.
Syntax
Following is the syntax of Node.js assert.strictEqaul() function −
assert.strictEqual(actual, expected[, message]);
Parameters
This function accepts three parameters. The same are described below.
actual − (required) The value passed in this parameter will be evaluated. The value can be of any type.
expected − (required) The value passed in this parameter will be compared to the actual value. The value can be of any type.
message − (optional) String or Error type can be passed as an input into this parameter.
Return Value
This function will return the AssertionError on the terminal if both actual and expected are not matched.
Example
In the following example, we are passing two identical integers to the actual and expected parameters of the Node.js assert.strictEqual() function.
const assert = require('assert'); var num1 = 45; var num2 = 45; assert.strictEqual(num1, num2, 'Both the values are same');
Output
If we compile and run the code, No AssertionError will be thrown to the output. This is true because 45 == 45.
// Returns nothing
Example
In the following example, we are passing two distinct integers to the actual and expected parameters of the function.
const assert = require('assert'); var int1 = 34; var int2 = 10; assert.strictEqual(int1, int2, 'Both the values are not same');
Output
If we compile and run the code, it will throw AssertionError to the output along with the text in message parameter. This is false because 34 !== 10.
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Both the values are not same at Object.<anonymous> (/home/cg/root/639c3f570123e/main.js:5:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Example
In the following example, we are passing two identical strings to the actual and expected parameters of the function.
const assert = require('assert'); var text1 = 'Welcome to India'; var text2 = 'Welcome to India'; assert.strictEqual(text1, text2, 'Both the strings are identical');
Output
If we compile and run the code, No AssertionError will be thrown to the output. This is true because text1 == text2.
// Returns nothing
Example
In the following example, we are passing two string values and checking their type.
const assert = require('assert'); var text1 = 'Rise'; var text2 = 'Rule'; assert.strictEqual(typeof text1 === 'string', typeof text2 === 'number', 'Both the strings are not identical');
Output
If we compile and run the code, it will throw an AssertionError along with the text in the message to the output. This is false because 'text2' == 'number'.
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Both the strings are not identical at Object.<anonymous> (/home/cg/root/639c3f570123e/main.js:6:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Example
In the following example, we are passing two different string values and not passing any text to the message parameter.
const assert = require('assert'); assert.strictEqual('RRR', 'ZZZ');
Output
If we compile and run the code, it will throw an AssertionError to the output and a default message will be assigned by the function.
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Input A expected to strictly equal input B: + expected - actual - 'RRR' + 'ZZZ' at Object.<anonymous> (/home/cg/root/639c3f570123e/main.js:3:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Example
In the following example below, we are not passing any input values to the actual, expected, and message parameters of the function.
const assert = require('assert'); assert.strictEqual();
Output
// Returns Nothing
Note − Sometimes online compiler may not give us the expected result, so we are executing the above code locally.
If we compile and run the code, the function will throw an AssertionError along with the default message "The 'actual' and 'expected' arguments must be specified" to the output.
node:assert:568 throw new ERR_MISSING_ARGS('actual', 'expected'); ^ TypeError [ERR_MISSING_ARGS]: The "actual" and "expected" arguments must be specified at new NodeError (node:internal/errors:387:5) at Function.strictEqual (node:assert:568:11) at Object.<anonymous> (C:\Users\Lenovo\Desktop\JavaScript\nodefile.js:3:8) at Module._compile (node:internal/modules/cjs/loader:1126:14) at Object.Module._extensions..js (node:internal/modules/cjs/loader:1180:10) at Module.load (node:internal/modules/cjs/loader:1004:32) at Function.Module._load (node:internal/modules/cjs/loader:839:12) at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12) at node:internal/main/run_main_module:17:47 { code: 'ERR_MISSING_ARGS' }
To Continue Learning Please Login