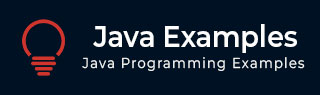
- Java Programming Examples
- Example - Home
- Example - Environment
- Example - Strings
- Example - Arrays
- Example - Date & Time
- Example - Methods
- Example - Files
- Example - Directories
- Example - Exceptions
- Example - Data Structure
- Example - Collections
- Example - Networking
- Example - Threading
- Example - Applets
- Example - Simple GUI
- Example - JDBC
- Example - Regular Exp
- Example - Apache PDF Box
- Example - Apache POI PPT
- Example - Apache POI Excel
- Example - Apache POI Word
- Example - OpenCV
- Example - Apache Tika
- Example - iText
- Java Tutorial
- Java - Tutorial
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
How to display a message in a new frame using Java
Problem Description
How to display a message in a new frame?
Solution
Following example demonstrates how to display message in a new frame by creating a frame using JFrame() & using JFrames getContentPanel(), setSize() & setVisible() methods to display this frame.
import java.awt.*; import java.awt.font.FontRenderContext; import java.awt.geom.Rectangle2D; import javax.swing.JFrame; import javax.swing.JPanel; public class Main extends JPanel { public void paint(Graphics g) { Graphics2D g2 = (Graphics2D) g; g2.setRenderingHint(RenderingHints.KEY_ANTIALIASING, RenderingHints.VALUE_ANTIALIAS_ON); g2.setFont(new Font("Serif", Font.PLAIN, 48)); paintHorizontallyCenteredText(g2, "Java Source", 200, 75); paintHorizontallyCenteredText(g2, "and", 200, 125); paintHorizontallyCenteredText(g2, "Support", 200, 175); } protected void paintHorizontallyCenteredText( Graphics2D g2, String s, float centerX, float baselineY) { FontRenderContext frc = g2.getFontRenderContext(); Rectangle2D bounds = g2.getFont().getStringBounds(s, frc); float width = (float) bounds.getWidth(); g2.drawString(s, centerX - width / 2, baselineY); } public static void main(String[] args) { JFrame f = new JFrame(); f.getContentPane().add(new Main()); f.setSize(450, 350); f.setVisible(true); } }
Result
The above code sample will produce the following result.
JAVA and J2EE displayed in a new Frame.
The following is an example to display a message in a new frame.
import java.awt.BorderLayout; import java.awt.Button; import java.awt.Component; import java.awt.Frame; import java.awt.TextArea; public class Main { public static void main(String[] args) { Frame f = new Frame("Tutorialspoint"); Component text = new TextArea("Sairamkrishna Mamamahe"); Component button = new Button("Button"); f.add(text, BorderLayout.NORTH); f.add(button, BorderLayout.SOUTH); int width = 300; int height = 300; f.setSize(width, height); f.setVisible(true); } }
java_simple_gui.htm
Advertisements