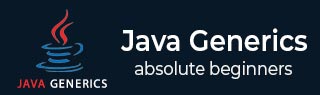
- Java Generics Tutorial
- Java Generics - Home
- Java Generics - Overview
- Java Generics - Environment Setup
- Examples - Generic Classes
- Java Generics - Generic Classes
- Type Parameter Naming Conventions
- Java Generics - Type inference
- Java Generics - Generic Methods
- Java Generics - Multiple Type
- Java Generics - Parameterized Types
- Java Generics - Raw Types
- Examples - Bounded Type
- Bounded Type Parameters
- Java Generics - Multiple Bounds
- Examples - Collections
- Java Generics - Generic List
- Java Generics - Generic Set
- Java Generics - Generic Map
- Examples - Wild Cards
- Upper Bounded Wildcards
- Generics - Unbounded Wildcards
- Lower Bounded Wildcards
- Generics - Guidelines for Wildcards
- Type Erasure
- Java Generics - Types Erasure
- Java Generics - Bound Types Erasure
- Unbounded Types Erasure
- Java Generics - Methods Erasure
- Restrictions on Generics
- Java Generics - No Primitive Types
- Java Generics - No Instance
- Java Generics - No Static field
- Java Generics - No Cast
- Java Generics - No instanceOf
- Java Generics - No Array
- Java Generics - No Exception
- Java Generics - No Overload
- Java Generics Useful Resources
- Java Generics - Quick Guide
- Java Generics - Useful Resources
- Java Generics - Discussion
Java Generics - Multiple Type Parameters
A Generic class can have muliple type parameters. Following example will showcase above mentioned concept.
Example
Create the following java program using any editor of your choice.
GenericsTester.java
package com.tutorialspoint; public class GenericsTester { public static void main(String[] args) { Box<Integer, String> box = new Box<Integer, String>(); box.add(Integer.valueOf(10),"Hello World"); System.out.printf("Integer Value :%d\n", box.getFirst()); System.out.printf("String Value :%s\n", box.getSecond()); Box<String, String> box1 = new Box<String, String>(); box1.add("Message","Hello World"); System.out.printf("String Value :%s\n", box1.getFirst()); System.out.printf("String Value :%s\n", box1.getSecond()); } } class Box<T, S> { private T t; private S s; public void add(T t, S s) { this.t = t; this.s = s; } public T getFirst() { return t; } public S getSecond() { return s; } }
This will produce the following result.
Output
Integer Value :10 String Value :Hello World String Value :Message String Value :Hello World
Advertisements