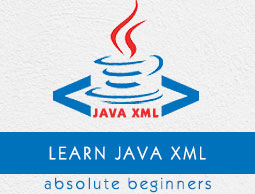
- Javax.xml.bind classes
- Home
- Binder
- DatatypeConverter
- JAXB
- JAXBContext
- JAXBElement
- JAXBElement.GlobalScope
- JAXBIntrospector
- Marshaller.Listener
- SchemaOutputResolver
- Unmarshaller.Listener
- Javax.xml.bind.util classes
- JAXBResult
- JAXBSource
- ValidationEventCollector
- Javax.xml.parsers classes
- DocumentBuilder
- DocumentBuilderFactory
- SAXParser
- SAXParserFactory
- Javax.xml.soap classes
- AttachmentPart
- MessageFactory
- MimeHeader
- MimeHeaders
- SAAJMetaFactory
- SOAPConnection
- SOAPConnectionFactory
- SOAPFactory
- SOAPMessage
- SOAPPart
- Javax.xml.validation classes
- Schema
- SchemaFactory
- TypeInfoProvider
- Validator
- ValidatorHandler
- Javax.xml.xpath classes
- XPathConstants
- XPathFactory
- Java Useful Resources
- Java - Quick Guide
- Java - Useful Resources
AttachmentPart setBase64Content() Method
Description
The Javax.xml.soap.AttachmentPart.setBase64Content(InputStream content, String contentType) method sets the content of this attachment part from the Base64 source InputStream and sets the value of the Content-Type header to the value contained in contentType, This method would first decode the base64 input and write the resulting raw bytes to the attachment. A subsequent call to getSize() may not be an exact measure of the content size.
Declaration
Following is the declaration for javax.xml.soap.AttachmentPart.setBase64Content() method
public abstract void setBase64Content(InputStream content, String contentType)
Parameters
content − the base64 encoded data to add to the attachment part
contentType − the value to set into the Content-Type header
Return Value
This method does not return a value.
Exception
SOAPException − if an there is an error in setting the content
NullPointerException − if content is null
Example
The following example shows the usage of javax.xml.soap.AttachmentPart.setBase64Content() method.
package com.tutorialspoint; import com.sun.xml.internal.messaging.saaj.util.Base64; import com.sun.xml.internal.messaging.saaj.util.ByteInputStream; import java.io.InputStream; import javax.xml.soap.*; public class AttachmentPartDemo { public static void main(String[] args) { try { // create a new SOAPMessage SOAPMessage message = MessageFactory.newInstance().createMessage(); // create a string as a new attachment String attachment = "This is an attachment"; // create the attachment part AttachmentPart attachPart = message.createAttachmentPart(); // encode attachment and add it as AttachmentPart byte[] encoded = Base64.encode(attachment.getBytes()); ByteInputStream bis = new ByteInputStream(encoded, 0, encoded.length); attachPart.setBase64Content(bis, "plain/text"); // add the attachment part in the message message.addAttachmentPart(attachPart); // get the inputstream for attachPart InputStream is = attachPart.getBase64Content(); // print the content for (int i = 0; i < is.available(); i++) { System.out.print("" + (char) is.read()); } } catch (Exception ex) { ex.printStackTrace(); } } }
If we compile the code and execute it, this will produce the following result −
VGhpcyBpcyBhbi
To Continue Learning Please Login