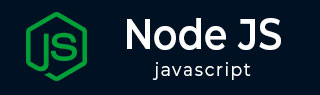
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - new assert.CallTracker()
In this chapter, we will discuss what is the assert.CallTracker() class in Node.js. Before jumping into the above-mentioned class let's get to know what is Assertion in Node.js.
Assertion in Node.js
Programming expressions can be evaluated using Assertions. Node.js provides a module called assertion and this module contains different types of assertion functions. The assert module is used to perform unit testing in Node.js.
Assertions are crucial components in programming language because they allow us to evaluate if a piece of code is correct or accurate. By this, we can build a good quality code.
Note − To understand and to get to know how assertion functions work, you need to install the latest Node version on your machine.
How to import and use the Assertion module
To import the assert module, we need to include the piece of code which is in the below snippet.
const assert = require('assert');
What is assert.CallTracker() ?
The Node.js assert.CallTracker() is used to track if the function(s) were called a specific number of times and that will be done by using some of its special functions.
To create a CallTracker object, we need to add the code which is in the below snippet.
const assert = require('assert'); const tracker = new assert.CallTracker();
Now let's add a few more lines of code in the examples below to see the working of CallTracker functions.
In the following example,
We are creating a function func that will print a multiplication table of a number up to a particular number.
Then we are creating a function funccall that takes the function func as the first parameter of the Node.js tracker.calls() function.
We are not passing integer value in the second parameter, so by default tracker.calls() assumes the value is 1 and expects the function to call one time.
The only way to track the number of times function func was called is to wrap it tracker.calls(), this will hold the record of the number of times the func is called.
Then we are calling the tracker.verify() to verify if the funccall has been called one time as expected.
Example
const assert = require('assert'); const tracker = new assert.CallTracker(); function func() { const number = 5; //creating a multiplication table for(let i = 1; i <= 5; i++) { // multiply i with number const result = i * number; // display the result console.log(`${number} * ${i} = ${result}`); } }; const funccall = tracker.calls(func); funccall(); tracker.verify();
Output
const assert = require('assert'); const tracker = new assert.CallTracker(); function func() { const number = 5; //creating a multiplication table for(let i = 1; i <= 5; i++) { // multiply i with number const result = i * number; // display the result console.log(`${number} * ${i} = ${result}`); } }; const funccall = tracker.calls(func); funccall(); tracker.verify();
Note − Sometimes online compiler may not give us the expected result, so we are executing the above code locally.
If we compile and run the code, no error will be thrown as funcall was called as per expected the number of times.
5 * 1 = 5 5 * 2 = 10 5 * 3 = 15 5 * 4 = 20 5 * 5 = 25
What is a tracker report?
The tracker report is an array of objects containing detailed information about the error that occurred when tracking a function(s).
The information object holding is described below −
message<string> The message will be assigned by the function automatically.
actual<number> This shows the actual number of times the function was called.
expected<number> This shows the number of times the function was expected to call.
operator<operator> This will let us know the name of the function that is wrapped by the wrapper function.
stack<Object> A stack trace of the function.
Now let's look into the example where we used tracker.report() to get detailed information about the error that occurred when tracking a function.
Example
In the following example below,
In this code we expected funccall to be called for 3 times. But the funcall was called only once so that an error will be thrown.
As we called tracker.report(), it will show the details of how many times the function is called and expected to call, etc.
const assert = require('assert'); const tracker = new assert.CallTracker(); function func() { const number = 5; //creating a multiplication table for(let i = 1; i <= 2; i++) { // multiply i with number const result = i * number; // display the result console.log(`${number} * ${i} = ${result}`); } }; const funccall = tracker.calls(func, 3); funccall(); console.log(tracker.report());
Output
/home/cg/root/63a002c52763b/main.js:3 const tracker = new assert.CallTracker(); ^ TypeError: assert.CallTracker is not a constructor at Object.<anonymous> (/home/cg/root/63a002c52763b/main.js:3:17) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Note − Sometimes online compiler may not give us the expected result, so we are executing the above code locally.
On executing the above program, it will generate the following output −
5 * 1 = 5 5 * 2 = 10 [ { message: 'Expected the func function to be executed 3 time(s) but was executed 1 time(s).', actual: 1, expected: 3, operator: 'func', stack: Error at CallTracker.calls (node:internal/assert/calltracker:44:19) at Object.<anonymous> (C:\Users\Lenovo\Desktop\JavaScript\nodefile.js:19:26) at Module._compile (node:internal/modules/cjs/loader:1126:14) at Object.Module._extensions..js (node:internal/modules/cjs/loader:1180:10) at Module.load (node:internal/modules/cjs/loader:1004:32) at Function.Module._load (node:internal/modules/cjs/loader:839:12) at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12) at node:internal/main/run_main_module:17:47 } ]
To Continue Learning Please Login