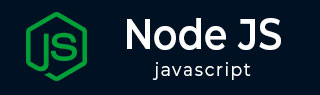
- Node.js Tutorial
- Node.js - Home
- Node.js - Introduction
- Node.js - Environment Setup
- Node.js - First Application
- Node.js - REPL Terminal
- Node.js - Command Line Options
- Node.js - Package Manager (NPM)
- Node.js - Callbacks Concept
- Node.js - Upload Files
- Node.js - Send an Email
- Node.js - Events
- Node.js - Event Loop
- Node.js - Event Emitter
- Node.js - Debugger
- Node.js - Global Objects
- Node.js - Console
- Node.js - Process
- Node.js - Scaling Application
- Node.js - Packaging
- Node.js - Express Framework
- Node.js - RESTFul API
- Node.js - Buffers
- Node.js - Streams
- Node.js - File System
- Node.js MySQL
- Node.js - MySQL Get Started
- Node.js - MySQL Create Database
- Node.js - MySQL Create Table
- Node.js - MySQL Insert Into
- Node.js - MySQL Select From
- Node.js - MySQL Where
- Node.js - MySQL Order By
- Node.js - MySQL Delete
- Node.js - MySQL Update
- Node.js - MySQL Join
- Node.js MongoDB
- Node.js - MongoDB Get Started
- Node.js - MongoDB Create Database
- Node.js - MongoDB Create Collection
- Node.js - MongoDB Insert
- Node.js - MongoDB Find
- Node.js - MongoDB Query
- Node.js - MongoDB Sort
- Node.js - MongoDB Delete
- Node.js - MongoDB Update
- Node.js - MongoDB Limit
- Node.js - MongoDB Join
- Node.js Modules
- Node.js - Modules
- Node.js - Built-in Modules
- Node.js - Utility Modules
- Node.js - Web Module
- Node.js Useful Resources
- Node.js - Quick Guide
- Node.js - Useful Resources
- Node.js - Dicussion
Node.js - assert.doesNotReject() Function
The Node.js assert.doesNotReject() function is an inbuilt function of the assert module of Node.js. It is used to check if the given promise is not rejected.
In the assert.doesNotReject() function if the provided parameter is a promise, it awaits the promise. If the provided parameter is a function, then it is called immediately and awaits the return promise to complete. This function will check if the provided promise is rejected or not. This function behaves identically to assert.doesNotThrow().
The assert.doesNotReject() is not useful to use because there is only a little benefit in catching an error and rejecting the error again. Instead, it would be better if we add appropriate comments to a particular code path that should not be rejected and keeping error message as expressive as possible.
Syntax
Following is the syntax of Node.js assert.doesNotReject() function −
assert.doesNotReject(asyncFn[, error][, message]);
Parameters
This function accepts three parameters. The same are described below.
asyncFn − (Required) This parameter holds an async function that will throw errors synchronously.
error − (Optional) This parameter can hold Class, Regular Expression, Validation function, or an object where each property will be tested.
message − (Optional) This parameter can hold Class, Regular Expression, Validation function, or an object where each property will be tested.
Return Value
The function assert.doesNotReject() will return the rejected promise to the output.
Example
Following is the usage of the Node.js assert.doesNotReject() function.
const assert = require('assert'); (async () => { await assert.doesNotReject( async () => { throw new TypeError('Wrong value'); }, TypeError ); } )();
Output
Following is the output of the above code −
(node:40094) UnhandledPromiseRejectionWarning: AssertionError [ERR_ASSERTION]: Got unwanted rejection. Actual message: "Wrong value" at process._tickCallback (internal/process/next_tick.js:68:7) at Function.Module.runMain (internal/modules/cjs/loader.js:746:11) at startup (internal/bootstrap/node.js:238:19) at bootstrapNodeJSCore (internal/bootstrap/node.js:572:3) (node:40094) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 2) (node:40094) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled will terminate the Node.js process with a non-zero exit code.
Example
Following is the usage of the Node.js assert.doesNotReject() function in another scenario.
const assert = require('assert'); (async () => { await assert.doesNotReject( async () => { throw new TypeError('Error occured!!!'); }, SyntaxError ); } )();
Output
Following is the output of the above code −
(node:43403) UnhandledPromiseRejectionWarning: TypeError: Error occured!!! at assert.doesNotReject (/home/cg/root/639c2bf348ea8/main.js:6:23) at waitForActual (assert.js:518:21) at Function.doesNotReject (assert.js:620:39)at /home/cg/root/639c2bf348ea8/main.js:4:22 at Object.<anonymous> (/home/cg/root/639c2bf348ea8/main.js:11:2) at Module._compile (internal/modules/cjs/loader.js:702:30) at Object.Module._extensions..js (internal/modules/cjs/loader.js:713:10) at Module.load (internal/modules/cjs/loader.js:612:32) at tryModuleLoad (internal/modules/cjs/loader.js:551:12) at Function.Module._load (internal/modules/cjs/loader.js:543:3) (node:43403) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 2) (node:43403) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled will terminate the Node.js process with a non-zero exit code.
To Continue Learning Please Login