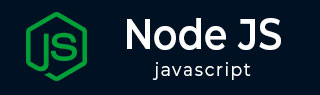
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - assert.doesNotThrow() Function
The Node.js assert.doesNotThrow() function asserts that the function passed as a parameter to fn does not throw an error.
Using the assert.doesNotThrow() function is not useful because there is no proper benefit of catching an error and throwing it again. Instead, it is quite expressive when comments are added to the specific code that should not throw.
Syntax
Following is the syntax of Node.js assert.doesNotThrow() function −
assert.doesNotThrow(fn[, error][, message]);
Parameters
This function accepts three parameters. The same are described below.
fn − (Required) This parameter holds a function fn and expects the function to not throw an error.
error − (Optional) This parameter can be a RegExp, validation function.
message − (Optional) String or Error type can be passed as an input into this parameter.
Return Value
This function asserts that the function fn does not throw an error. If an error is thrown, there will be two scenarios −
If the error type is the same as the error parameter, then the assert.doesNotThrow() function throws an AssertionError to the output.
If the error parameter is not passed or the error type does not match, then propagates back to the caller.
Example
In the following example, we are creating a function and inside the function, we are invoking a TypeError.
const assert = require('assert').strict; function func(){ throw new TypeError('Error...'); }; assert.doesNotThrow( () => { func(); }, TypeError );
Output
On executing the above program, it will result in an Assertion error with the message 'Got unwanted exception'.
assert.js:79 throw new AssertionError(obj); ^ AssertionError [ERR_ASSERTION]: Got unwanted exception. Actual message: "Error..." at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:7:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
Example
In the following example, we are creating a function and inside the function, we are invoking a TypeError. But the error parameter is holding SyntaxError instead of TypeError which is of a different type.
const assert = require('assert'); function func(){ throw new TypeError('Error...'); } assert.doesNotThrow( () => { func() }, SyntaxError);
Output
On executing the above program, the function will throw a TypeError instead of AssertionError because there is no matching error type in the assertion.
assert.js:604 throw actual; ^ TypeError: Error... at func (/home/cg/root/639c2bf348ea8/main.js:4:9) at assert.doesNotThrow (/home/cg/root/639c2bf348ea8/main.js:7:30) at getActual (assert.js:497:5) at Function.doesNotThrow (assert.js:616:32) at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:7:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32)at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3)
Example
In the following example below, we are creating a function and inside the function, we are invoking a TypeError with an error message. We are also passing a text into the message parameter.
const assert = require('assert'); function func(){ throw new TypeError('Error...'); } assert.doesNotThrow( () => { func() }, /Error.../, 'This is a text from MESSAGE parameter.');
Output
Since the parameter is also the same as the error message in TypeError, the function will throw an AssertionError along with the message to the output.
assert.js:79 throw new AssertionError(obj); ^AssertionError [ERR_ASSERTION]: Got unwanted exception: This is a text from MESSAGE parameter. Actual message: "Error..." at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:7:8) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) at Function.Module.runMain (internal/modules/cjs/loader.js:744:10) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3)
To Continue Learning Please Login