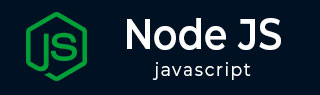
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - assert.fail() Function
The Node.js assert.fail() function will throw an AssertionError along with the error message we passed as a parameter or with the default error message. If the message, we passed is an instance of an Error then it will be thrown instead of the AssertionError.
The assert module provides a set of assertion functions for verifying invariants. The Node.js assert.fail() function is an inbuilt function of the assert module of Node.js.
Syntax
Following is the syntax of Node.js assert.fail() function −
assert.fail([message]);
Parameters
message − (optional) String or Error type can be passed as an input into this parameter. By default, it will print 'Failed'.
Return Value
This method will return an AssertionError along with the message passed in the parameter to the output.
Example
In the example below, we are passing a text to the message parameter of the Node.js assert.fail() function.
const assert = require('assert'); const err = 'Warning'; assert.fail(err);
Output
When we compile and run the code, the function will throw AssertionError along with the message to the output.
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Warning at Object.<anonymous> (/home/cg/root/639c30a0a5fb2/main.js:4:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Example
In the example below, we are passing an instance of an Error to the message parameter of the Node.js assert.fail() function.
const assert = require('assert'); const err = new TypeError('Alert, this is a warning...') assert.fail(err);
Output
When we compile and run the code, the function will not throw AssertionError instead; the Error will be thrown.
assert.js:77 if (obj.message instanceof Error) throw obj.message; ^ TypeError: Alert, this is a warning... at Object.<anonymous> (/home/cg/root/639c30a0a5fb2/main.js:3:13) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Example
In the example below, we are not passing any text to the message parameter to the function. So it will assign the default message as 'Failed'.
const assert = require('assert'); assert.fail();
Output
On executing the above program, it will generate the following output −
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Failed at Object.<anonymous> (/home/cg/root/639c30a0a5fb2/main.js:3:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
To Continue Learning Please Login